1720:
(计算两个16进制的数的10进制形式的和)
Input may contain multiple test cases. Each case contains A and B in one line.
A, B are hexadecimal number.
Input terminates by EOF.
Output
Output A+B in decimal number in one line.
Sample Input
1 9
A B
a b
Sample Output
10
21
21

#include<stdio.h> int main() { int x, y; while (scanf("%x%x", &x, &y) != EOF)//以十六进制输入,o为八进制,没有二进制输入法。 { printf("%d ", x + y);//十进制输出 } return 0; }
心得: 可通过“%x”控制输入是十六进制的数,输出就照常%d“”,十进制输出就行
1062:
(把输入的每一个词的字母反过来输出)
Ignatius likes to write words in reverse way. Given a single line of text which is written by Ignatius, you should reverse all the words and then output them.
Input
The input contains several test cases. The first line of the input is a single integer T which is the number of test cases. T test cases follow.
Each test case contains a single line with several words. There will be at most 1000 characters in a line.
Each test case contains a single line with several words. There will be at most 1000 characters in a line.
Output
For each test case, you should output the text which is processed.
Sample Input
3
olleh !dlrow
m'I morf .udh
I ekil .mca
Sample Output
hello world!
I'm from hdu.
I like acm.

#include<stdio.h> #include<string.h> int main() { int n, i, j, flag; char str[1005]; scanf("%d", &n); getchar(); while (n--) { gets(str); flag = 0; int l = strlen(str); for (i = 0; i < l; i++) { if (str[i] == ' ') { for (j = i - 1; j >= flag; j--) //不用真的翻转,倒序输出就行,当然string使用reverse函数也行 { printf("%c", str[j]); } printf(" "); flag = i + 1; } } for (j = l - 1; j >= flag; j--) { printf("%c", str[j]); } printf(" "); } return 0; }
心得:数组字符串的使用
2104:
(总个数为n,每次跳过m-1个位置,问从1开始跳能不能在有限次数后找到n)
Problem Description
The Children’s Day has passed for some days .Has you remembered something happened at your childhood? I remembered I often played a game called hide handkerchief with my friends.
Now I introduce the game to you. Suppose there are N people played the game ,who sit on the ground forming a circle ,everyone owns a box behind them .Also there is a beautiful handkerchief hid in a box which is one of the boxes .
Then Haha(a friend of mine) is called to find the handkerchief. But he has a strange habit. Each time he will search the next box which is separated by M-1 boxes from the current box. For example, there are three boxes named A,B,C, and now Haha is at place of A. now he decide the M if equal to 2, so he will search A first, then he will search the C box, for C is separated by 2-1 = 1 box B from the current box A . Then he will search the box B ,then he will search the box A.
So after three times he establishes that he can find the beautiful handkerchief. Now I will give you N and M, can you tell me that Haha is able to find the handkerchief or not. If he can, you should tell me "YES", else tell me "POOR Haha".
Now I introduce the game to you. Suppose there are N people played the game ,who sit on the ground forming a circle ,everyone owns a box behind them .Also there is a beautiful handkerchief hid in a box which is one of the boxes .
Then Haha(a friend of mine) is called to find the handkerchief. But he has a strange habit. Each time he will search the next box which is separated by M-1 boxes from the current box. For example, there are three boxes named A,B,C, and now Haha is at place of A. now he decide the M if equal to 2, so he will search A first, then he will search the C box, for C is separated by 2-1 = 1 box B from the current box A . Then he will search the box B ,then he will search the box A.
So after three times he establishes that he can find the beautiful handkerchief. Now I will give you N and M, can you tell me that Haha is able to find the handkerchief or not. If he can, you should tell me "YES", else tell me "POOR Haha".
Input
There will be several test cases; each case input contains two integers N and M, which satisfy the relationship: 1<=M<=100000000 and 3<=N<=100000000. When N=-1 and M=-1 means the end of input case, and you should not process the data.
Output
For each input case, you should only the result that Haha can find the handkerchief or not.
Sample Input
3 2
-1 -1
Sample Output
YES
(1)凭借我查找的资料和多年玩丢手绢的经验,丢手绢是跑一圈找不到就算失败,我对这个题的理解是只能跑一圈也就是最多只能找n/m次数,所以有如下代码,:

