前言
Mybatis
是一个半自动化ORM(Object Relation Mapping)
框架, 之所以说Mybatis
半自动化,是因为SQL语句需要用户自定义,SQL
的解析,执行等工作由Mybatis
执行,这里记录下SpringBoot
整合Mybatis
的案例。
环境
SpringBoot2.53 + Mybatis2.2.0
具体实现
项目结构
- 项目结构如下所示
项目配置
pom.xml
<!-- web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- jdbc -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!-- mysql -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- mybatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
application.yml
spring:
application:
name: mybatis-learn
# 文件编码 UTF8
mandatory-file-encoding: UTF-8
# 数据源配置,请修改为你项目的实际配置
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/cms?useSSL=false&serverTimezone=UTC&characterEncoding=UTF8
username: root
password: sunday
# mybatis配置(顶头开始写,否则读取不到)
mybatis:
# mapper路径位置
mapper-locations: classpath:mapper/*.xml
configuration:
# 开启下划线转驼峰
map-underscore-to-camel-case: true
server:
port: 8083
Table
CREATE TABLE `product` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(100) COLLATE utf8_unicode_ci DEFAULT NULL,
`create_time` datetime DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci
实现代码
- 启动类
@SpringBootApplication
@MapperScan(basePackages = {"com.coisini.mybatislearn.mapper"}) // 扫描的mapper
public class MybatisLearnApplication {
public static void main(String[] args) {
SpringApplication.run(MybatisLearnApplication.class, args);
}
}
ProductController.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RequestMapping("/product")
@RestController
public class ProductController {
@Autowired
private ProductService productService;
/**
* 查询Products
* @return
*/
@GetMapping("/select")
public List<Product> selectProducts() {
return productService.getProducts();
}
/**
* 查询Products
* @return
*/
@GetMapping("/select1")
public List<Product> getProducts1() {
return productService.getProducts1();
}
/**
* 插入product
* @return
*/
@GetMapping("/insert")
public long insertProduct() {
return productService.insertProduct();
