文件架构
.
├── app
│ ├── connect.py
│ ├── cvv.py
│ ├── date.py
│ ├── hello.py
│ └── picture.jpg
├── Dockerfile
└── requirements.txt
构建python镜像
PyMySQL
opencv-python
FROM python
WORKDIR /app
#工作目录
COPY ./requirements.txt /requirements.txt FROM python
MAINTAINER y00
WORKDIR /app
#工作目录
COPY ./requirements.txt /requirements.txt
#添加依赖文件
RUN pip install -r /requirements.txt -i https://pypi.douban.com/simple
#修改源并安装依赖
ENTRYPOINT [ "python" ]
# 实现命令行式调用容器
CMD [ "hello.py" ]
#设置ENTRYPOINT默认参数
RUN pip install -r /requirements.txt -i https://pypi.douban.com/simple
#修改源并安装依赖
ENTRYPOINT [ "python" ]
# 实现命令行式调用容器
CMD [ "hello.py" ]
#设置ENTRYPOINT默认参数
- 在当前目录构建镜像
sudo docker build -t docker-python .
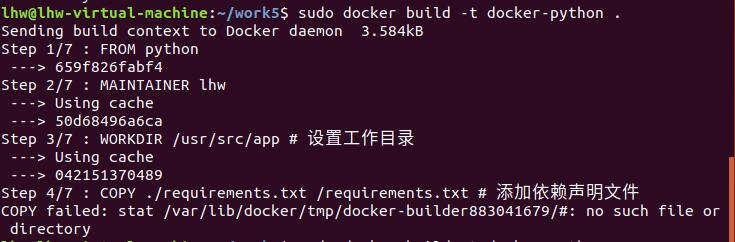
简单程序的部署运行
# hello.py
print('hello world')
sudo docker run --rm -v /home/lhw/work5/app:/app docker-python hello.py

# date.py
import calendar
yy = int(input("输入年份: "))
mm = int(input("输入月份: "))
print(calendar.month(yy,mm))
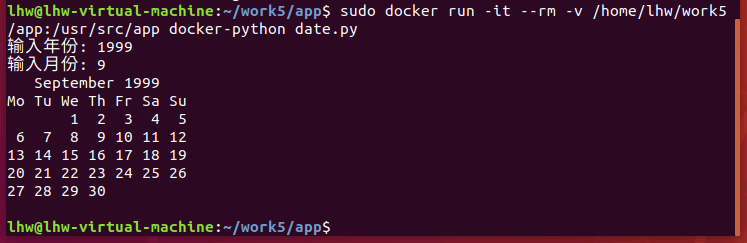
连接数据库并返回版本
#mysql1.py
#!/usr/bin/python3
import pymysql
# 打开数据库连接
db = pymysql.connect(host="mysql",user="root",password="123456",database="myDB" )
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
# 使用 execute() 方法执行 SQL 查询
cursor.execute("SELECT VERSION()")
# 使用 fetchone() 方法获取单条数据.
data = cursor.fetchone()
print ("Database version : %s " % data)
# 关闭数据库连接
db.close()

新建表
#mysql2.py
#!/usr/bin/python3
import pymysql
# 打开数据库连接
db = pymysql.connect(host="mysql",user="root",password="123456",database="myDB" )
# 使用 cursor() 方法创建一个游标对象 cursor
cursor = db.cursor()
# 使用 execute() 方法执行 SQL,如果表存在则删除
cursor.execute("DROP TABLE IF EXISTS EMPLOYEE")
# 使用预处理语句创建表
sql = """CREATE TABLE EMPLOYEE (
FIRST_NAME CHAR(20) NOT NULL,
LAST_NAME CHAR(20),
AGE INT,
SEX CHAR(1),
INCOME FLOAT )"""
cursor.execute(sql)
# 关闭数据库连接
db.close()
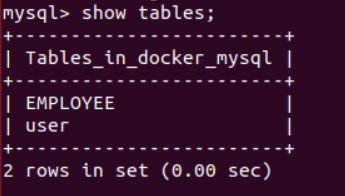
- opencv程序
函数 cv2.moments() 会将计算得到的矩以一个字典的形式返回
#cvv.py
import cv2
import numpy as np
img = cv2.imread('picture.jpg',0)
ret,thresh = cv2.threshold(img,127,255,0)
contours,hierarchy = cv2.findContours(thresh, 1, 2)
cnt = contours[0]
M = cv2.moments(cnt)
print (M)
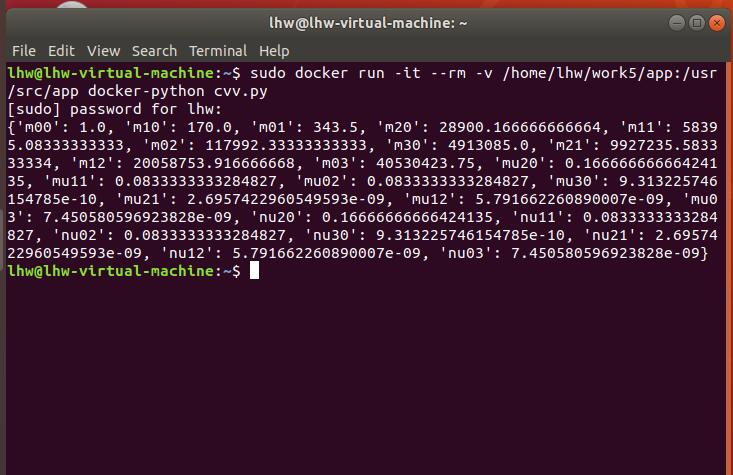