//by lmc in 2020年6月6日15:14:49 #include<stdio.h> int main() { int n,m,i; while (scanf("%d%d", &n, &m) != EOF&&n!= -1 ,m != -1) { if (n<m+1) printf("no "); else for (i = 0; i < n / m; i++) { if (n = 1 + (m - 1) * i) printf("yes "); else continue; } } }
(2)但是大家的答案是理解为可以无限跑圈找到为止,使用了辗转相除法,具体步骤如图:
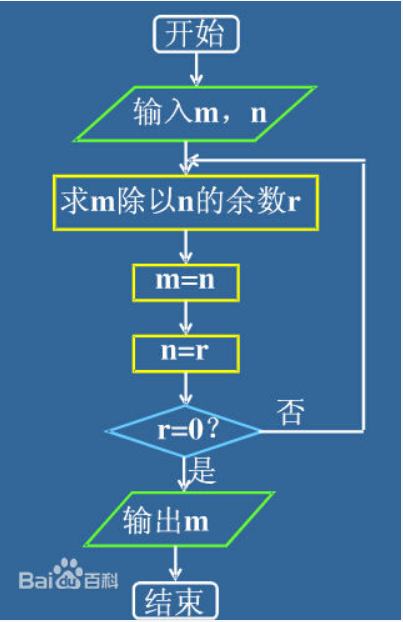

#include<stdio.h> int main() { int n, m, r; while (scanf("%d%d", &n, &m) != EOF && n != -1, m != -1) { r = 1; while (r != 0) { r = m % n; m = n; n = r; } if (m == 1) printf("yes "); else printf("no "); } return 0; }
1064:
(算数求平均值)
Problem Description
Larry graduated this year and finally has a job. He’s making a lot of money, but somehow never seems to have enough. Larry has decided that he needs to grab hold of his financial portfolio and solve his financing problems. The first step is to figure out what’s been going on with his money. Larry has his bank account statements and wants to see how much money he has. Help Larry by writing a program to take his closing balance from each of the past twelve months and calculate his average account balance.
Input
The input will be twelve lines. Each line will contain the closing balance of his bank account for a particular month. Each number will be positive and displayed to the penny. No dollar sign will be included.
Output
The output will be a single number, the average (mean) of the closing balances for the twelve months. It will be rounded to the nearest penny, preceded immediately by a dollar sign, and followed by the end-of-line. There will be no other spaces or characters in the output.
Sample Input
100.00 489.12 12454.12 1234.10 823.05 109.20 5.27 1542.25 839.18 83.99 1295.01 1.75
Sample Output
$1581.42

