Catalog
Application Domain Introduction
How to create and unloading Application Domain
How to Call Remote Application Domain Method
How to make Multiple applications in a single process running OS
Application Domain Introduction
Application domain is a kind of nature, isolation; the purpose is to enable applications to run the code cannot directly access other applications or funding source. Application domain for the security, reliability, version control, and set uninstall program provides isolation border. Application domain is usually created by the runtime host; Runtime host is responsible for running the application before the common language runtime guide. Application domain is the operating system and runtime environment would normally be provided in the application of some form of isolation.
How to create and unload Application Domain
The .NET Framework provides an AppDomain category for the implementation of managed code to provide isolation, unloading and security of the border. The following code shows how to create and unload an Application Domain.
/// <summary>
/// This method shows how to create and unloading an Application Domain
/// </summary>
public static void CreateAndUnloadAppDomain()
{
AppDomainSetup info = new AppDomainSetup();
info.LoaderOptimization = LoaderOptimization.SingleDomain;
AppDomain domain = AppDomain.CreateDomain("MyDomainTest", null, info);
domain.ExecuteAssembly("E:\\CSharpStudy\\Data Structure\\MyLinkedList\\MyLinkedList\\bin\\Debug\\MyLinkedList.exe");
AppDomain.Unload(domain);
}
In the above code, the class AppDomainSetup used to define a new AppDomain. An example is used to load SingleDomain said in the application process may not be shared between the domain of internal resources; you can also use MultiDomain, MultiDomainHost and other options.
The following code shows an AppDomain that used the MultiDomain to create AppDomin.
/// <summary>
/// This method shows how to create and unloading an Application Domain
/// </summary>
public static void CreateAndUnloadingAppDomain1()
{
AppDomainSetup info = new AppDomainSetup();
info.LoaderOptimization = LoaderOptimization.MultiDomain;
AppDomain domain = AppDomain.CreateDomain("MyDomainTest", null, info);
Console.WriteLine("The result of the first application exceted.");
domain.ExecuteAssembly("E:\\CSharpStudy\\Data Structure\\MyLinkedList\\MyLinkedList\\bin\\Debug\\MyLinkedList.exe");
Console.WriteLine("The result of the second application exceted.");
domain.ExecuteAssembly(@"E:\CSharpStudy\test\test\bin\Debug\test.exe");
AppDomain.Unload(domain);
}
In the above code, we can see there are two application excuted.
The statement “AppDomain.Unload(domain);” used to unloading the Application Domain.
All of the above example is shows to excute an application of the remote application domain.
How to Call Remote Application Domain Method
If there is no the Application Domain, Call other Applications Domain method usually means to carry out the process of inter-call. First, we have to create a simple class and an assemble with library type. The following class RemoteHello used to create an assemble will be called by Remote Application Domain.
using System;
namespace AppDomainTest
{
public class RemoteHello:MarshalByRefObject
{
string messages;
public RemoteHello(string messages)
{
this.messages = messages;
}
public void ShowMessages()
{
Console.WriteLine("Hello, " + messages);
}
}
}
In the above code, class RemoteHello inherited from the MarshalByRefObject class. MarshalByRefObject class is an abstract class, it can allow access object between Application Domains. Now we have to compile the code RemoteHello.cs to RemoteHello.dll.
The following code shows how to load the DDL assembly to an user define Application Domain.
using System;
using System.Reflection;
using System.Runtime.Remoting;
namespace AppDomainTest
{
class RemotingAppDomain
{
public static void Main()
{
//Set up information regarding the application domain
AppDomainSetup info = new AppDomainSetup();
info.ApplicationBase = Environment.CurrentDirectory;
//Create an application domain with null evidence
AppDomain dom = AppDomain.CreateDomain("RemoteDoamin", null, info);
BindingFlags flags = (
BindingFlags.Public
| BindingFlags.Instance
| BindingFlags.CreateInstance
);
ObjectHandle objh = dom.CreateInstance(
"RemoteHello"
, "AppDomainTest.RemoteHello"
, true
, flags
, null
, new string[] { "you have a good job." }
, null
, null
, null
);
object obj = objh.Unwrap();
//Cast to the actual type
RemoteHello h = (RemoteHello)obj;
//Invoke the method
h.ShowMessages();
//Clean up by unloading the application domain
AppDomain.Unload(dom);
}
}
}
To compile in the above code and execute, the result is:
How to make Multiple applications in a single process running OS
Using the Application Domain as a unit, it can make multiple applications in a single process running OS, and it does not affect other applications to stop an application. Now we create two assembly to have a demonstrate of how to make multiple applications in a single process running OS.
using System;
using System.Threading;
namespace Test1
{
class App1
{
static void Main(string[] args)
{
//Create a Thread
Thread t = new Thread(new ThreadStart(App1.Run));
t.Start();//Start a Thread
}
public static void Run()
{
while (true)
{
Thread.Sleep(400); //Let Thread sleep 400ms
Console.WriteLine("The App1 Running...");
}
}
}
}
Compile in the above application for creating an assembly.
csc App1.csc
using System;
using System.Threading;
namespace Test1
{
class App2
{
static void Main(string[] args)
{
//Create a Thread
Thread t = new Thread(new ThreadStart(App2.Run));
t.Start();//Start a Thread
}
public static void Run()
{
while (true)
{
Thread.Sleep(400); //Let Thread sleep 400ms
Console.WriteLine("The App2 Running...");
}
}
}
}
Compile in the above application for creating an assembly.
csc App2.csc
using System;
using System.Threading;
using System.Reflection;
namespace Test1
{
class TestApp
{
AppDomain dom1;
AppDomain dom2;
public TestApp()
{
AppDomainSetup info = new AppDomainSetup();
info.ApplicationBase = Environment.CurrentDirectory;
//Create an application domain with null evidence
dom1 = AppDomain.CreateDomain("RemoteDomain1", null, info);
//Create an application domain with null evidence
dom2 = AppDomain.CreateDomain("RemoteDomain2", null, info);
dom1.ExecuteAssembly(@"D:\WinForm\Remoting2\Test1\App1.exe");
dom2.ExecuteAssembly(@"D:\WinForm\Remoting2\Test1\App2.exe");
}
static void Main(string[] args)
{
//Create a Thread
Thread t = new Thread(new ThreadStart(new TestApp().Run));
t.Start();//Start the Thread
}
public void Run()
{
Thread.Sleep(1000);//Let Thread sleep 1000ms
Console.WriteLine("Unloading dom1...");
AppDomain.Unload(dom1);//Unloading the dom1
Thread.Sleep(1000);
Console.WriteLine("Unloading dom2...");
AppDomain.Unload(dom2);//Unloading the dom2
}
}
}
Compile in the above application for creating an assembly.
csc TestApp.csc
The result of TestApp.exe run is like following picture.
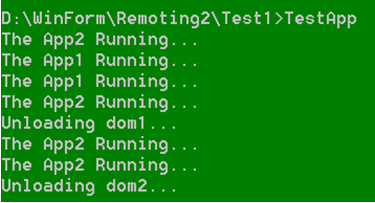
Reference
1. .NET For Java Developers Migrating to C#
2. Microsoft .NET Framework 2.0 SDK Document