import java.io.*; public class ThrowMultiExceptionsDemo { public static void main(String[] args) { try { throwsTest(); } catch(IOException e) { System.out.println("捕捉异常"); } } private static void throwsTest() throws ArithmeticException,IOException { System.out.println("这只是一个测试"); // 程序处理过程假设发生异常 throw new IOException(); //throw new ArithmeticException(); } }
public class CatchWho { public static void main(String[] args) { try { try { throw new ArrayIndexOutOfBoundsException(); } catch(ArrayIndexOutOfBoundsException e) { System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch"); } throw new ArithmeticException(); } catch(ArithmeticException e) { System.out.println("发生ArithmeticException"); } catch(ArrayIndexOutOfBoundsException e) { System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch"); } } }
CatchWho2.java public class CatchWho2 { public static void main(String[] args) { try { try { throw new ArrayIndexOutOfBoundsException(); } catch(ArithmeticException e) { System.out.println( "ArrayIndexOutOfBoundsException" + "/内层try-catch"); } throw new ArithmeticException(); } catch(ArithmeticException e) { System.out.println("发生ArithmeticException"); } catch(ArrayIndexOutOfBoundsException e) { System.out.println( "ArrayIndexOutOfBoundsException" + "/外层try-catch"); } } }
CheckedExceptionDemo.java import java.io.*; public class CheckedExceptionDemo { public static void main(String[] args) { try { BufferedReader buf = new BufferedReader( new InputStreamReader(System.in)); //抛出受控的异常 System.out.print("请输入整数: "); int input = Integer.parseInt(buf.readLine()); //有可能引发运行时异常 System.out.println("input x 10 = " + (input*10)); } //以下异常处理语句块是必须的,否则无法通过编译 catch(IOException e) { System.out.println("I/O错误"); } //以下异常处理语句块可以省略,不影响编译,但在运行时出错 catch(NumberFormatException e) { System.out.println("输入必须为整数"); } } }
EmbededFinally.java public class EmbededFinally { public static void main(String args[]) { int result; try { System.out.println("in Level 1"); try { System.out.println("in Level 2"); // result=100/0; //Level 2 try { System.out.println("in Level 3"); result=100/0; //Level 3 } catch (Exception e) { System.out.println("Level 3:" + e.getClass().toString()); } finally { System.out.println("In Level 3 finally"); } // result=100/0; //Level 2 } catch (Exception e) { System.out.println("Level 2:" + e.getClass().toString()); } finally { System.out.println("In Level 2 finally"); } // result = 100 / 0; //level 1 } catch (Exception e) { System.out.println("Level 1:" + e.getClass().toString()); } finally { . System.out.println("In Level 1 finally"); } } }
ExceptionLinkInRealWorld.java /** * 自定义的异常类 * @author JinXuLiang * */ class MyException extends Exception { public MyException(String Message) { super(Message); } public MyException(String message, Throwable cause) { super(message, cause); } public MyException( Throwable cause) { super(cause); } } public class ExceptionLinkInRealWorld { public static void main( String args[] ) { try { throwExceptionMethod(); //有可能抛出异常的方法调用 } catch ( MyException e ) { System.err.println( e.getMessage() ); System.err.println(e.getCause().getMessage()); } catch ( Exception e ) { System.err.println( "Exception handled in main" ); } doesNotThrowException(); //不抛出异常的方法调用 } public static void throwExceptionMethod() throws MyException { try { System.out.println( "Method throwException" ); throw new Exception("系统运行时引发的特定的异常"); // 产生了一个特定的异常 } catch( Exception e ) { System.err.println( "Exception handled in method throwException" ); //转换为一个自定义异常,再抛出 throw new MyException("在方法执行时出现异常",e); } finally { System.err.println( "Finally executed in throwException" ); } // any code here would not be reached } public static void doesNotThrowException() { try { System.out.println( "Method doesNotThrowException" ); } catch( Exception e ) { System.err.println( e.toString() ); } finally { System.err.println( "Finally executed in doesNotThrowException" ); } System.out.println( "End of method doesNotThrowException" ); } }
ExplorationJDKSource.java public class ExplorationJDKSource { /** * @param args */ public static void main(String[] args) { System.out.println(new A()); } } class A{}
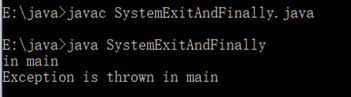
OverrideThrows.java import java.io.*; public class OverrideThrows { public void test()throws IOException { FileInputStream fis = new FileInputStream("a.txt"); } } class Sub extends OverrideThrows { //如果test方法声明抛出了比父类方法更大的异常,比如Exception //则代码将无法编译…… public void test() throws FileNotFoundException { //... } } + // UsingExceptions.java // Demonstrating the getMessage and printStackTrace // methods inherited into all exception classes. public class PrintExceptionStack { public static void main( String args[] ) { try { method1(); } catch ( Exception e ) { System.err.println( e.getMessage() + " " ); e.printStackTrace(); } } public static void method1() throws Exception { method2(); } public static void method2() throws Exception { method3(); } public static void method3() throws Exception { throw new Exception( "Exception thrown in method3" ); } } public class SystemExitAndFinally { public static void main(String[] args) { try{ System.out.println("in main"); throw new Exception("Exception is thrown in main"); //System.exit(0); } catch(Exception e) { System.out.println(e.getMessage()); System.exit(0); } finally { System.out.println("in finally"); } } }
ThrowDemo.java public class ThrowDemo { public static void main(String[] args) { // try { double data = 100 / 0.0; System.out.println("浮点数除以零:" + data); // if(String.valueOf(data).equals("Infinity")) // { // System.out.println("In Here" ); // throw new ArithmeticException("除零异常"); // } // } // catch(ArithmeticException e) { // System.out.println(e); // } } }
import java.io.*; public class ThrowMultiExceptionsDemo { public static void main(String[] args) { try { throwsTest(); } catch(IOException e) { System.out.println("捕捉异常"); } } private static void throwsTest() throws ArithmeticException,IOException { System.out.println("这只是一个测试"); // 程序处理过程假设发生异常 throw new IOException(); //throw new ArithmeticException(); } }