VUE中 父组件给子组件传值流程
1.创建父组件以及子组件;
2.在父组件中引入子组件;
3.在父组件中使用子组件;
4.在子组件标签上绑定指令,指定内容为需要传递的值;
5.在子组件内部使用props属性接收值,并绑定在子组件标签上;
VUE中 子组件给父组件传值流程
1.创建父组件以及子组件;
2.在父组件中引入子组件;
3.在父组件中使用子组件;
4.在子组件中使用:this.$emit("fromChildB", this.toMsg),”fromChildB“为父组件中绑定的指令名,”this.toMsg“为需要传递的参数;
5.在父组件中子组件标签上绑定函数:@fromChildB="fromChildB",”@fromChildB“为子组件中触发的第一个参数,”fromChildB“为接收的方法名;
6.在父组件methods钩子中:定义方法”fromChildB“,接收参数e,e为子组件传递的参数
VUE中 兄弟组件之间传值流程(中央事件总线BUS)
1.创建父组件以及子组件;
2.在父组件中引入子组件;
3.在父组件中使用子组件;
4.在父组件中创建中央事件总线BUS:Vue.prototype.bus = new Vue();
4.在子组件A中使用:this.bus.$emit('childAToChildB',this.toMsg);,”childAToChildB“为B组件中on指令的第一个参数,”this.toMsg“为需要传递的参数;
5.在组件B created钩子中:this.bus.$on("childAToChildB",this.childAToChildB);”childAToChildB“为A组件中触发的第一个参数,“this.childAToChildB”为指令触发后的回调函数;
6.在组件B methods钩子中:定义方法”childAToChildB“,接收参数e,e为A组件传递的参数;
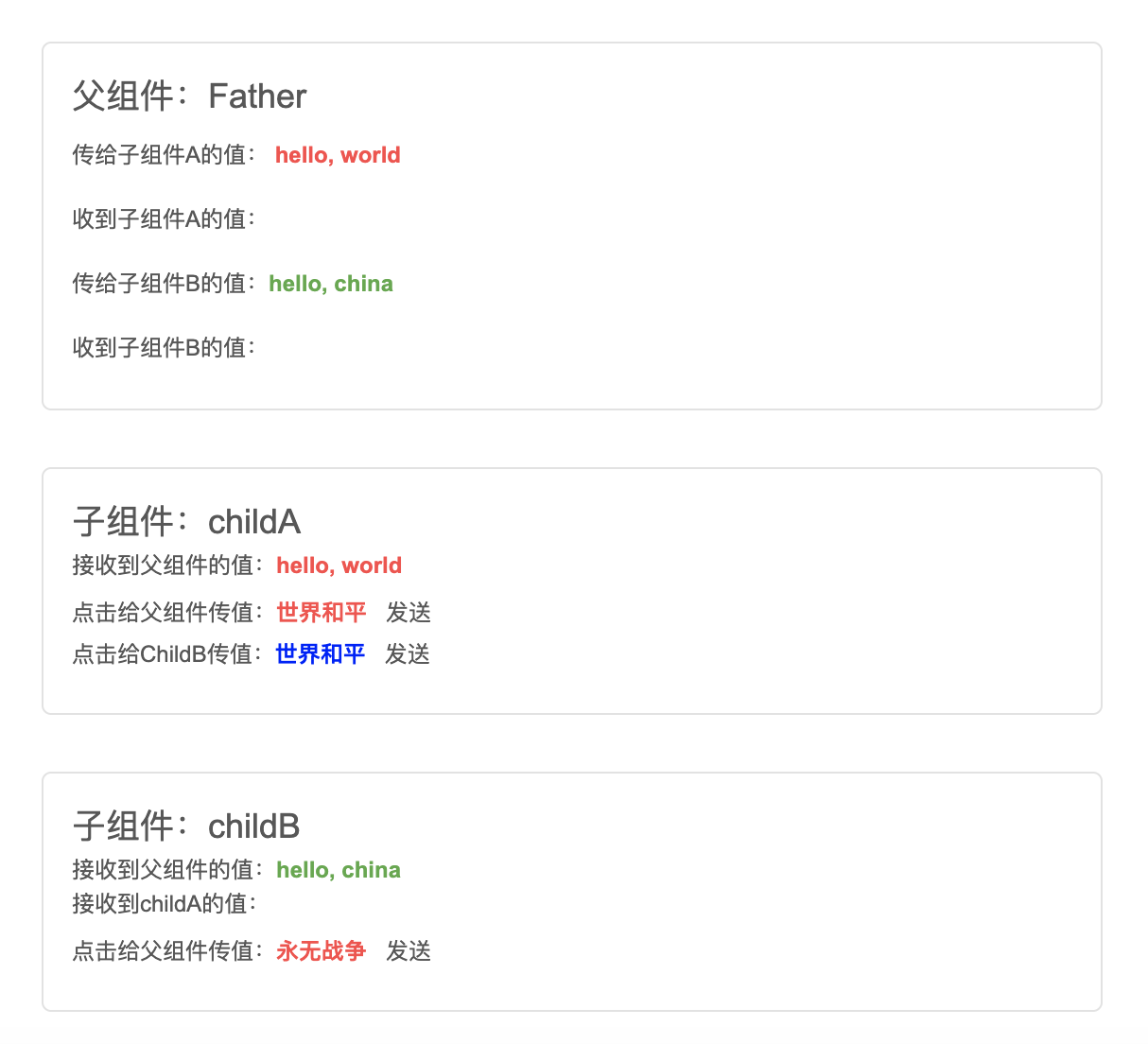
具体代码实现
父组件:Father
<template> <div class="wrap"> <div> <h2>父组件:Father</h2> <p> 传给子组件A的值: <b style="color:#ff4747">{{sendA}}</b> </p> <p> 收到子组件A的值: <b style="color:#ff4747">{{fromA}}</b> </p> <p>传给子组件B的值:<b style="color:#52aa44">{{sendB}}</b></p> <p> 收到子组件B的值: <b style="color:#52aa44">{{fromB}}</b> </p> </div> <div> <childA :msg="sendA" @fromChildA="fromChildA"></childA> </div> <div> <childB :msg="sendB" @fromChildB="fromChildB"></childB> </div> </div> </template> <script> // 引入子组件 import childA from "./childA.vue"; import childB from "./childB.vue"; //定义中央事件总线 import Vue from 'vue'; Vue.prototype.bus = new Vue(); export default { name: "Father", components: { childA: childA, childB: childB }, data() { return { sendA: "hello, world", sendB: "hello, china", fromA: "", fromB: "" }; }, methods: { fromChildA(e) { this.fromA = e; }, fromChildB(e) { this.fromB = e; } } }; </script> <style type="text/css" scoped> .wrap { padding: 20px; } .wrap > div { padding: 15px; margin: 15px; border: 1px solid #e2e2e2; border-radius: 5px; } .wrap > div > p { padding: 8px 0; } </style>
