The Donkey of Gui Zhou
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 308 Accepted Submission(s): 118
Problem Description
There was no donkey in the province of Gui Zhou, China. A trouble maker shipped one and put it in the forest which could be considered as an N×N grid. The coordinates of the up-left cell is (0,0) , the down-right cell is (N-1,N-1) and the cell below the up-left cell is (1,0)..... A 4×4 grid is shown below: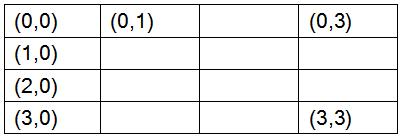
The donkey lived happily until it saw a tiger far away. The donkey had never seen a tiger ,and the tiger had never seen a donkey. Both of them were frightened and wanted to escape from each other. So they started running fast. Because they were scared, they were running in a way that didn't make any sense. Each step they moved to the next cell in their running direction, but they couldn't get out of the forest. And because they both wanted to go to new places, the donkey would never stepped into a cell which had already been visited by itself, and the tiger acted the same way. Both the donkey and the tiger ran in a random direction at the beginning and they always had the same speed. They would not change their directions until they couldn't run straight ahead any more. If they couldn't go ahead any more ,they changed their directions immediately. When changing direction, the donkey always turned right and the tiger always turned left. If they made a turn and still couldn't go ahead, they would stop running and stayed where they were, without trying to make another turn. Now given their starting positions and directions, please count whether they would meet in a cell.
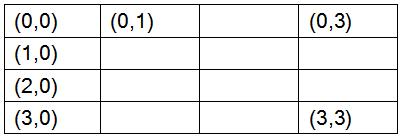
Input
There are several test cases.
In each test case: First line is an integer N, meaning that the forest is a N×N grid.
The second line contains three integers R, C and D, meaning that the donkey is in the cell (R,C) when they started running, and it's original direction is D. D can be 0, 1, 2 or 3. 0 means east, 1 means south , 2 means west, and 3 means north.
The third line has the same format and meaning as the second line, but it is for the tiger.
The input ends with N = 0. ( 2 <= N <= 1000, 0 <= R, C < N)
In each test case: First line is an integer N, meaning that the forest is a N×N grid.
The second line contains three integers R, C and D, meaning that the donkey is in the cell (R,C) when they started running, and it's original direction is D. D can be 0, 1, 2 or 3. 0 means east, 1 means south , 2 means west, and 3 means north.
The third line has the same format and meaning as the second line, but it is for the tiger.
The input ends with N = 0. ( 2 <= N <= 1000, 0 <= R, C < N)
Output
For each test case, if the donkey and the tiger would meet in a cell, print the coordinate of the cell where they meet first time. If they would never meet, print -1 instead.
Sample Input
2
0 0 0
0 1 2
4
0 1 0
3 2 0
0
Sample Output
-1
1 3
Source
杭州网络预选赛第三题,很简单的一道题,只要按照给定的走法一步上步模拟即可
代码是比赛时候写的,略难看。。。

1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #define UP 3 5 #define DOWN 1 6 #define LEFT 2 7 #define RIGHT 0 8 9 using namespace std; 10 11 class POINT 12 { 13 public: 14 int x; 15 int y; 16 bool operator != (const POINT &p) const 17 { 18 if(x==p.x&&y==p.y) 19 return false; 20 return true; 21 } 22 }; 23 24 int n; 25 26 27 int donkey_dir,tiger_dir; 28 bool donkey_end,tiger_end; 29 bool donkey_map[1010][1010],tiger_map[1010][1010]; 30 POINT donkey_current,tiger_current; 31 32 int main() 33 { 34 while(scanf("%d",&n)) 35 { 36 if(n==0) 37 break; 38 memset(donkey_map,false,sizeof(donkey_map)); 39 memset(tiger_map,false,sizeof(tiger_map)); 40 donkey_end=tiger_end=false; 41 int r,c,d; 42 scanf("%d %d %d",&r,&c,&d); 43 POINT temp; 44 temp.x=r+1; 45 temp.y=c+1; 46 donkey_current=temp; 47 donkey_dir=d; 48 scanf("%d %d %d",&r,&c,&d); 49 temp.x=r+1; 50 temp.y=c+1; 51 tiger_current=temp; 52 tiger_dir=d; 53 for(int i=0;i<=n+1;i++) 54 { 55 tiger_map[i][0]=tiger_map[i][n+1]=donkey_map[i][0]=donkey_map[i][n+1]=true; 56 tiger_map[0][i]=tiger_map[n+1][i]=donkey_map[0][i]=donkey_map[n+1][i]=true; 57 } 58 59 while(tiger_current!