概述
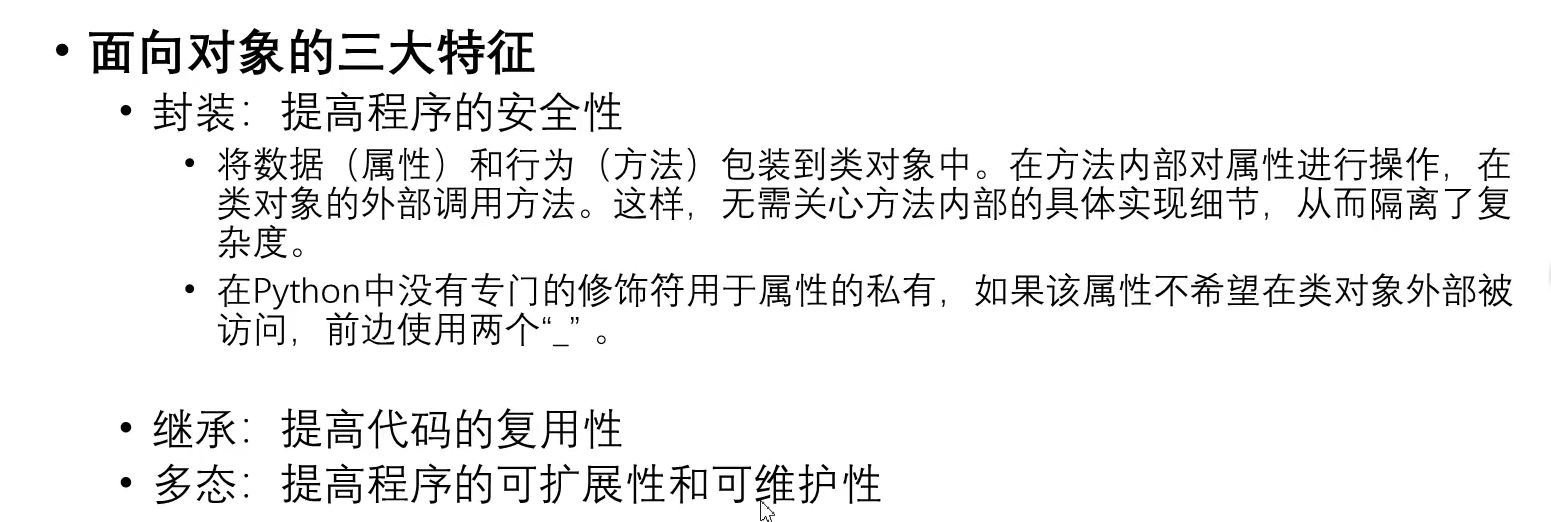
封装
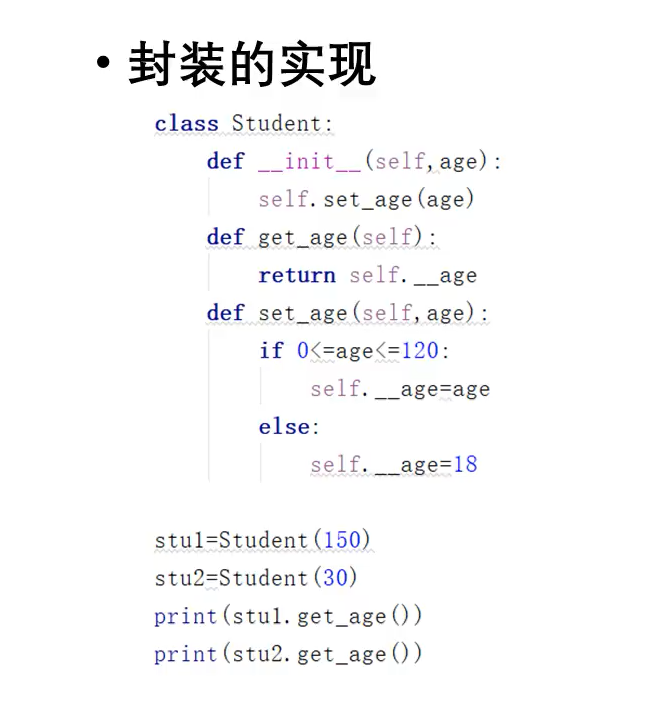
1 #
2 # @author:浊浪
3 # @version:0.1
4 # @time: 2021/4/16 9:13
5 #
6
7 class Student:
8 def __init__(self, name, age):
9 self.name = name
10 self.__age = age
11 def show(self):
12 print(self.name, self.__age)
13
14
15 stu = Student('张三', 20)
16 stu.show()
17
18 # 在类的外部调用属性
