题目
判断二叉树是否为搜素二叉树
java代码
package com.lizhouwei.chapter3;
/**
* @Description:判断二叉树是否为搜素二叉树
* @Author: lizhouwei
* @CreateDate: 2018/4/14 23:10
* @Modify by:
* @ModifyDate:
*/
public class Chapter3_13 {
public boolean isBalance(Node head) {
if (head == null) {
return false;
}
boolean[] record = new boolean[1];
record[0] = true;
getHight(head, 1, record);
return record[0];
}
public int getHight(Node head, int level, boolean[] record) {
if (head == null) {
return level;
}
int leftDep = getHight(head.left, level + 1, record);
if (!record[0]) {
return level;
}
int rightDep = getHight(head.right, level + 1, record);
if (!record[0]) {
return level;
}
if (Math.abs(rightDep - leftDep) > 1) {
record[0] = false;
}
return Math.max(leftDep, rightDep);
}
//测试
public static void main(String[] args) {
Chapter3_13 chapter = new Chapter3_13();
Node head = new Node(1);
head.left = new Node(2);
head.right = new Node(3);
head.left.left = new Node(4);
head.left.right = new Node(5);
head.left.left.left = new Node(8);
head.left.left.right = new Node(9);
head.left.right.left = new Node(10);
System.out.print("head是否为平衡树 :" + chapter.isBalance(head));
System.out.println();
Node head2 = new Node(1);
head2.left = new Node(2);
head2.right = new Node(3);
head2.left.left = new Node(4);
head2.left.right = new Node(5);
head2.right.left = new Node(6);
head2.right.right = new Node(7);
System.out.print("head2是否为平衡树 :" + chapter.isBalance(head2));
}
}
结果
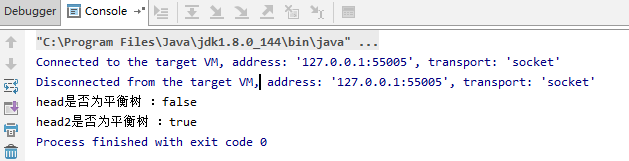