我做了一个查询单词的简单app, 当在EditText中输入单词的时候,点击lookup,则在TextView区域显示出该单词的意思,当EditText中没有任何字符时,显示"word definition result", 注意,单词的最大长度限制是20个字符,且禁止回车键(换行键);
这个里面需要用到读文件操作、禁止键盘回车键、Textwatcher监听、限制输入长度、提示语等操作!
1. 首先需要一个Lookup的button, 一个可以输入字符的EditText 和一个显示单词结果的TextView;
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.chenye.dictionary.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/wordEditText" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:hint="input a word" android:maxLength="20" android:singleLine="true"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="lookup" android:onClick="lookup"/> </LinearLayout> <TextView android:id="@+id/result" android:layout_width="match_parent" android:layout_height="match_parent" android:text="word definition result" android:textSize="20dp"/> </LinearLayout>
其中android:singleLine="true"为禁止虚拟键盘的操作; android:maxLength="20"为限制最大长度;android:hint="input a word"为提示语;这里EditText和button部分是水平布局,而它俩与TextView显示结果区域是垂直布局;
2. java代码;
package com.chenye.dictionary; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.text.Editable; import android.text.TextWatcher; import android.util.Log; import android.view.View; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import java.util.HashMap; import java.util.Scanner; public class MainActivity extends AppCompatActivity { private HashMap<String, String> dictionary; EditText wordEditText; TextView resultTextView; public void readAllDefinition() { Scanner scanner = new Scanner(getResources().openRawResource(R.raw.gre_words)); if(this.dictionary == null){ this.dictionary = new HashMap<>(); } while (scanner.hasNext()){ String line = scanner.nextLine(); String[] pieces = line.split(" "); this.dictionary.put(pieces[0], pieces[1]); } scanner.close(); } @Override protected void onCreate (Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); readAllDefinition(); wordEditText = findViewById(R.id.wordEditText); resultTextView = findViewById(R.id.result); wordEditText.addTextChangedListener(new TextWatcher() { private CharSequence word; @Override public void beforeTextChanged(CharSequence charSequence, int start, int count, int after) { word = charSequence; } @Override public void onTextChanged(CharSequence charSequence, int start, int before, int count) { //resultTextView.setText(""); } @Override public void afterTextChanged(Editable editable) { if(word.length()==0) { resultTextView.setText("word definition result"); } else if (word.length() == 20){ Toast.makeText(MainActivity.this, "已到达输入的最大长度!", Toast.LENGTH_SHORT).show(); } } }); } // button的方法 public void lookup(View view) { EditText wordText = findViewById(R.id.wordEditText); String word = wordText.getText().toString(); if(this.dictionary == null){ readAllDefinition(); } String def = this.dictionary.get(word); TextView defTextView = findViewById(R.id.result); if(def == null){ defTextView.setText("word not fond!"); }else{ defTextView.setText(def); } } }
readAllDefinition()是在app启动的时候就会被调用的方法,此时已经将所有的单词,以及其对应的含义放在了map中;
其中,getResources().openRawResource(R.raw.gre_words)方法获取到InputStream流, gre_words是存放单词及对应含义的文本文件;
onCreate()方法中,分别采用wordEditText = findViewById(R.id.wordEditText);resultTextView = findViewById(R.id.result)方法获取id
最重要的是addTextChangedListener这个方法,是监听EditText的方法,里面有三个方法:
beforeTextChange()是在EditText改变之前里面可以做一些操作;
onTextChanged()是改变中;
afterTextChanged()是改变后;
参数:charSequence是这串字符串;start表示开始变化的位置,count表示变化的字符串长度,after表示变化之后该位置的字符数量, before表示变化之前该位置的字符数量;
3. 结果如下:
初始情况: 查询单词如下: 删除单词中间结果如下:
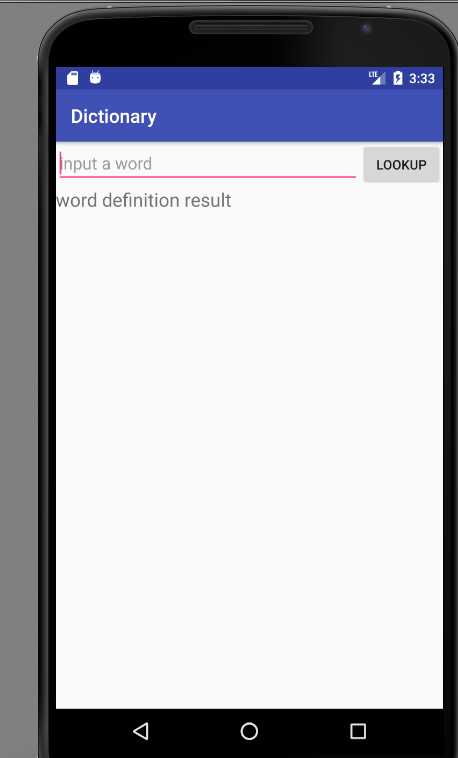
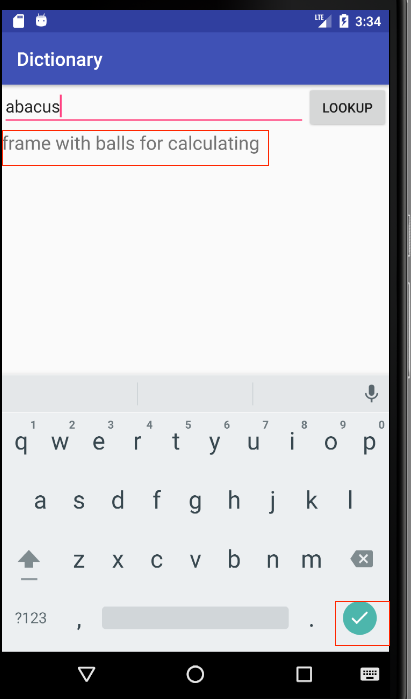

长度超出情况如下:
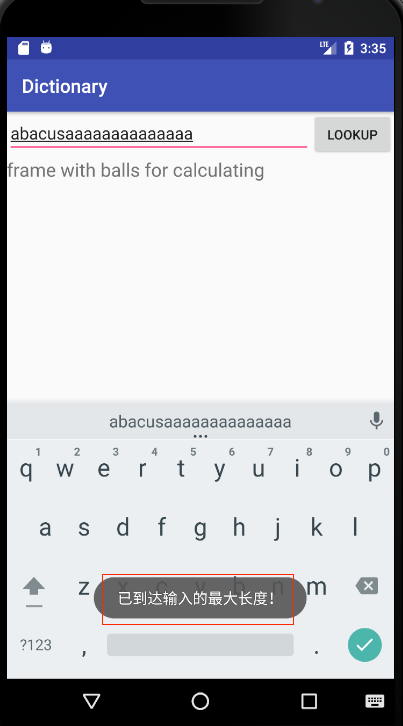
ps: 本宝也是刚开始学习android, 记录一下自己的学习过程,好记性不如烂笔头!