Ultra-QuickSort
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 34470 | Accepted: 12382 |
Description
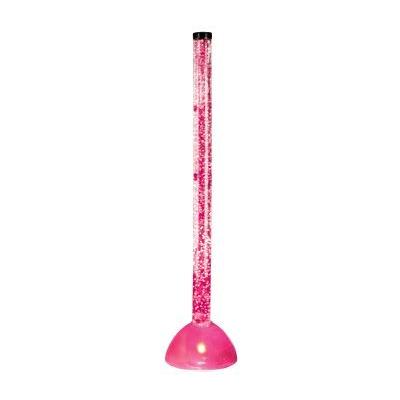
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
题目大意:给出一段数字序列,每次只能交换相邻的两个数,问最少需要多少次可以使得这段序列变成顺序序列。
思路:用冒泡排序超时,因为给的数据量很大。用归并排序的方法可以求出逆序数,在这里,逆序数就是冒泡排序的交换次数,所以这道题目就是用归并排序的方法对数组进行排序,并得出逆序数,输出即可。
代码:

1 #include<iostream> 2 #include<string.h> 3 #include<cstdio> 4 #include<cstdlib> 5 #define max 1000000 6 using namespace std; 7 int n,f[max],g[max]; 8 long long int sum=0; 9 void guibing(int l,int mid,int r) 10 { 11 int t=0; 12 int i=l,j=mid+1; 13 while(i<=mid&&j<=r) 14 { 15 if(f[i]<=f[j]) 16 { 17 g[t++]=f[i]; 18 i++; 19 } 20 else 21 { 22 g[t++]=f[j]; 23 j++; 24 sum=sum+mid-i+1; 25 } 26 } 27 while(i<=mid)g[t++]=f[i++]; 28 while(j<=r)g[t++]=f[j++]; 29 for(i=0;i<t;i++) 30 f[l+i]=g[i]; 31 } 32 void quicksort(int l,int r) 33 { 34 if(l<r) 35 { 36 int mid=(l+r)/2; 37 quicksort(l,mid); 38 quicksort(mid+1,r); 39 guibing(l,mid,r); 40 } 41 } 42 int main() 43 { 44 while(1) 45 { 46 cin>>n; 47 if(n==0)break; 48 int i; 49 sum=0; 50 for(i=0;i<=n-1;i++) 51 cin>>f[i]; 52 quicksort(0,n-1); 53 cout<<sum<<endl; 54 } 55 return 0; 56 }