原型模式:用原型实例指定创建对象的种类,并且通过复制这些原型创建新的对象
原型模式其实就是一个从一个对象再创建另一个可定制的对象,而且不需要知道任何创建细节,NET在System命名空间下,提供了ICloneable接口,实现这个接口完成原型模式。一般在初始化信息不变的时候,克隆是最好的办法。隐藏了创建对象的细节,性能也大大提高。MemberWiseClone(),复制仅仅对线程栈进行复制,是属于浅复制,要深复制,需要稍微修改代码。
一般人的简历是不变,需要多份,这个时候使用克隆模式比较好:
简历类:
class Resume : ICloneable
{
private string name;
private string sex;
private string age;
private WorkExperience work;
public Resume(string name)
{
this.name = name;
this.work = new WorkExperience();
}
public Resume(WorkExperience work)
{
this.work = (WorkExperience)work.Clone();
}
public void SerPersonalInfo(string sex, string age)
{
this.sex = sex;
this.age = age;
}
public void SerWorkExperience(string timeArea,string company)
{
this.work.WorkDate = timeArea;
this.work.Company = company;
}
public void Display()
{
Console.WriteLine("{0}--{1}--{2}",name,sex,age);
Console.WriteLine("工作经验:{0}--{1}", work.WorkDate, work.Company);
}
//深复制
public object Clone()
{
Resume obj = new Resume(this.work);
obj.name = this.name;
obj.sex = this.sex;
obj.age = this.age;
return obj;
}
//浅复制
//public object Clone()
//{
// return (object)this.MemberwiseClone();
//}
}
{
private string name;
private string sex;
private string age;
private WorkExperience work;
public Resume(string name)
{
this.name = name;
this.work = new WorkExperience();
}
public Resume(WorkExperience work)
{
this.work = (WorkExperience)work.Clone();
}
public void SerPersonalInfo(string sex, string age)
{
this.sex = sex;
this.age = age;
}
public void SerWorkExperience(string timeArea,string company)
{
this.work.WorkDate = timeArea;
this.work.Company = company;
}
public void Display()
{
Console.WriteLine("{0}--{1}--{2}",name,sex,age);
Console.WriteLine("工作经验:{0}--{1}", work.WorkDate, work.Company);
}
//深复制
public object Clone()
{
Resume obj = new Resume(this.work);
obj.name = this.name;
obj.sex = this.sex;
obj.age = this.age;
return obj;
}
//浅复制
//public object Clone()
//{
// return (object)this.MemberwiseClone();
//}
}
工作经验类:
class WorkExperience : ICloneable
{
public string WorkDate { get; set; }
public string Company { get; set; }
public Object Clone()
{
return (Object)this.MemberwiseClone();
}
}
{
public string WorkDate { get; set; }
public string Company { get; set; }
public Object Clone()
{
return (Object)this.MemberwiseClone();
}
}
主函数调用:
class Program
{
static void Main(string[] args)
{
Resume a = new Resume("刘明");
a.SerPersonalInfo("男","24");
a.SerWorkExperience("1998-2000","XXX公司");
Resume b = (Resume)a.Clone();
b.SerWorkExperience("1998-2000", "yyy公司");
Resume c = (Resume)a.Clone();
c.SerWorkExperience("1998-2000", "fff公司");
c.SerPersonalInfo("女","小丽");
a.Display();
b.Display();
c.Display();
Console.ReadKey();
}
}
{
static void Main(string[] args)
{
Resume a = new Resume("刘明");
a.SerPersonalInfo("男","24");
a.SerWorkExperience("1998-2000","XXX公司");
Resume b = (Resume)a.Clone();
b.SerWorkExperience("1998-2000", "yyy公司");
Resume c = (Resume)a.Clone();
c.SerWorkExperience("1998-2000", "fff公司");
c.SerPersonalInfo("女","小丽");
a.Display();
b.Display();
c.Display();
Console.ReadKey();
}
}
看一张原型模式结构图:
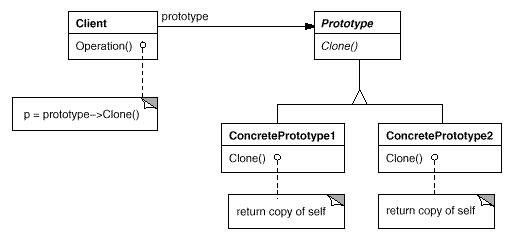