小记:人最痛苦的事情,就是我原本可以...
言归正传,直接插入排序的基本思想:
将一个记录插入到已排序好的有序表中,从而得到一个新,记录数增1的有序表。即:先将序列的第1个记录看成是一个有序的子序列,然后从第2个记录逐个进行插入,比较前面元素之间大小,交换元素保证子序列有序。子序列有序后,再加入后面的元素,成为一个新的序列,然后遍历这个新的序列,调整加入的元素在合适的位置,使这个新的序列有序。直至整个序列有序为止。排序后相等的元素前后顺序不会发生变化,所以这种排序方法是稳定。 时间复杂度:O(n2)。
假设有一个数组 int[] list={15,8,45,6,8,12,7,9,2},对它进行排序,每次加入新的元素,用“()”括起来表示。排序的顺序如下图:
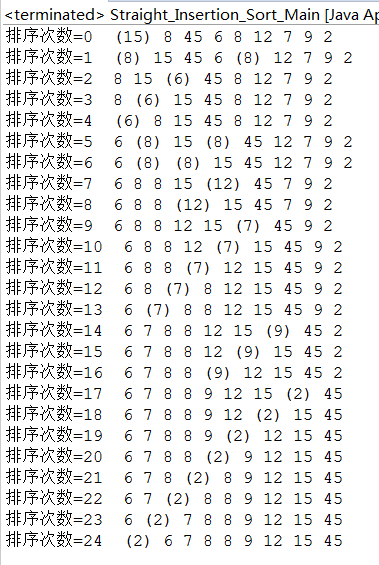
以前一直从事.NET开发,最近可能接受Java的项目,所以这次用java代码学习,所以一些理解不到位的地方,请多不吝指教。
1 //直接插入排序 2 public class Straight_Insertion_Sort_Main { 3 4 /*将一个记录插入到已排序好的有序表中, 5 * 从而得到一个新,记录数增1的有序表。 6 * 即:先将序列的第1个记录看成是一个有序的子序列,然后从第2个记录逐个进行插入, 7 * 直至整个序列有序为止。*/ 8 public static int recorde=0;//显示交换次数,和算法无关 9 public static int forIndex=0;//循环次数统计,和算法无关无效值 10 public static void print(int[] list,int change) 11 { 12 int len=list.length; 13 System.out.print("排序次数="+recorde+" "); 14 recorde++; 15 for(int index=0;index<len;index++) 16 { 17 if(list[index]==change) 18 { 19 System.out.print("("+list[index]+") "); 20 } 21 else 22 { 23 System.out.print(list[index]+" "); 24 } 25 if(index==len-1) 26 { 27 System.out.println(""); 28 } 29 } 30 } 31 32 public static void Sort(int[] list) 33 { 34 int len=list.length; 35 print(list,list[0]); 36 forIndex++; 37 for(int index=1;index<len;index++) 38 { 39 if(list[index]<list[index-1]) 40 { 41 int last=list[index]; 42 list[index]=list[index-1]; 43 list[index-1]=last;//将最大的数放在最后 44 print(list,last); 45 int reindex=index-1; 46 while(reindex>0)//同时向前遍历,保证数组的有序 47 { 48 forIndex++; 49 if(list[reindex-1]>last) 50 { 51 list[reindex]=list[reindex-1]; 52 list[reindex-1]=last; 53 54 print(list,last); 55 } 56 else// 57 { 58 break; 59 } 60 reindex--; 61 62 } 63 } 64 } 65 } 66 67 68 public static void main(String[] args) { 69 // TODO Auto-generated method stub 70 int[] list={15,8,45,6,8,12,7,9,2}; 71 Sort(list); 72 } 73 74 }
参考链接地址:http://blog.csdn.net/hguisu/article/details/7776068