Binary Watch (E)
题目
A binary watch has 4 LEDs on the top which represent the hours (0-11), and the 6 LEDs on the bottom represent the minutes (0-59).
Each LED represents a zero or one, with the least significant bit on the right.
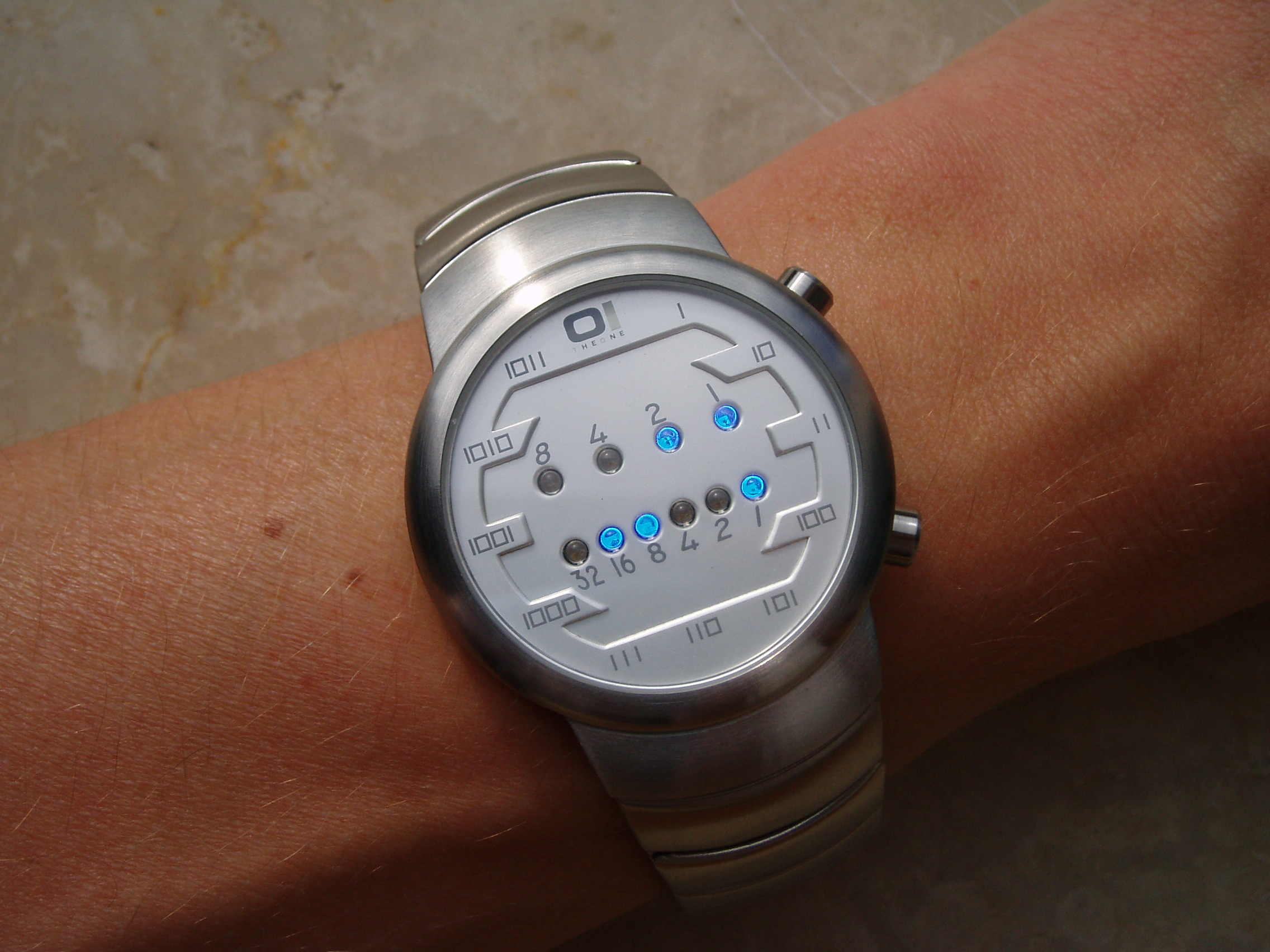
For example, the above binary watch reads "3:25".
Given a non-negative integer n which represents the number of LEDs that are currently on, return all possible times the watch could represent.
Example:
Input: n = 1
Return: ["1:00", "2:00", "4:00", "8:00", "0:01", "0:02", "0:04", "0:08", "0:16", "0:32"]
Note:
- The order of output does not matter.
- The hour must not contain a leading zero, for example "01:00" is not valid, it should be "1:00".
- The minute must be consist of two digits and may contain a leading zero, for example "10:2" is not valid, it should be "10:02".
题意
一个特立独行的手表,用二进制位来表示时间。4位表示小时,6位表示分钟。求在10位中选出n位一共可以得到的时间组合。
思路
回溯法。需要注意其中的坑:小时最大值只能为11,分钟最大值只能为59。
另一种暴力法是,小时数只有12种可能,分钟数只有60种可能,直接暴力搜索每一种组合,判断它们二进制1的位数相加是否为n。
代码实现
Java
回溯法
class Solution {
public List<String> readBinaryWatch(int num) {
List<String> ans = new ArrayList<>();
int[] available = { 1, 2, 4, 8, 101, 102, 104, 108, 116, 132 };
dfs(num, new int[2], available, 0, ans);
return ans;
}
private void dfs(int num, int[] time, int[] available, int index, List<String> ans) {
if (num == 0) {
ans.add(time[0] + ":" + (time[1] < 10 ? "0" + time[1] : time[1]));
return;
}
if (index == 10) {
return;
}
if (available[index] > 100 && time[1] + available[index] - 100 < 60) {
time[1] += available[index] - 100;
dfs(num - 1, time, available, index + 1, ans);
time[1] -= available[index] - 100;
} else if (available[index] < 100 && time[0] + available[index] < 12) {
time[0] += available[index];
dfs(num - 1, time, available, index + 1, ans);
time[0] -= available[index];
}
dfs(num, time, available, index + 1, ans);
}
}
暴力法
class Solution {
public List<String> readBinaryWatch(int num) {
List<String> ans = new ArrayList<>();
for (int h = 0; h < 12; h++) {
for (int m = 0; m < 60; m++) {
if (countOne(h) + countOne(m) == num) {
ans.add(h + ":" + (m < 10 ? "0" + m : m));
}
}
}
return ans;
}
private int countOne(int num) {
int count = 0;
while (num != 0) {
if ((num & 1) == 1) {
count++;
}
num >>= 1;
}
return count;
}
}