=donkey_current) 60 { 61 if(donkey_end&&tiger_end) 62 break; 63 if(!donkey_end) 64 { 65 int x=donkey_current.x; 66 int y=donkey_current.y; 67 if(donkey_dir==UP) 68 { 69 if(donkey_map[x-1][y]) 70 { 71 if(donkey_map[x][y+1]) 72 donkey_end=true; 73 else 74 { 75 temp.x=x; 76 temp.y=y+1; 77 donkey_current=temp; 78 donkey_map[x][y]=true; 79 donkey_dir=RIGHT; 80 } 81 } 82 else 83 { 84 temp.x=x-1; 85 temp.y=y; 86 donkey_current=temp; 87 donkey_map[x][y]=true; 88 } 89 } 90 else if(donkey_dir==RIGHT) 91 { 92 if(donkey_map[x][y+1]) 93 { 94 if(donkey_map[x+1][y]) 95 donkey_end=true; 96 else 97 { 98 temp.x=x+1; 99 temp.y=y; 100 donkey_current=temp; 101 donkey_map[x][y]=true; 102 donkey_dir=DOWN; 103 } 104 } 105 else 106 { 107 temp.x=x; 108 temp.y=y+1; 109 donkey_current=temp; 110 donkey_map[x][y]=true; 111 } 112 } 113 else if(donkey_dir==DOWN) 114 { 115 if(donkey_map[x+1][y]) 116 { 117 if(donkey_map[x][y-1]) 118 donkey_end=true; 119 else 120 { 121 temp.x=x; 122 temp.y=y-1; 123 donkey_current=temp; 124 donkey_map[x][y]=true; 125 donkey_dir=LEFT; 126 } 127 } 128 else 129 { 130 temp.x=x+1; 131 temp.y=y; 132 donkey_current=temp; 133 donkey_map[x][y]=true; 134 } 135 } 136 else if(donkey_dir==LEFT) 137 { 138 if(donkey_map[x][y-1]) 139 { 140 if(donkey_map[x-1][y]) 141 donkey_end=true; 142 else 143 { 144 temp.x=x-1; 145 temp.y=y; 146 donkey_current=temp; 147 donkey_map[x][y]=true; 148 donkey_dir=UP; 149 } 150 } 151 else 152 { 153 temp.x=x; 154 temp.y=y-1; 155 donkey_current=temp; 156 donkey_map[x][y]=true; 157 } 158 } 159 } 160 if(!tiger_end) 161 { 162 int x=tiger_current.x; 163 int y=tiger_current.y; 164 if(tiger_dir==UP) 165 { 166 if(tiger_map[x-1][y]) 167 { 168 if(tiger_map[x][y-1]) 169 tiger_end=true; 170 else 171 { 172 temp.x=x; 173 temp.y=y-1; 174 tiger_current=temp; 175 tiger_map[x][y]=true; 176 tiger_dir=LEFT; 177 } 178 } 179 else 180 { 181 temp.x=x-1; 182 temp.y=y; 183 tiger_current=temp; 184 tiger_map[x][y]=true; 185 } 186 } 187 else if(tiger_dir==RIGHT) 188 { 189 if(tiger_map[x][y+1]) 190 { 191 if(tiger_map[x-1][y]) 192 tiger_end=true; 193 else 194 { 195 temp.x=x-1; 196 temp.y=y; 197 tiger_current=temp; 198 tiger_map[x][y]=true; 199 tiger_dir=UP; 200 } 201 } 202 else 203 { 204 temp.x=x; 205 temp.y=y+1; 206 tiger_current=temp; 207 tiger_map[x][y]=true; 208 } 209 } 210 else if(tiger_dir==DOWN) 211 { 212 if(tiger_map[x+1][y]) 213 { 214 if(tiger_map[x][y+1]) 215 tiger_end=true; 216 else 217 { 218 temp.x=x; 219 temp.y=y+1; 220 tiger_current=temp; 221 tiger_map[x][y]=true; 222 tiger_dir=RIGHT; 223 } 224 } 225 else 226 { 227 temp.x=x+1; 228 temp.y=y; 229 tiger_current=temp; 230 tiger_map[x][y]=true; 231 } 232 } 233 else if(tiger_dir==LEFT) 234 { 235 if(tiger_map[x][y-1]) 236 { 237 if(tiger_map[x+1][y]) 238 tiger_end=true; 239 else 240 { 241 temp.x=x+1; 242 temp.y=y; 243 tiger_current=temp; 244 tiger_map[x][y]=true; 245 tiger_dir=DOWN; 246 } 247 } 248 else 249 { 250 temp.x=x; 251 temp.y=y-1; 252 tiger_current=temp; 253 tiger_map[x][y]=true; 254 } 255 } 256 } 257 } 258 if(tiger_current!=donkey_current) 259 cout<<"-1"<<endl; 260 else 261 cout<<tiger_current.x-1<<" "<<tiger_current.y-1<<endl; 262 } 263 264 return 0; 265 }