Structure Contains | Represents |
CvPoint int x, y | Point in image |
CvPoint2D32f float x, y | Points in R 2 |
CvPoint3D32f float x, y, z | Points in R 3 |
CvSize int width, height | Size of image |
CvRect int x, y, width, height | Portion of image |
CvScalar double val[4] | RGBA value |
cvScalar() , takes one, two, three, or four arguments and assigns those arguments to the corresponding elements of val[] . set四个值
cvRealScalar() ; it takes one argument, which it assigns to val[0] while setting the other entries to 0. set第一个值,其余为0
cvScalarAll() , which takes a single argument but sets all four elements of val[] to that same argument. 全set成同一个值
For all intents andpurposes, an IplImage can be thought of as being derived from CvMat . Therefore, it is best to understand the (would-be) base class before attempting to understand the added complexities of the derived class. A third class, called CvArr , can be thought of as an abstract base class from which CvMat is itself derived. You will oft en see CvArr (or, more accurately, CvArr* ) in function prototypes. When it appears, it is acceptable to pass CvMat* or IplImage* to the routine.
32-bit floats ( CV_32FC1 ),
unsigned integer 8-bit triplets ( CV_8UC3 )

typedef struct CvMat { int type; int step; int* refcount; // for internal use only union { uchar* ptr; short* s; int* i; float* fl; double* db; } data; union { int rows; int height; }; union { int cols; int width; }; } CvMat;
cvCreateMatHeader() creates the CvMat structure without allocating memory for the data.
cvCreateData() handles the data allocation.
cvCreateMat() = cvCreateMatHeader() + cvCreateData() .
cvCloneMat(CvMat*) creates a new matrix from an existing one.*
cvReleaseMat(CvMat**) release.
* cvCloneMat() and other OpenCV functions containing the word “clone” not only create a new header that
is identical to the input header, they also allocate a separate data area and copy the data from the source to
the new object.
* For the regular two-dimensional matrices discussed here, dimension zero (0) is always the “width” and dimension one (1) is always the height.
二维矩阵中,维度0通常是宽,维度1通常是高。
矩阵某个位置的元素:the location of any given point is given by the formula:
δ = ( row ) ⋅ N cols ⋅ N channels + ( col ) ⋅ N channels + ( channel) 通道
IplImage header structure

typedef struct _IplImage { int nSize; int ID; int nChannels; int alphaChannel; int depth; char colorModel[4]; char channelSeq[4]; int dataOrder; int origin; int align; int width; int height; struct _IplROI* roi; struct _IplImage* maskROI; void* imageId; struct _IplTileInfo* tileInfo; int imageSize; char* imageData; int widthStep; int BorderMode[4]; int BorderConst[4]; char* imageDataOrigin; } IplImage;
Th e possible values for nChannels are 1, 2, 3, or 4.
origin : IPL_ORIGIN_TL or IPL_ORIGIN_BL (the origin of coordinates being located in either the upper-left or lower-left corners of the image, respectively.)
dataOrder: IPL_DATA_ORDER_PIXEL or IPL_DATA_ORDER_PLANE .(whether the data should be packed with multiple channels one aft er the other for each pixel (interleaved, the usual case), or rather all of the channels clustered into image planes with the planes placed one aft er another.)