子组件:ChildA
<template> <div class="wrap"> <h2>子组件:childA</h2> <p>接收到父组件的值:<b style="color:#ff4747">{{msg}}</b></p> <div> <p>点击给父组件传值:<b style="color:#ff4747">{{toMsg}}</b><span style="margin-left:10px;cursor:pointer;" @click="sendToFather()">发送</span></p> <p>点击给ChildB传值:<b style="color:blue">{{toMsg}}</b><span style="margin-left:10px;cursor:pointer;" @click="sendToChildB()">发送</span></p> </div> </div> </template> <script> export default { name: "childA", props: ["msg"], data() { return { toMsg: "世界和平" }; }, methods: { sendToFather() { // 向父组件传值 this.$emit('fromChildA',this.toMsg); }, sendToChildB() { // 向兄弟组件传值 this.bus.$emit('childAToChildB',this.toMsg); } } }; </script> <style type="text/css" scoped> .wrap { padding: 0px!important; } .wrap > div { padding: 5px 0; } .wrap > div > p { padding: 2px 0; } </style>
子组件:ChildB
<template> <div class="wrap"> <h2>子组件:childB</h2> <p>接收到父组件的值:<b style="color:#52aa44">{{msg}}</b></p> <p>接收到childA的值:<b style="color:blue">{{fromAmsg}}</b></p> <div> <p>点击给父组件传值:<b style="color:#ff4747">{{toMsg}}</b><span style="margin-left:10px;cursor:pointer;" @click="sendToFather()">发送</span></p> </div> </div> </template> <script> export default { name: "childB", props: ["msg"], data() { return { toMsg: "永无战争", fromAmsg: "", }; }, methods: { sendToFather() { // 向父组件传值 this.$emit("fromChildB", this.toMsg); }, childAToChildB(e){ // 接收传值后的回调 this.fromAmsg = e; } }, created(){ // 接收兄弟组件的传值 this.bus.$on("childAToChildB",this.childAToChildB); } }; </script> <style type="text/css" scoped> .wrap { padding: 0px!important; } .wrap > div { padding: 5px 0; } .wrap > div > p { padding: 2px 0; } </style>