}
}
ProductService.java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ProductService {
@Autowired
private ProductMapper productMapper;
/**
* 查询products
* @return
*/
public List<Product> getProducts() {
return productMapper.getProducts();
}
/**
* 查询products
* @return
*/
public List<Product> getProducts1() {
return productMapper.getProducts1();
}
/**
* 插入product
* @return
*/
public long insertProduct() {
Product product = new Product();
product.setTitle("NewProduct");
productMapper.insertProduct(product);
return product.getId();
}
}
ProductMapper.java
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public interface ProductMapper {
/**
* 查询products
* @return
*/
List<Product> getProducts();
/**
* 查询products
* 注解编写sql
* @return
*/
@Select("select * from product")
List<Product> getProducts1();
/**
* 插入product
* @param product
* @return
*/
long insertProduct(Product product);
}
Product.java
import lombok.Getter;
import lombok.Setter;
import java.util.Date;
@Getter
@Setter
public class Product {
private Integer id;
private String title;
private Date createTime;
}
ProductMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.coisini.mybatislearn.mapper.ProductMapper">
<resultMap id="BaseResultMap" type="com.coisini.mybatislearn.model.Product">
<id column="id" property="id"/>
<result column="title" property="title"/>
<result column="create_time" property="createTime"/>
</resultMap>
<select id="getProducts" resultMap="BaseResultMap">
select * from product
</select>
<!-- useGeneratedKeys="true" 通过jdbc getGeneratedKeys方法获取主键 -->
<!-- keyProperty="id" 映射到resultMap id 属性上 -->
<insert id="insertProduct" useGeneratedKeys="true" keyProperty="id"
parameterType="com.coisini.mybatislearn.model.Product">
INSERT INTO product(title) VALUES (#{title})
</insert>
</mapper>
- 注意事项:
1.mapper-namespace需要与Mapper接口对应
2.mapper接口文件名最好与Mybatis的映射文件名一样
2.标签中的id必须与mapper接口中的方法名一致
测试
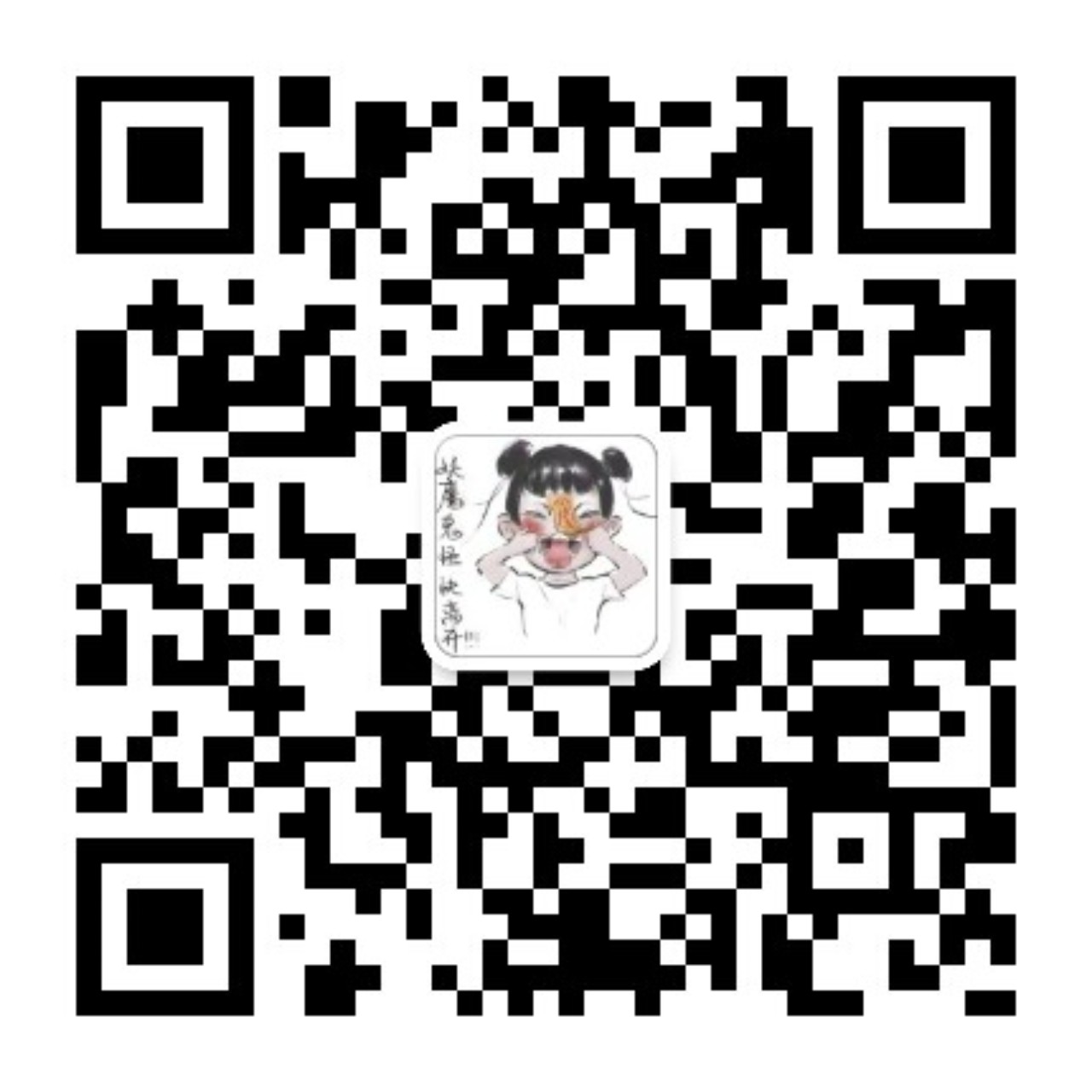