iOS开发UI篇—CAlayer(自定义layer)
一、第一种方式
1.简单说明
以前想要在view中画东西,需要自定义view,创建一个类与之关联,让这个类继承自UIView,然后重写它的DrawRect:方法,然后在该方法中画图。
绘制图形的步骤:
(1)获取上下文
(2)绘制图形
(3)渲染图形
如果在layer上画东西,与上面的过程类似。
代码示例:
新建一个类,让该类继承自CALayer
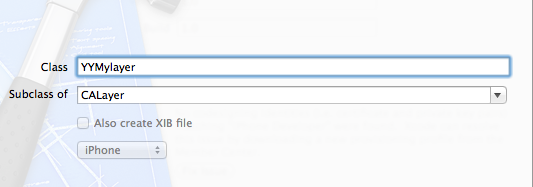
YYMylayer.m文件
1 // 2 // YYMylayer.m 3 // 05-自定义layer(1) 4 // 5 // Created by apple on 14-6-21. 6 // Copyright (c) 2014年 itcase. All rights reserved. 7 // 8 9 #import "YYMylayer.h" 10 11 @implementation YYMylayer 12 //重写该方法,在该方法内绘制图形 13 -(void)drawInContext:(CGContextRef)ctx 14 { 15 //1.绘制图形 16 //画一个圆 17 CGContextAddEllipseInRect(ctx, CGRectMake(50, 50, 100, 100)); 18 //设置属性(颜色) 19 // [[UIColor yellowColor]set]; 20 CGContextSetRGBFillColor(ctx, 0, 0, 1, 1); 21 22 //2.渲染 23 CGContextFillPath(ctx); 24 } 25 @end
在控制器中,创建一个自定义的类
1 // 2 // YYViewController.m 3 // 05-自定义layer(1) 4 // 5 // Created by apple on 14-6-21. 6 // Copyright (c) 2014年 itcase. All rights reserved. 7 // 8 9 #import "YYViewController.h" 10 #import "YYMylayer.h" 11 12 @interface YYViewController () 13 14 @end 15 16 @implementation YYViewController 17 18 - (void)viewDidLoad 19 { 20 [super viewDidLoad]; 21 22 //1.创建自定义的layer 23 YYMylayer *layer=[YYMylayer layer]; 24 //2.设置layer的属性 25 layer.backgroundColor=[UIColor brownColor].CGColor; 26 layer.bounds=CGRectMake(0, 0, 200, 150); 27 layer.anchorPoint=CGPointZero; 28 layer.position=CGPointMake(100, 100); 29 layer.cornerRadius=20; 30 layer.shadowColor=[UIColor blackColor].CGColor; 31 layer.shadowOffset=CGSizeMake(10, 20); 32 layer.shadowOpacity=0.6; 33 34 [layer setNeedsDisplay]; 35 //3.添加layer 36 [self.view.layer addSublayer:layer]; 37 38 } 39 40 @end
注意点:
(1)默认为无色,不会显示。要想让绘制的图形显示出来,还需要设置图形的颜色。注意不能直接使用UI框架中的类
(2)在自定义layer中的-(void)drawInContext:方法不会自己调用,只能自己通过setNeedDisplay方法调用,在view中画东西DrawRect:方法在view第一次显示的时候会自动调用。
实现效果:
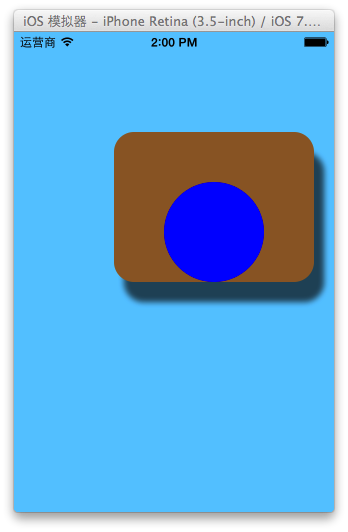
2.拓展
UIView中绘图说明
1 #import "YYVIEW.h" 2 3 @implementation YYVIEW 4 5 6 - (void)drawRect:(CGRect)rect 7 { 8 //1.获取上下文 9 CGContextRef ctx=UIGraphicsGetCurrentContext(); 10 //2.绘制图形 11 CGContextAddEllipseInRect(ctx, CGRectMake(50, 50, 100, 100)); 12 //设置属性(颜色) 13 // [[UIColor yellowColor]set]; 14 CGContextSetRGBFillColor(ctx, 0, 0, 1, 1); 15 16 //3.渲染 17 CGContextFillPath(ctx); 18 //在执行渲染操作的时候,本质上它的内部相当于调用了下面的方法 19 [self.layer drawInContext:ctx]; 20 }