19 print(stu.name)
20 # print(stu.__age) #AttributeError: 'Student' object has no attribute '__age'
21
22 print(dir(stu)) # 查看所有的属性和方法
23 print(stu._Student__age) # 在类的外部也可以使用
继承
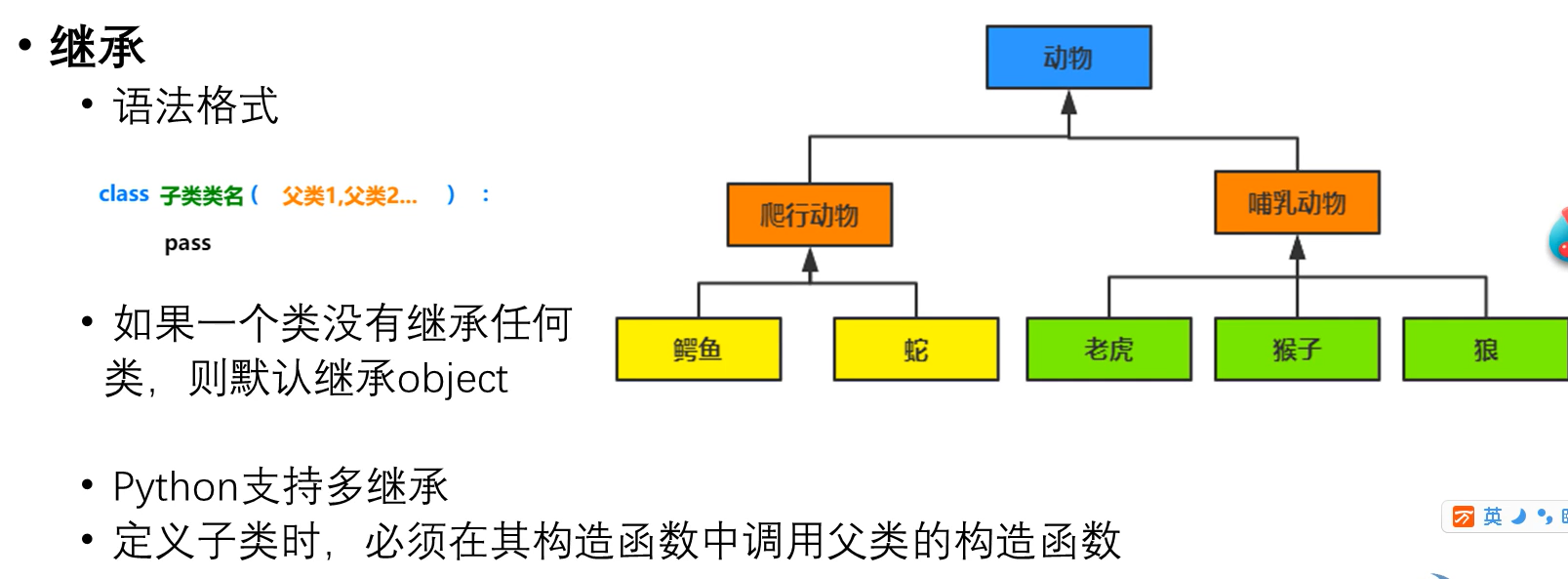
1 #
2 # @author:浊浪
3 # @version:0.1
4 # @time: 2021/4/16 9:31
5 #
6
7 class Person(object):
8 def __init__(self, name, age):
9 self.name = name