//by lmc in 2020年6月6日16:30:22 #include<stdio.h> int main() { int i; float sum=0, n, m; for (i = 1; i <=12; i++) { scanf("%f", &n); sum += n; getchar(); } printf("$%.2f",sum/12); }
2734:
(把大写字母用数字代替,根据位置进行乘积作和)
Problem Description
A checksum is an algorithm that scans a packet of data and returns a single number. The idea is that if the packet is changed, the checksum will also change, so checksums are often used for detecting transmission errors, validating document contents, and in many other situations where it is necessary to detect undesirable changes in data.
For this problem, you will implement a checksum algorithm called Quicksum. A Quicksum packet allows only uppercase letters and spaces. It always begins and ends with an uppercase letter. Otherwise, spaces and letters can occur in any combination, including consecutive spaces.
A Quicksum is the sum of the products of each character's position in the packet times the character's value. A space has a value of zero, while letters have a value equal to their position in the alphabet. So, A=1, B=2, etc., through Z=26. Here are example Quicksum calculations for the packets "ACM" and "MID CENTRAL":
ACM: 1*1 + 2*3 + 3*13 = 46MID CENTRAL: 1*13 + 2*9 + 3*4 + 4*0 + 5*3 + 6*5 + 7*14 + 8*20 + 9*18 + 10*1 + 11*12 = 650
For this problem, you will implement a checksum algorithm called Quicksum. A Quicksum packet allows only uppercase letters and spaces. It always begins and ends with an uppercase letter. Otherwise, spaces and letters can occur in any combination, including consecutive spaces.
A Quicksum is the sum of the products of each character's position in the packet times the character's value. A space has a value of zero, while letters have a value equal to their position in the alphabet. So, A=1, B=2, etc., through Z=26. Here are example Quicksum calculations for the packets "ACM" and "MID CENTRAL":
ACM: 1*1 + 2*3 + 3*13 = 46MID CENTRAL: 1*13 + 2*9 + 3*4 + 4*0 + 5*3 + 6*5 + 7*14 + 8*20 + 9*18 + 10*1 + 11*12 = 650
Input
The input consists of one or more packets followed by a line containing only # that signals the end of the input. Each packet is on a line by itself, does not begin or end with a space, and contains from 1 to 255 characters.
Output
For each packet, output its Quicksum on a separate line in the output.
Sample Input
ACM
MID CENTRAL
REGIONAL PROGRAMMING CONTEST
ACN
A C M
ABC
BBC
#
Sample Output
46
650
4690
49
75
14
15

#include <string.h> #include <stdio.h> int main() { char str[10000]; int len, i, a[26], sum; for (i = 0; i < 26; i++) { a[i] = i + 1; } while (gets(str)) { if (strcmp(str, "#") == 0) break; len = strlen(str); sum = 0; for (i = 0; i < len; i++) { if (str[i] >= 'A' && str[i] <= 'Z') sum += (i + 1) * a[str[i] - 'A']; } printf("%d ", sum); } return 0; }
1170:
(四则运算)
Problem Description
The contest starts now! How excited it is to see balloons floating around. You, one of the best programmers in HDU, can get a very beautiful balloon if only you have solved the very very very... easy problem.
Give you an operator (+,-,*, / --denoting addition, subtraction, multiplication, division respectively) and two positive integers, your task is to output the result.
Is it very easy?
Come on, guy! PLMM will send you a beautiful Balloon right now!
Good Luck!
Give you an operator (+,-,*, / --denoting addition, subtraction, multiplication, division respectively) and two positive integers, your task is to output the result.
Is it very easy?
Come on, guy! PLMM will send you a beautiful Balloon right now!
Good Luck!
Input
Input contains multiple test cases. The first line of the input is a single integer T (0<T<1000) which is the number of test cases. T test cases follow. Each test case contains a char C (+,-,*, /) and two integers A and B(0<A,B<10000).Of course, we all know that A and B are operands and C is an operator.
Output
For each case, print the operation result. The result should be rounded to 2 decimal places If and only if it is not an integer.
Sample Input
4
+ 1 2
- 1 2
* 1 2
/ 1 2
Sample Output
3
-1
2
0.50

