全局广播
前提: 先具备良好的 RabbitMQ 环境
1. 演示广播效果,增加复杂度,再以3355为模板再制做一个3366模块
<!--pom.xml--> <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>cloud2020</artifactId> <groupId>com.atguigu.springcloud</groupId> <version>1.0-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>cloud-config-client-3366</artifactId> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> </project>
#bootstrap.yml server: port: 3366 spring: application: name: config-client cloud: #Config客户端配置 config: label: master #分支名称 name: config #配置文件名称 profile: dev #读取后缀名称 上述3个综合:master分支上config-dev.yml的配置文件被读取 http://config-3344.com:3344/master/config-dev.yml uri: http://localhost:3344 #配置中心地址 #服务注册到eureka地址 eureka: client: service-url: defaultZone: http://localhost:7001/eureka #暴露监控端点 management: endpoints: web: exposure: include: "*"
//主启动类 package com.atguigu.springcloud; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; @SpringBootApplication @EnableEurekaClient public class ConfigClientMain3366 { public static void main(String[] args) { SpringApplication.run(ConfigClientMain3366.class,args); } }
//controller package com.atguigu.springcloud.config; import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.context.config.annotation.RefreshScope; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RefreshScope public class ConfigClientController { @Value("${server.port}") private String serverPort; @Value(("${config.info}")) private String configInfo; @GetMapping("/configInfo") public String getConfigInfo(){ return "serverPort: "+serverPort+" configInfo: "+configInfo; } }
2. 讲一下设计思想
1)利用消息总线触发一个客户端/bus/refresh,而刷新所有客户端的配置
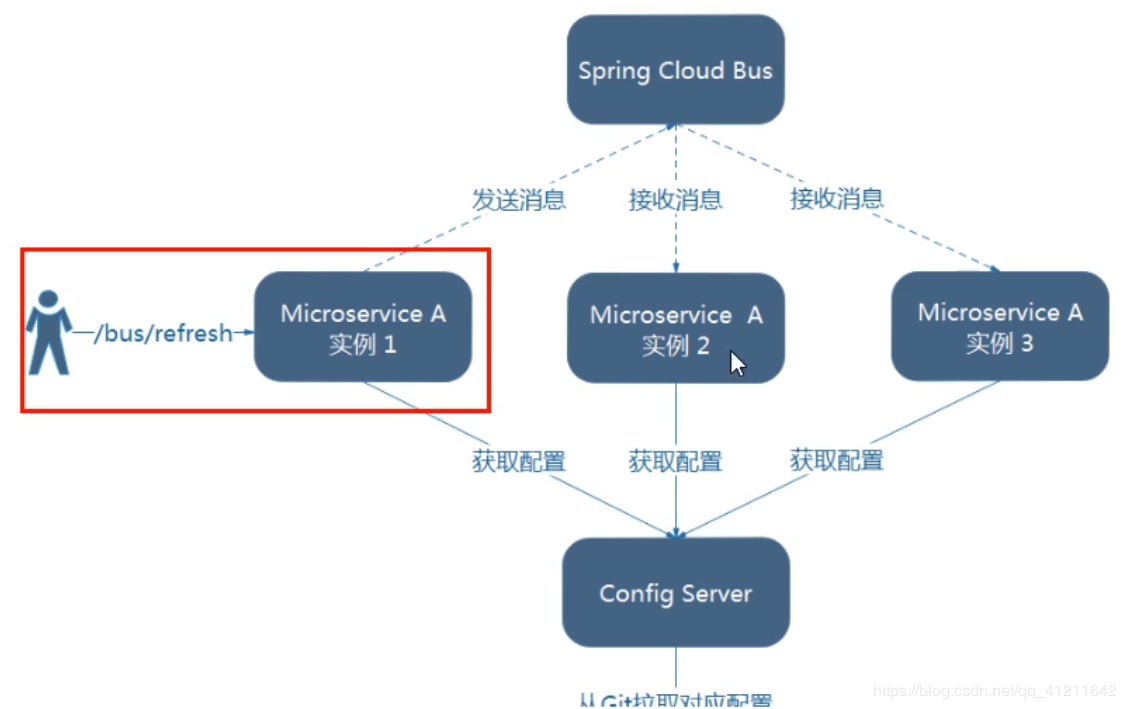
2)利用消息总线触发一个服务端ConfigServer的/bus/refresh端点,而刷新所有客户端的配置
图二的架构显然更加合适,图一不合适的原因如下:
打破了微服务的职责单一性,因为微服务本身是业务模块,它本不应该承担配置刷新的职责
破坏了微服务各节点的对等性
有一定的局限性。例如,微服务在迁移时,它的网络地址常常会发生变化,此时如果想要做到自动刷新,那就好增加更多的修改
3. 给cloud-config-center-3344配置中心服务端端架消息总线支持
<!--添加消息总线RbbitMQ支持--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
#rabbit相关配置 rabbitmq: host: localhost port: 5672 username: guest password: guest #rabbitmq相关配置,暴露bus刷新配置的端点 management: endpoints: #暴露bus刷新配置的端点 web: exposure: include: 'bus-refresh' #凡是暴露监控、刷新的都要有actuator依赖,bus-refresh就是actuator的刷新操作
4. 给cloud-config-client-3355客户端添加消息总线支持
<!--添加消息总线rabbitMQ支持--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
#rabbit相关配置 15672是web管理界面的端口,5672是MQ访问的端口 rabbitmq: host: localhost port: 5672 username: guest password: guest #这是客户端,不需要刷新
5. 给cloud-config-client-3366客户端添加消息总线支持
<!--添加消息总线rabbitMQ支持--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
#rabbit相关配置 15672是web管理界面的端口,5672是MQ访问的端口 rabbitmq: host: localhost port: 5672 username: guest password: guest #这是客户端,不需要刷新
6. 启动7001、3344、3355、3366,测试
先看一下eureka注册中心,3344,3355,3366都已经注册进来了
github上现在version 是3,访问3344,看拿到的是多少
是3,正常,访问3355
正常,访问3366
现在,在github将version改为4,如果不使用Bus消息总线通知,3344可以拿到新数据:
3355/3366要想拿到新数据需要每个都发送一个Post请求激活,但现在使用了Bus总线,我们只需给3344服务端发送一次Post请求,3355/3366就能自动的跟着更新。测试一下,给3344发送Post:
然后测试3355、3366,这时候已经可以拿到新数据了,但我们并没有重启3355/3366,或给每一个发送post请求
这样就达到了一次修改,广播通知,处处生效。
定点通知
如果现在不想全部通知,指向定点通知该如何呢?
解决:
指定具体某一个实例生效而不是全部
公式:http://localhost:配置中心端口号/actuator/bus-refresh/{destination}
/bus/refresh请求不再发送到具体的服务实例上,而是发给 config server并通过destination参数类指定需要更新配置的服务或实例
案例: 这里我们以刷新运行在3355端口上的config-client为例,只通知3355,不通知3366
现在再来修改github配置文件的version,改为5
现在3344是可以直接拿到新数据,3355/3366不能,我们来发一条这样的Post请求
curl -X -POST "http://localhost:3344/actuator/bus-refresh/config-client:3355"
config-client就是3355的微服务名,3355即端口号
现在来刷新3355、3366,只有3355拿到了新数据,3366没被通知,没拿到新数据
通知总结