10 self.age = age
11 def info(self):
12 print(self.name, self.age)
13
14 class Student(Person):
15 def __init__(self,name, age, stu_no):
16 super().__init__(name, age)
17 self.stu_no = stu_no
18
19 class Teacher(Person):
20 def __init__(self,name, age, tea_y):
21 super(Teacher, self).__init__(name, age)
22 self.tea_y = tea_y
23
24 stu = Student('张三', 18, 20001)
25 tea = Teacher('李四', 40, 18)
26 stu.info()
27 tea.info()
方法重写
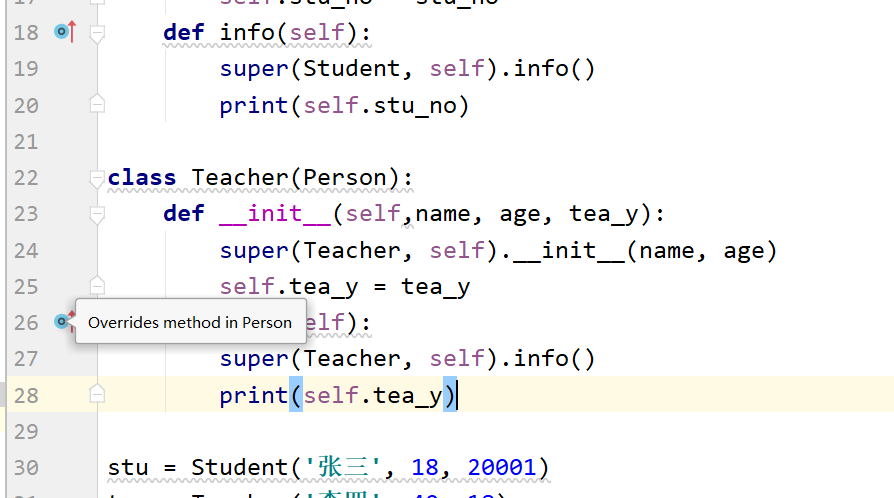
objec类
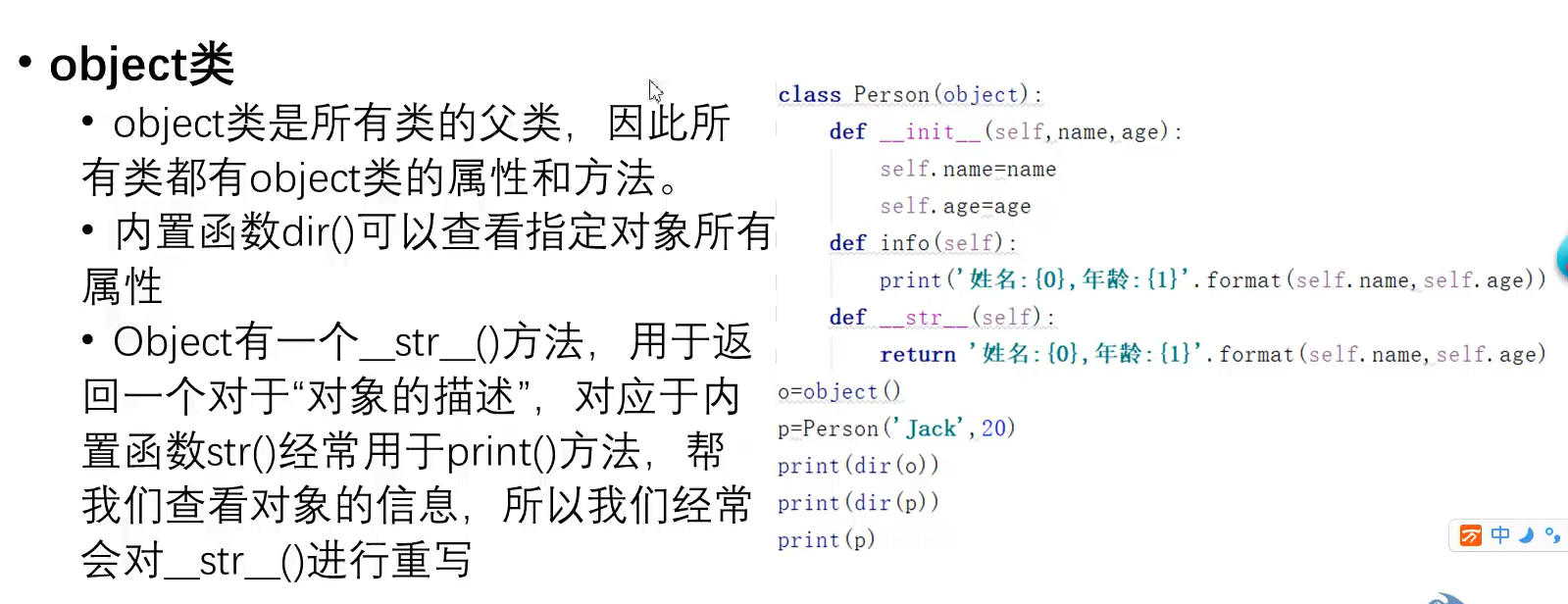
多态
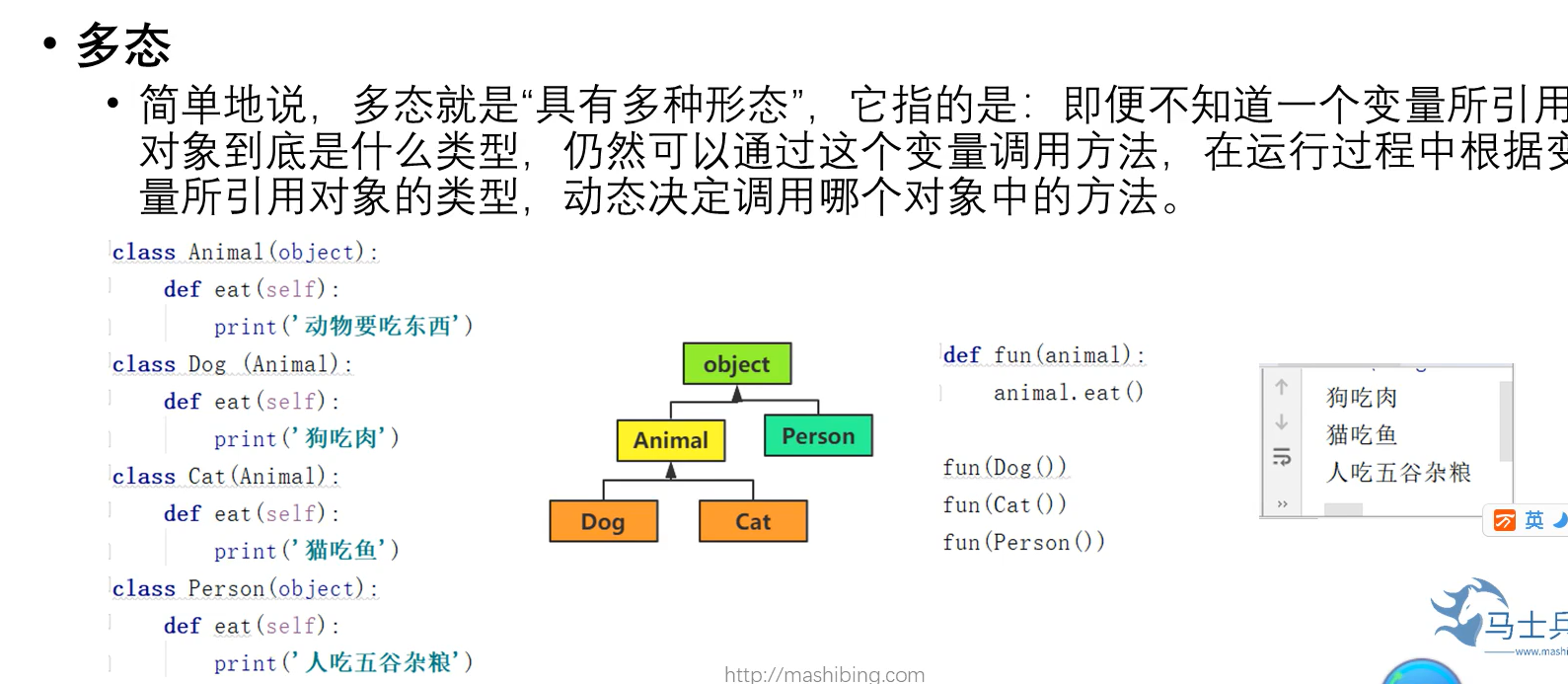
1 #
2 # @author:浊浪
3 # @version:0.1
4 # @time: 2021/4/16 10:46
5 #
6
7 class Animal(object):
8 def eat(self):
9 print('动物吃。。。')
10
11 class Dog(Animal):