#include <stdio.h> int main() { char c; int n,a, b; scanf("%d",&n); while (n--) { getchar(); //标准输入其实是先放在一个缓冲区,getchar()会保留缓冲区中的换行符,如果下面还需要输入,那么这个换行符可能就会影响下面的输入,所以要消除干扰; scanf("%c%d%d", &c, &a, &b); if (c == '+') { printf("%d ", a + b); } else if (c == '-') { printf("%d ", a - b); } else if (c == '*') { printf("%d ", a * b); } else { if (a % b == 0) printf("%d ", a / b);//这里注意,看清题目意思 else printf("%.2f ", (float)a / (float)b); } } return 0; }
1197:
Problem Description
Find and list all four-digit numbers in decimal notation that have the property that the sum of its four digits equals the sum of its digits when represented in hexadecimal (base 16) notation and also equals the sum of its digits when represented in duodecimal (base 12) notation.
For example, the number 2991 has the sum of (decimal) digits 2+9+9+1 = 21. Since 2991 = 1*1728 + 8*144 + 9*12 + 3, its duodecimal representation is 1893(12), and these digits also sum up to 21. But in hexadecimal 2991 is BAF16, and 11+10+15 = 36, so 2991 should be rejected by your program.
The next number (2992), however, has digits that sum to 22 in all three representations (including BB016), so 2992 should be on the listed output. (We don't want decimal numbers with fewer than four digits - excluding leading zeroes - so that 2992 is the first correct answer.)
For example, the number 2991 has the sum of (decimal) digits 2+9+9+1 = 21. Since 2991 = 1*1728 + 8*144 + 9*12 + 3, its duodecimal representation is 1893(12), and these digits also sum up to 21. But in hexadecimal 2991 is BAF16, and 11+10+15 = 36, so 2991 should be rejected by your program.
The next number (2992), however, has digits that sum to 22 in all three representations (including BB016), so 2992 should be on the listed output. (We don't want decimal numbers with fewer than four digits - excluding leading zeroes - so that 2992 is the first correct answer.)
Input
There is no input for this problem.
Output
Your output is to be 2992 and all larger four-digit numbers that satisfy the requirements (in strictly increasing order), each on a separate line with no leading or trailing blanks, ending with a new-line character. There are to be no blank lines in the output. The first few lines of the output are shown below.
Sample Input
There is no input for this problem.
Sample Output
2992
2993
2994
2995
2996
2997
2998
2999

#include<string.h> #include<stdio.h> int f(int n, int m)//对于数n 求出m进制下各位的和; { int sum = 0; int t; while (n) { t = n % m; sum += t; n /= m; } return sum; } int main() { int a; for (a = 2991; a <= 9999; a++) { if ((f(a, 10) == f(a, 12)) && (f(a, 16) == f(a, 12))) { printf("%d ", a); } } return 0; }
2629:
Problem Description
Do you own an ID card?You must have a identity card number in your family's Household Register. From the ID card you can get specific personal information of everyone. The number has 18 bits,the first 17 bits contain special specially meanings:the first 6 bits represent the region you come from,then comes the next 8 bits which stand for your birthday.What do other 4 bits represent?You can Baidu or Google it.
Here is the codes which represent the region you are in.
However,in your card,maybe only 33 appears,0000 is replaced by other numbers.
Here is Samuel's ID number 331004198910120036 can you tell where he is from?The first 2 numbers tell that he is from Zhengjiang Province,number 19891012 is his birthday date (yy/mm/dd).
Here is the codes which represent the region you are in.

However,in your card,maybe only 33 appears,0000 is replaced by other numbers.
Here is Samuel's ID number 331004198910120036 can you tell where he is from?The first 2 numbers tell that he is from Zhengjiang Province,number 19891012 is his birthday date (yy/mm/dd).
Input
Input will contain 2 parts:
A number n in the first line,n here means there is n test cases. For each of the test cases,there is a string of the ID card number.
A number n in the first line,n here means there is n test cases. For each of the test cases,there is a string of the ID card number.
Output
Based on the table output where he is from and when is his birthday. The format you can refer to the Sample Output.
Sample Input
1
330000198910120036
Sample Output
He/She is from Zhejiang,and his/her birthday is on 10,12,1989 based on the table.

#include <stdio.h> #include <string.h> int main() { char str[20], s[20]; int n; int i; scanf("%d", &n); getchar(); while (n--) { gets(str); if (str[0] == '1') strcpy(s, "Beijing"); else if (str[0] == '7') strcpy(s, "Taiwan"); else if (str[0] == '5') strcpy(s, "Tibet"); else if (str[0] == '2') strcpy(s, "Liaoning"); else if (str[0] == '3' && str[1] == '3') strcpy(s, "Zhejiang"); else if (str[0] == '3' && str[1] == '1') strcpy(s, "Shanghai"); else if (str[0] == '8' && str[1] == '1') strcpy(s, "Hong Kong"); else strcpy(s, "Macao"); printf("He/She is from %s,and his/her birthday is on %c%c,%c%c,%c%c%c%c based on the table. ", s, str[10], str[11], str[12], str[13], str[6], str[7], str[8], str[9]); } }