说明:在UIView中绘制图形,获取的上下文就是这个view对应的layer的上下文。在渲染的时候,就是把图形渲染到对应的layer上。
在执行渲染操作的时候,本质上它的内部相当于执行了 [self.layer drawInContext:ctx];
二、第二种方式
方法描述:设置CALayer的delegate,然后让delegate实现drawLayer:inContext:方法,当CALayer需要绘图时,会调用delegate的drawLayer:inContext:方法进行绘图。
代码示例:
1 // 2 // YYViewController.m 3 // 06-自定义layer(2) 4 // 5 // Created by apple on 14-6-21. 6 // Copyright (c) 2014年 itcase. All rights reserved. 7 8 #import "YYViewController.h" 9 @interface YYViewController () 10 @end 11 12 @implementation YYViewController 13 14 - (void)viewDidLoad 15 { 16 [super viewDidLoad]; 17 //1.创建自定义的layer 18 CALayer *layer=[CALayer layer]; 19 //2.设置layer的属性 20 layer.backgroundColor=[UIColor brownColor].CGColor; 21 layer.bounds=CGRectMake(0, 0, 200, 150); 22 layer.anchorPoint=CGPointZero; 23 layer.position=CGPointMake(100, 100); 24 layer.cornerRadius=20; 25 layer.shadowColor=[UIColor blackColor].CGColor; 26 layer.shadowOffset=CGSizeMake(10, 20); 27 layer.shadowOpacity=0.6; 28 29 //设置代理 30 layer.delegate=self; 31 [layer setNeedsDisplay]; 32 //3.添加layer 33 [self.view.layer addSublayer:layer]; 34 } 35 36 -(void)drawLayer:(CALayer *)layer inContext:(CGContextRef)ctx 37 { 38 //1.绘制图形 39 //画一个圆 40 CGContextAddEllipseInRect(ctx, CGRectMake(50, 50, 100, 100)); 41 //设置属性(颜色) 42 // [[UIColor yellowColor]set]; 43 CGContextSetRGBFillColor(ctx, 0, 0, 1, 1); 44 45 //2.渲染 46 CGContextFillPath(ctx); 47 } 48 @end
实现效果:
注意点:不能再将某个UIView设置为CALayer的delegate,因为UIView对象已经是它内部根层的delegate,再次设置为其他层的delegate就会出问题。
在设置代理的时候,它并不要求我们遵守协议,说明这个方法是nsobject中的,就不需要再额外的显示遵守协议了。
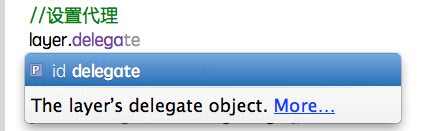
提示:以后如果要设置某个类的代理,但是这个代理没要求我们遵守什么特定的协议,那么可以认为这个协议方法是NSObject里边的。
三、补充说明
(1)无论采取哪种方法来自定义层,都必须调用CALayer的setNeedsDisplay方法才能正常绘图。
(2)详细现实过程:
当UIView需要显示时,它内部的层会准备好一个CGContextRef(图形上下文),然后调用delegate(这里就是UIView)的drawLayer:inContext:方法,并且传入已经准备好的CGContextRef对象。而UIView在drawLayer:inContext:方法中又会调用自己的drawRect:方法。平时在drawRect:中通过UIGraphicsGetCurrentContext()获取的就是由层传入的CGContextRef对象,在drawRect:中完成的所有绘图都会填入层的CGContextRef中,然后被拷贝至屏幕。