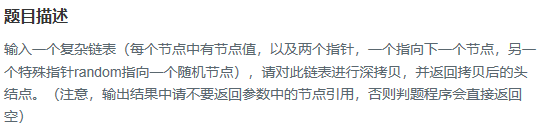
/*
public class RandomListNode {
int label;
RandomListNode next = null;
RandomListNode random = null;
RandomListNode(int label) {
this.label = label;
}
}
*/
import java.util.*;
public class Solution {
public RandomListNode Clone(RandomListNode pHead)
{
if(pHead==null)
return null;
RandomListNode Lphead=pHead;
Map<RandomListNode,RandomListNode> map=new HashMap<>();
while(Lphead!=null){
map.put(Lphead,new RandomListNode(Lphead.label) ) ;
Lphead=Lphead.next;
}
Lphead=pHead;
while(Lphead!=null){
map.get(Lphead).next=map.get(Lphead.next);
Lphead=Lphead.next;
}
Lphead=pHead;
while(Lphead!=null){
map.get(Lphead).random=map.get(Lphead.random);
Lphead=Lphead.next;
}
return map.get(pHead);
}
}

/*
public class ListNode {
int val;
ListNode next = null;
ListNode(int val) {
this.val = val;
}
}*/
public class Solution {
public ListNode Merge(ListNode p1,ListNode p2) {
if(p1==null)
return p2;
if(p2==null)
return p1;
ListNode head=new ListNode(-1);
ListNode L=head;
while(p1!=null && p2!=null){
if(p1.val<p2.val){
L.next=p1;
p1=p1.next;
L=L.next;
}else{
L.next=p2;
p2=p2.next;
L=L.next;
}
}
while(p1!=null){
L.next=p1;
p1=p1.next;
L=L.next;
}
while(p2!=null){
L.next=p2;
p2=p2.next;
L=L.next;
}
return head.next;
}
}
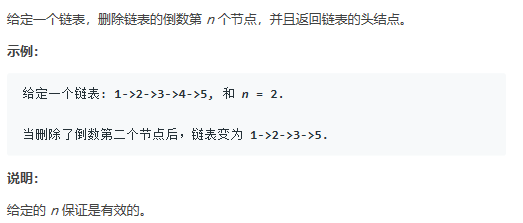
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode(int x) { val = x; }
* }
*/
class Solution {
public ListNode removeNthFromEnd(ListNode head, int n) {
ListNode fast=head;
ListNode slow=head;
for(int i=0;i<n;i++) //首先让快指针走n步
fast=fast.next;
if(fast==null){ //此时说明链表j正好有n个元素,因此需要删除第一个
head=head.next;
}else{
while(fast.next!=null){
fast=fast.next;
slow=slow.next; //满指针到达要删除结点的前一个结点
}
slow.next=slow.next.next; //删除结点
}
return head;
}
}