http://acm.hdu.edu.cn/showproblem.php?pid=1979
Fill the blanks
Time Limit: 3000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 373 Accepted Submission(s): 155
Problem Description
There is a matrix of 4*4, you should fill it with digits 0 – 9, and you should follow the rules in the following picture:
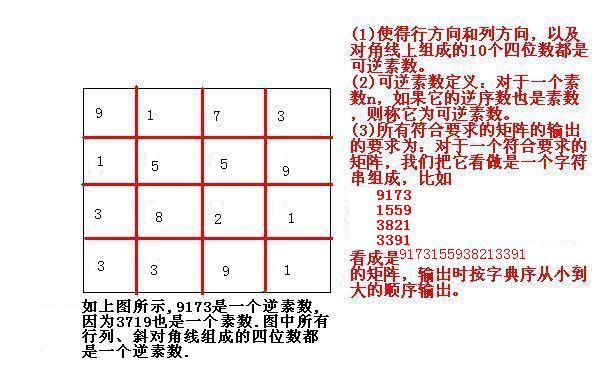
Input
No input.
Output
Print all the matrixs that fits the rules in the picture.
And there is a blank line between the every two matrixs.
And there is a blank line between the every two matrixs.
Sample Output
1193
1009
9221
3191
1193
1021
9029
3911
……
9173
1559
3821
3391
Author
8600
Source
1000 --- 9999中有204个顺着和倒着读都是素数的数。
考虑的就是暴力dfs,然后最后再判断?超时。可以打表。
可以用字典树维护前缀,
每次都维护主对角线和副对角线的数字,还有四条列。然后如果不存在这样的前缀,直接剪掉就好。

#include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <algorithm> #define IOS ios::sync_with_stdio(false) using namespace std; #define inf (0x3f3f3f3f) typedef long long int LL; #define MY "H:/CodeBlocks/project/CompareTwoFile/DataMy.txt", "w", stdout #define ANS "H:/CodeBlocks/project/CompareTwoFile/DataAns.txt", "w", stdout #include <iostream> #include <sstream> #include <vector> #include <set> #include <map> #include <queue> #include <string> const int maxn=1e5+20; bool prime[maxn];//这个用bool就够了, bool check[maxn]; int goodprime[maxn]; char strprime[204 + 2][10]; int lenprime = 0; struct node { int cnt; struct node * pNext[10]; } tree[maxn], *T; int num; struct node * create() { struct node * p = &tree[num++]; for (int i = 0; i <= 9; ++i) { p->pNext[i] = NULL; } p->cnt = 1; return p; } void insert(struct node **T, int val) { struct node *p = *T; if (p == NULL) { *T = p = create(); } char str[11] = {0}; int lenstr = 0; while (val / 10 > 0) { str[++lenstr] = val % 10 + '0'; val /= 10; } str[++lenstr] = val + '0'; str[lenstr + 1] = '