---恢复内容开始---
知识点总结报告
知识点:
栈
(原理)栈是一种只能在一端进行插入删除操作的线性表。栈的主要特点是后进先出。栈的存储可以分为顺序存储(顺序栈)和链式存储(链栈)。
顺序栈
Typedef struct{
ElemType data[Maxsize];//存放栈中数据元素
}
栈空条件 s->top==-1
栈满条件s->top==-1
进栈操作:指针加1,元素放在栈顶
出栈操作:取出栈顶元素放入e,栈顶指针减1
初始化栈initStack(&s)
void InitStack(SqStack *&s)
{ s=(SqStack *)malloc(sizeof(SqStack)); //分配顺序栈空间,首地址放s中
s->top=-1; //栈顶指针置为-1}
销毁栈DestroyStack(&s)
void DestroyStack(SqStack *&s)
{ free(s);
}
判断栈是否为空StackEmpty(s)
bool StackEmpty(SqStack *s)
{ return(s->top==-1);
}
进栈PUSH(&s,e)
bool Push(SqStack *&s, Elemtype e)
{ if(s->top==Maxsize-1) //栈满情况,栈上溢出
return false;
s->top++; //栈顶指针增加1
s->data[s->top]=e; //元素e放在栈顶指针处
return ture;
}
出栈Pop(&s,&e)
bool Pop(SqStack *&s,ElemType &e)
{ if(s->top==-1) //栈空情况,栈下溢出
return false;
e=s->data[s->top]; //取栈顶元素
s->top--; //栈顶指针减1
return ture;
}
取栈顶元素GetTop(s,&e)
bool GetTop(SqStack *s, ElemType &e)
{ if(s->top==-1) //栈空时下溢出
return false;
e=s->data[s->top]; //取栈顶元素
return true;
}
链栈
typedef struct linknode
{ ElemType data; //数据域
struct linknode *next; //指针域
} LinkStNode; //链栈结点类型
栈空条件 s->next==NULL
栈满条件 链栈不考虑栈满
进栈操作:新建结点存放e(p指向它),将结点插入到头结点之后
出栈操作:取出首结点data并删除
初始化栈initStack(&s)
void InitStack(LinkStNode *&s)
{ s=(LinkStNode *)malloc(sizeof(LinkStNode));
s->next=NULL;
}
销毁栈DestroyStack(&s)
void DestroyStack(LinkStNode *&s)
{ LinkStNOde *pre=s,*p=s->next; //pre指向头结点,p指向首结点
while(p!=NULL) //循环到p为空
{ free(pre); //释放pre结点
pre=p; //pre、p同步后移
p=pre->next;
}
free(pre); //此时pre指向尾结点,释放空间
}
判断栈是否为空StackEmpty(s)
bool StackEmpty(LinkStNode *s)
{ return(s->next==NULL);
}
进栈PUSH(&s,e)
bool Push(LinkStNode *&s, Elemtype e)
{ LinkStNode *p;
p=(LinkStNode *)malloc(sizeof(LinkStNode)); //新建结点p
p->data=e; //存放元素e
p->next=s->next; //p结点作为首结点插入
s->next=p;
}
出栈Pop(&s,&e)
bool Pop(LinkStNode *&s,ElemType &e)
{ LinkStNode *p;
if(s->next==NULL) //栈空情况
return false; //返回假
p=s->next; //p指向首结点
e=p->data; //取首结点值
s->next=p->next; //删除首结点
free(p); //释放被删结点的存储空间
return ture; //返回真
}
取栈顶元素GetTop(s,&e)
bool GetTop(LinkStNode *s, ElemType &e)
{ if(s->next==NULL) //栈空
return false; //返回假
e=s->next->data; //取首结点值
return true; //返回真
}
(例题)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1022
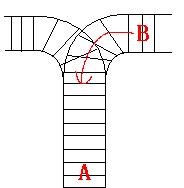

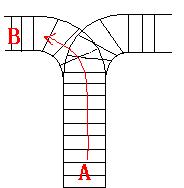
题目分析:
此题考查了栈这种数据结构的熟练使用,模拟和记录入栈和出栈的过程(先进先出)。不过需要注意的是测试数据是有多组,在处理完每组数据之后,需要保证栈为空,以备处理下一组数据。
题解代码:
#include"iostream" #include"stack" #include"string" using namespace std; int main() { int n, i, j, k; stack<char> s; string train1, train2; bool flags[2000]; while(cin>>n>>train1>>train2) { j = k = 0; for(i = 0; i < n; i++) { s.push(train1[i]);//进栈 flags[j++] = true;//记录进栈 while(!s.empty() && s.top() == train2[k])//模拟出栈 { s.pop(); flags[j++] = false;//记录出栈 k++; } } if(s.empty()) { cout<<"Yes."<<endl; //输出记录 for(i = 0; i < 2 * n; i++) { if(flags[i]) cout<<"in"<<endl; else cout<<"out"<<endl; } } else { //若栈非空,注意清空栈,以备下次使用 while(!s.empty())s.pop(); cout<<"No."<<endl; } cout<<"FINISH"<<endl; } return 0; }