2020-04-09
设计循环队列
设计你的循环队列实现。 循环队列是一种线性数据结构,其操作表现基于 FIFO(先进先出)原则并且队尾被连接在
队首之后以形成一个循环。它也被称为“环形缓冲器”
循环队列的一个好处是我们可以利用这个队列之前用过的空间。在一个普通队列里,一旦一个队列满了,
我们就不能插入下一个元素,即使在队列前面仍有空间。但是使用循环队列,我们能使用这些空间去存储新的值。
你的实现应该支持如下操作:
- MyCircularQueue(k): 构造器,设置队列长度为 k 。
- Front: 从队首获取元素。如果队列为空,返回 -1 。
- Rear: 获取队尾元素。如果队列为空,返回 -1 。
- enQueue(value): 向循环队列插入一个元素。如果成功插入则返回真。
- deQueue(): 从循环队列中删除一个元素。如果成功删除则返回真。
- isEmpty(): 检查循环队列是否为空。 isFull(): 检查循环队列是否已满。
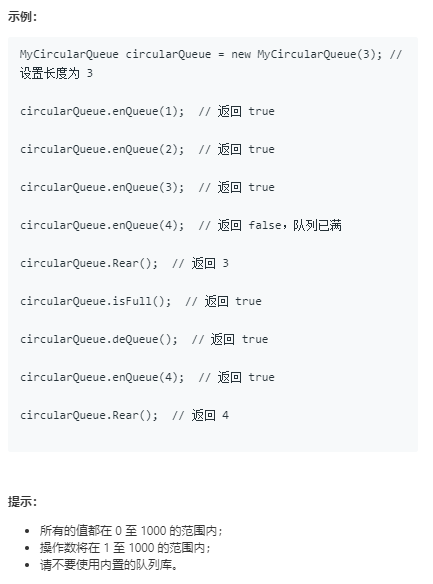
题解:
思路1: 数组
var MyCircularQueue = function (k) { this.length = k; // 原型中存new传入的k 记录这个quene的最大长度 this.queue = []; // queue数组 }; MyCircularQueue.prototype.enQueue = function (value) { if (this.queue.length < this.length) { // 如果queue的长度比最大长度小 则可以插入 this.queue.push(value); return true; } return false; }; MyCircularQueue.prototype.deQueue = function () { if (this.queue.length > 0) { // 如queue有的长度不为0 说明有值 把第一个删掉(先进先出) this.queue.shift(); return true; } return false; }; MyCircularQueue.prototype.Front = function () { if (this.queue.length > 0) return this.queue[0]; return -1; }; MyCircularQueue.prototype.Rear = function () { if (this.queue.length > 0) return this.queue[this.queue.length - 1]; return -1; }; MyCircularQueue.prototype.isEmpty = function () { return this.queue.length === 0; };
cularQueue.prototype.isFull = function () { return this.queue.length === this.length; };