由于最近工作上有些变动,已经快一个月没有写博客了。上一篇博客[React]全自动数据表格组件——BodeGrid介绍了全自动数据表格的设计思路以及分享了一个react.js的实现。但是现实情况中为了节约开发成本,很多中小型企业是很难做到前后端完全分离的,加上目前国内使用react.js的公司可能是太少了吧,于是又折腾着写了一个JQuery版本的,结合mvc的模板页也达到了相同的效果(代码量方面),有兴趣的小伙伴也可以尝试写一写angular或者vue版本的,用起来真的能少写很多代码的。
使用到的js库:
jquery:https://jquery.com/
layer弹出层:http://layer.layui.com/
bootstrap:http://www.bootcss.com/
bootstrap-datatable:https://editor.datatables.net/examples/styling/bootstrap.html
bootstrap-datetimepicker:http://www.bootcss.com/p/bootstrap-datetimepicker/
select2:http://select2.github.io/
webupoader:http://fex-team.github.io/webuploader/
效果图-列表页:
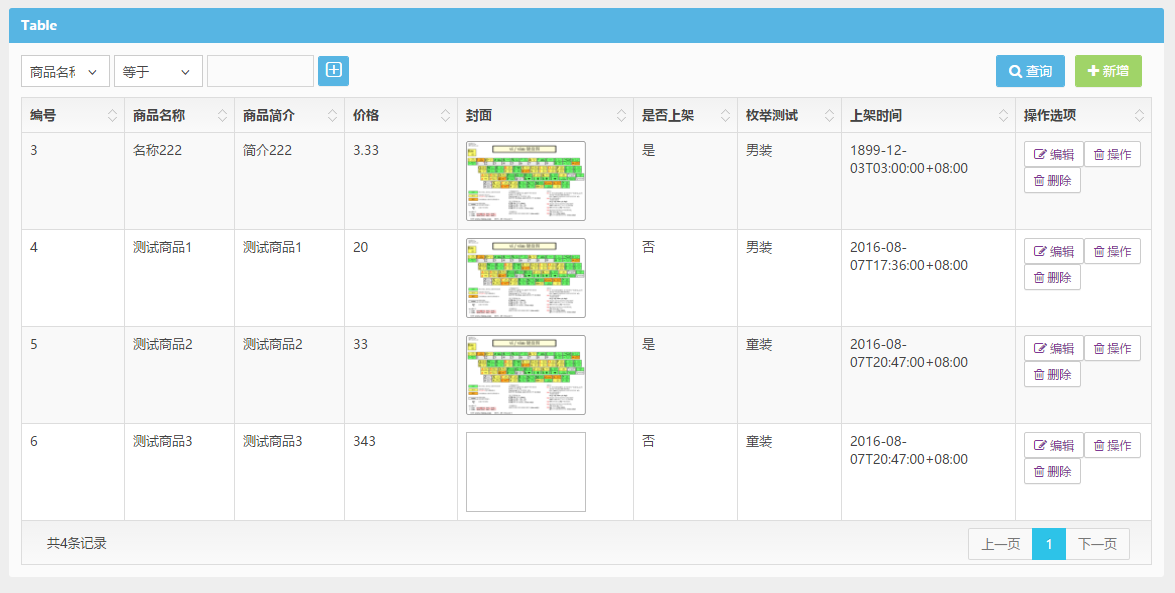
效果图-弹出框:

视图页全部代码:

@{ ViewBag.Title = "Table"; Layout = "~/Areas/Admin/Views/Shared/_GridLayout.cshtml"; } @section customScript{ <script type="text/javascript"> var enums = @Html.Raw(Json.Encode(@ViewBag.Enums)); url = { read: "/api/services/product/products/GetProductPagedList", add: "/api/services/product/products/CreateProduct", edit: "/api/services/product/products/UpdateProduct", delete: "/api/services/product/products/DeleteProduct" }; columns = [ { data: "id", title: "编号" }, { data: "name", title: "商品名称", type: "text", query: true, editor: {} }, { data: "brief", title: "商品简介", type: "textarea", query: true, editor: {} }, //{ data: "detail", title: "商品详情", type: "richtext", query: true, editor: {} }, { data: "price", title: "价格", type: "number", query: true, editor: {} }, { data: "cover2", title: "封面", type: "img", editor: {} }, { data: "isOnShelf", title: "是否上架", type: "switch", editor: {} }, { data: "enumTest", title: "枚举测试", type: "dropdown", editor: {},source:{data:enums} }, { data: "onShelfTime", title: "上架时间", type: "timepicker", editor: {} }, { title: "操作选项", type: "command", actions: [ { name: "操作", icon: "fa-trash-o", onClick: function (d) { alert(d["id"]); } } ] } ]; </script> }
服务端代码:

using System.Collections.Generic; using System.Data.Entity; using System.Linq; using System.Threading.Tasks; using Abp.Application.Services; using Abp.Application.Services.Dto; using Abp.Application.Services.Query; using Abp.AutoMapper; using Abp.Domain.Repositories; using BodeAbp.Product.Products.Dtos; namespace BodeAbp.Product.Products { /// <summary> /// 商品 应用服务 /// </summary> public class ProductsAppService : ApplicationService, IProductsAppService { private readonly IRepository<Domain.Product,long> _productRepository; /// <summary> /// 构造函数 /// </summary> /// <param name="productRepository"></param> public ProductsAppService(IRepository<Domain.Product, long> productRepository) { _productRepository = productRepository; } /// <inheritdoc/> public async Task<PagedResultOutput<GetProductListOutput>> GetProductPagedList(QueryListPagedRequestInput input) { int total; var list = await _productRepository.GetAll().Where(input, out total).ToListAsync(); return new PagedResultOutput<GetProductListOutput>(total, list.MapTo<List<GetProductListOutput>>()); } /// <inheritdoc/> public async Task CreateProduct(CreateProductInput input) { var product = input.MapTo<Domain.Product>(); await _productRepository.InsertAsync(product); } /// <inheritdoc/> public async Task UpdateProduct(UpdateProductInput input) { var product = await _productRepository.GetAsync(input.Id); input.MapTo(product); await _productRepository.UpdateAsync(product); } /// <inheritdoc/> public async Task DeleteProduct(IdInput input) { await _productRepository.DeleteAsync(input.Id); } } }
表格请求Json格式:
{ "pageIndex":1, "pageSize":15, "sortConditions":[ { "sortField":"name", "listSortDirection":1 } ], "filterGroup":{ "rules":[ { "field":"displayName", "operate":"contains", "value":"a" } ] } }
服务端响应Json格式:
{ "success": true, "result": { "totalCount": 4, "items": [ { "name": "名称222", "brief": "简介222", "detail": "<p>描述222</p>", "price": 3.33, "cover2": "xx", "isOnShelf": true, "onShelfTime": "1899-12-03T03:00:00+08:00", "enumTest": 1, "id": 3 } ] }, "error": null, "unAuthorizedRequest": false }
items中的字段应与视图页中列配置一一对应,这样很少的代码就能完全实现数据的展示、分页、新增、编辑、删除、查询、排序等功能,并且还统一了编码方式,方便质量把控和后期维护。数据表格核心文件:bode.grid.js。在BodeAbp项目中可以看到源码以及一个比较完善的demo。整个demo依然遵循前后端分离的模式,只用到了MVC的视图页作为前端展示。BodeAbp项目地址:https://github.com/liuxx001/BodeAbp。
bode.grid.js源码这里就不过多介绍了,思路和上一篇博客所说一致,只是换了一种实现方式而已。JQuery版表格与React.js版的api还是有一些出入,不过总体来说功能是增强了,具体介绍如下:
初始化方式:
var table=new $.bode.grid("#dataTable", {...});
表格api:
参数 | 类型 | 说明 | 默认值 |
url | object |
远程接口配置,示例:{read:"",add:"",edit:"",delete:""} 其中add、edit、delete属于功能项,不配置url相关功能不会显示 |
|
columns | array[object] | 列配置,下文会详细介绍 | |
actions | array[object] |
右上角操作按钮,默认添加"搜索"; 如果url配置了add,则添加"新增"选项 |
|
imgSaveUrl | string | img类型图片上传地址 | "/api/File/UploadPic" |
formId | string | 弹出框id | "bode-grid-autoform" |
formWidth | string | 弹出框宽度,支持px与百分数 |
无富文本编辑器时:40%; 有富文本编辑器时:60% |
pageIndex | number | 页序号 | 1 |
pageSize | number | 每页数量 | 15 |
beforeInit | function | 初始化前执行 | |
initComplete | function | 初始化后执行 | |
loadDataComplete | function | 每次数据重新加载后执行 |
column属性详细介绍:
title | string | 表头显示标题 | |
data | string | 从数据源获取对应的字段名 | |
type | string |
该列的类型,现支持的类型有: text、textarea、richtext、number、switch、dropdown、 img、datepicker、timepicker、hide、command |
|
query | boolean | 是否允许查询 | false |
editor | object | 编辑相关配置,还可以继续完善 | |
source | object |
dropdown类型下拉数据源,格式: {data:[{value:"xx",text:"vv"}],textField:"xx",valueField:"xx" } |
textField默认"text"; valueField默认"value" |
sortDisable | boolean | 是否禁止排序 | false |
render | function(v,d) | 自定义列渲染事件,v表示当前单元格的数据,d表示当前行的数据 | |
editor | object |
{ap:"hide",ep:"disabled"};ap:新增模式,ep:编辑模式;'hide'表示 隐藏该列,'disabled'表示该列不可编辑 |
目前整个bode.grid.js还有很多需要完善地方,我会在以后的使用过程中继续完善修改,本次分享仅供交流,如要使用,要做好填坑的准备。其实整个源码还是比较简单的,只有600行代码,有兴趣的小伙伴可以看看,欢迎交流。