别人已经写过很好的XML辅助类,可以直接引用后使用;
我这里自己写一个xml的操作类,目前能实现的是对一个不含集合的类可以操作,含集合的类无法将集合里的数据读取出来,
首先定义一个XML特性,用于区分属性和标签
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Text; 5 using System.Threading.Tasks; 6 /********************************************************************* 7 唯一标识:098b09ce-0d0e-4a87-ba21-2adf20d951b5 8 版本号:v1.0.0.0 9 CLR版本:4.0.30319.42000 10 ====================================================================== 11 创建人: liusg 12 创建时间:2018/7/6 11:10:25 13 **********************************************************************/ 14 namespace CrtXElement 15 { 16 /// <summary> 17 /// XML特性 18 /// </summary> 19 public class CrtXmlAttribute: Attribute 20 { 21 /// <summary> 22 /// 标记为属性 23 /// </summary> 24 public bool Property { get; set; } 25 /// <summary> 26 /// 标记为唯一标识键 27 /// </summary> 28 public bool Key { get; set; } 29 30 } 31 }
写一个测试用的对象类(类里面不能含集合)
1 using System; 2 using System.Collections.Generic; 3 using System.Linq; 4 using System.Text; 5 using System.Threading.Tasks; 6 /********************************************************************* 7 唯一标识:63818db9-6d98-45b9-8c3f-0545f8f4f9b3 8 版本号:v1.0.0.0 9 CLR版本:4.0.30319.42000 10 ====================================================================== 11 创建人: liusg 12 创建时间:2018/7/6 11:08:44 13 **********************************************************************/ 14 namespace CrtXElement 15 { 16 public class CrtModel 17 { 18 [CrtXml(Key=true,Property =true)] 19 public int Key { get; set; } 20 public string Name { get; set; } 21 } 22 23 }
然后是实现对XML的操作方式,
1 using CrtGloble; 2 using System; 3 using System.Collections.Generic; 4 using System.IO; 5 using System.Linq; 6 using System.Text; 7 using System.Threading; 8 using System.Threading.Tasks; 9 using System.Xml; 10 using System.Xml.Linq; 11 /********************************************************************* 12 唯一标识:d9c52e14-e9d1-4152-9ded-25b25383247e 13 版本号:v1.0.0.0 14 CLR版本:4.0.30319.42000 15 ====================================================================== 16 创建人: liusg 17 创建时间:2018/7/6 13:19:05 18 **********************************************************************/ 19 namespace CrtXElement 20 { 21 public class CxeDemo<T> where T : new() 22 { 23 public string _FilePath = "/"; 24 private XElement root; 25 public void Load(string filePath, string fileName) 26 { 27 this._FilePath = Path.Combine(filePath, fileName); 28 if (string.IsNullOrEmpty(filePath)) 29 throw new Exception("无效的路径"); 30 31 if (string.IsNullOrEmpty(fileName) || !fileName.Contains('.')) 32 throw new Exception("无效的文件名"); 33 34 if (!Directory.Exists(filePath)) 35 Directory.CreateDirectory(filePath); 36 37 try 38 { 39 40 if (!File.Exists(Path.Combine(filePath, fileName))) 41 root = new XElement($"XML"); 42 else root = XElement.Load(Path.Combine(filePath, fileName)); 43 } 44 catch (Exception e) 45 { 46 47 root = new XElement($"XML"); 48 } 49 if (root == null) 50 { 51 root = new XElement($"XML"); 52 } 53 54 55 } 56 private bool Save() 57 { 58 if (root == null) 59 return false; 60 61 62 try 63 { 64 root.Save(_FilePath); 65 } 66 catch (Exception e) 67 { 68 Console.WriteLine(e.Message); 69 return false; 70 } 71 return true; 72 } 73 74 public List<T> GetList() 75 { 76 List<T> list = new List<T>(); 77 var xmlList = root.Elements(); 78 var _type = typeof(T); 79 foreach (XElement item in xmlList) 80 { 81 var model = new T(); 82 foreach (var Propertie in _type.GetProperties()) 83 { 84 if (Propertie.IsDefined(typeof(CrtXmlAttribute), true)) 85 { 86 var Attributes = (CrtXmlAttribute)Propertie.GetCustomAttributes(false).FirstOrDefault(); 87 if (Attributes == null) 88 { 89 var attrValue = item.Element(Propertie.Name); 90 if (attrValue != null) 91 Propertie.SetValue(model, GetTypeVal(attrValue.Value, Propertie.PropertyType)); 92 else 93 Propertie.SetValue(model, GetTypeVal("", Propertie.PropertyType)); 94 } 95 else 96 { 97 if (Attributes.Property) 98 { 99 var attrValue = item.Attribute(Propertie.Name); 100 if (attrValue != null) 101 