12 def eat(self):
13 print('狗吃肉。。。')
14
15 class Cat(Animal):
16 def eat(self):
17 print('猫吃鱼。。。')
18
19 class Person(object):
20 def eat(self):
21 print('人吃很多东西。。')
22
23
24
25 # 定义一个函数
26
27 def fun(obj):
28 obj.eat()
29
30 fun(Cat())
31 fun(Dog())
32 fun(Animal())
33 print('----------------------')
34 fun(Person())
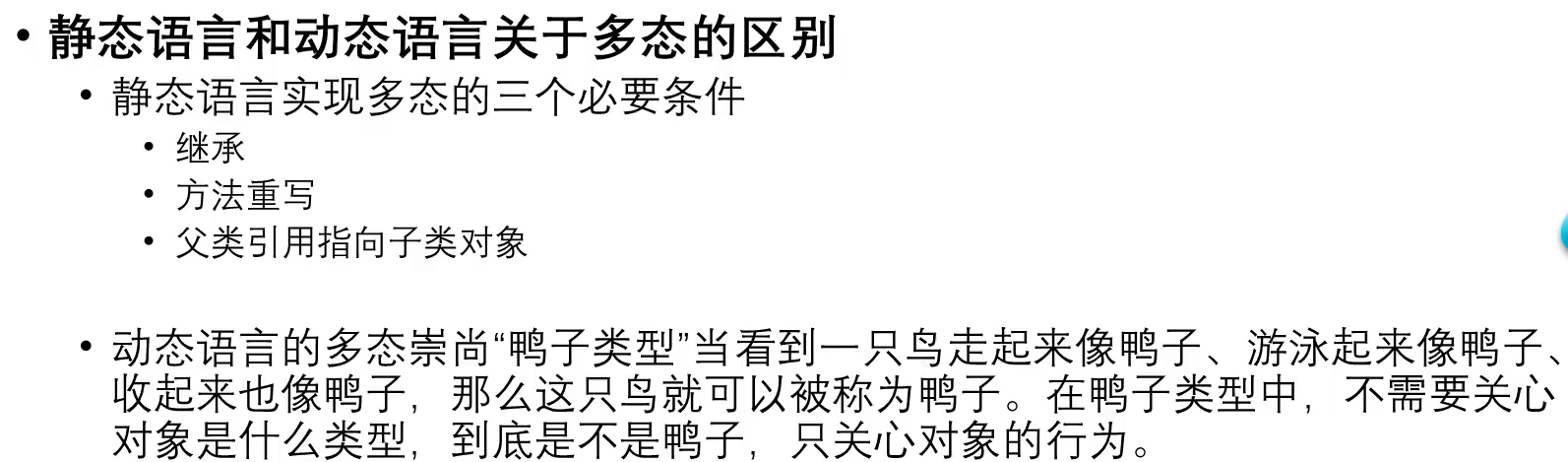