Propertie.SetValue(model, GetTypeVal(attrValue.Value, Propertie.PropertyType)); 102 else 103 Propertie.SetValue(model, GetTypeVal("", Propertie.PropertyType)); 104 } 105 else 106 { 107 var attrValue = item.Element(Propertie.Name); 108 if (attrValue != null) 109 Propertie.SetValue(model, GetTypeVal(attrValue.Value, Propertie.PropertyType)); 110 else 111 Propertie.SetValue(model, GetTypeVal("", Propertie.PropertyType)); 112 } 113 } 114 } 115 else 116 { 117 var attrValue = item.Element(Propertie.Name); 118 if (attrValue != null) 119 Propertie.SetValue(model, GetTypeVal(attrValue.Value, Propertie.PropertyType)); 120 else 121 Propertie.SetValue(model, GetTypeVal("", Propertie.PropertyType)); 122 } 123 } 124 list.Add(model); 125 } 126 return list; 127 } 128 public void Add(T model) 129 { 130 var _type = typeof(T); 131 XElement newel = new XElement(_type.Name); 132 foreach (var item in _type.GetProperties()) 133 { 134 if (item.IsDefined(typeof(CrtXmlAttribute), true)) 135 { 136 var Attributes = (CrtXmlAttribute)item.GetCustomAttributes(false).FirstOrDefault(); 137 if (Attributes == null) 138 { 139 XElement net = new XElement(item.Name); 140 net.SetValue(item.GetValue(model)); 141 newel.Add(net); 142 } 143 else 144 { 145 if (Attributes.Property) 146 { 147 newel.SetAttributeValue(item.Name, item.GetValue(model)); 148 149 } 150 else 151 { 152 XElement net = new XElement(item.Name); 153 net.SetValue(item.GetValue(model)); 154 newel.Add(net); 155 } 156 } 157 } 158 else 159 { 160 XElement net = new XElement(item.Name); 161 net.SetValue(item.GetValue(model)); 162 newel.Add(net); 163 } 164 } 165 root.Add(newel); 166 167 Save(); 168 169 } 170 171 public void Update(T model) 172 { 173 if(Delete(model)) 174 { 175 Add(model); 176 } 177 } 178 public bool Delete(Func<T,bool> where) 179 { 180 var model = GetList().Where(where).FirstOrDefault(); 181 if (model == null) 182 return false; 183 return Delete(model); 184 } 185 public bool Delete(T model) 186 { 187 var _type = typeof(T); 188 foreach (var item in _type.GetProperties()) 189 { 190 var Attributes = (CrtXmlAttribute)item.GetCustomAttributes(false).FirstOrDefault(); 191 if (Attributes != null) 192 { 193 if (Attributes.Key) 194 { 195 var rootFist = root.Elements(_type.Name).FirstOrDefault(p => p.Attribute(item.Name).Value == item.GetValue(model).ToString()); 196 if (rootFist != null) 197 { 198 root.Elements(_type.Name).Where(p => p == rootFist).Remove(); 199 return true; 200 201 } 202 } 203 } 204 } 205 return false; 206 } 207 208 object GetTypeVal(object obj, Type ty) 209 { 210 var val = ty.Name; 211 if (val == "Int32") 212 { 213 return obj.ToInt_V(); 214 } 215 else if (val == "String") 216 { 217 return obj.ToString_V(); 218 } 219 else if (val == "DateTime") 220 { 221 return obj.ToDateTime_V(); 222 } 223 else if (val == "Double") 224 { 225 return obj.ToDouble_V(); 226 } 227 else if (val == "Boolean") 228 { 229 return obj.ToBoolean_V(); 230 } 231 else if (val == "Decimal") 232 { 233 return obj.ToDecimal_V(); 234 } 235 else if (val == "Int64") 236 { 237 return obj.ToLong_V(); 238 } 239 else if (val == "UInt32") 240 { 241 return obj.ToUint_V(); 242 } 243 else 244 { 245 return obj.ToString_V(); 246 } 247 } 248 } 249 }
接下来就是测试数据
1 using CrtGloble; 2 using System; 3 using System.Collections.Generic; 4 using System.Linq; 5 using System.Text; 6 using System.Threading.Tasks; 7 8 namespace CrtXElement 9 { 10 class Program 11 { 12 static void Main(string[] args) 13 { 14 CxeDemo<CrtModel> demo = new CxeDemo<CrtModel>(); 15 demo.Load(@"F:", "demo.txt"); 16 //demo.Add(new CrtModel 17 //{ 18 // Key = 23, 19 // Name = "ddd" 20 //}); 21 //demo.Add(new CrtModel 22 //{ 23 // Key = 24, 24 // Name = "ddd" 25 //}); 26 //demo.Update(new CrtModel 27 //{ 28 // Key = 24, 29 // Name = "sdfgserg", 30 //}); 31 //demo.Update(new CrtModel 32 //{ 33 // Key = 240, 34 // Name = "sdfgserg", 35 //}); 36 foreach (var item in demo.GetList()) 37 { 38 Console.WriteLine(item.ToProperties_V()); 39 } 40 41 42 43 } 44 } 45 }
xml文件
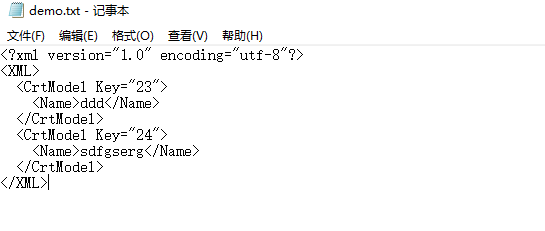