ROI:感兴趣的区域
COI:感兴趣的通道 channel of interest
矩阵和图像操作的方法
cvAbs 计算数组中所有元素的绝对值
cvAbsDiff 计算两个数组差值的绝对值
cvXXX 对两个数组作XXX操作
cvXXXS 对数组和一个固定值作XXX操作
cvXXXRS 对固定值和数组作XXX操作(和cvXXXS相反)
void cvCvtColor(
const CvArr* src,
CvArr* dst,
int code
);
code转换方式
RGB555
RGB565
RGB24
RGB32
HSV(Hue, Saturation, Value)是根据颜色的直观特性由A. R. Smith在1978年创建的一种颜色空间, 也称六角锥体模型(Hexcone Model)。
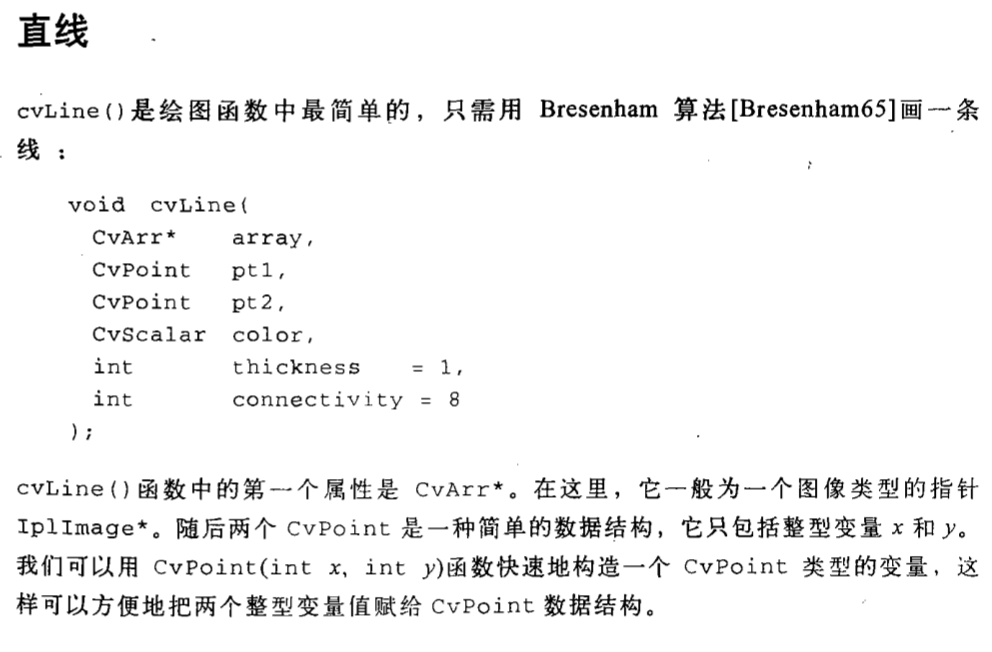
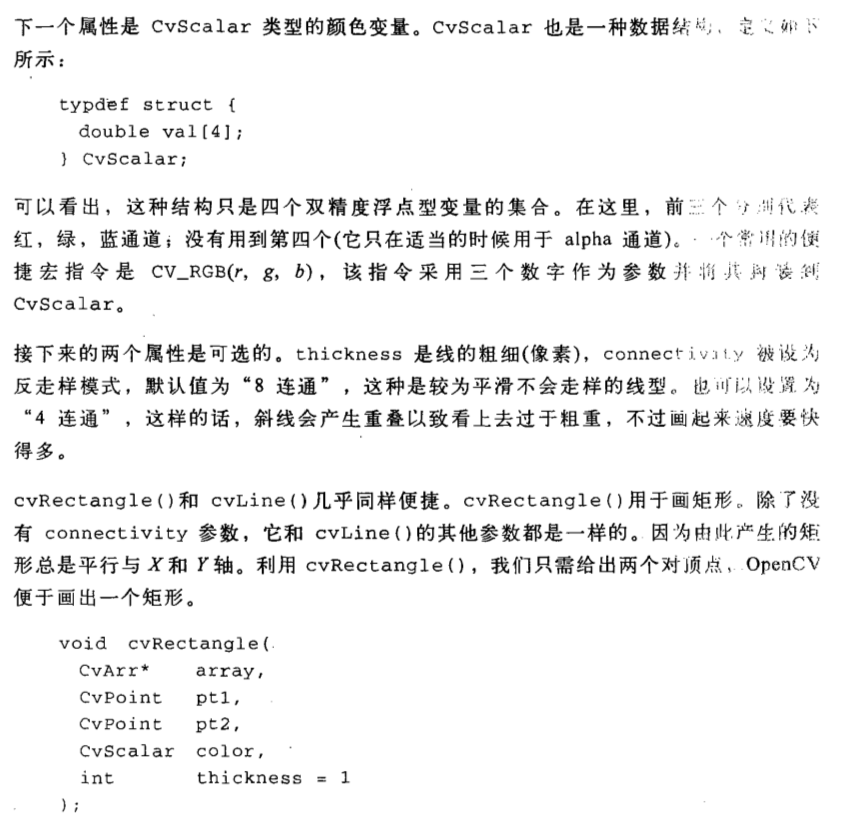
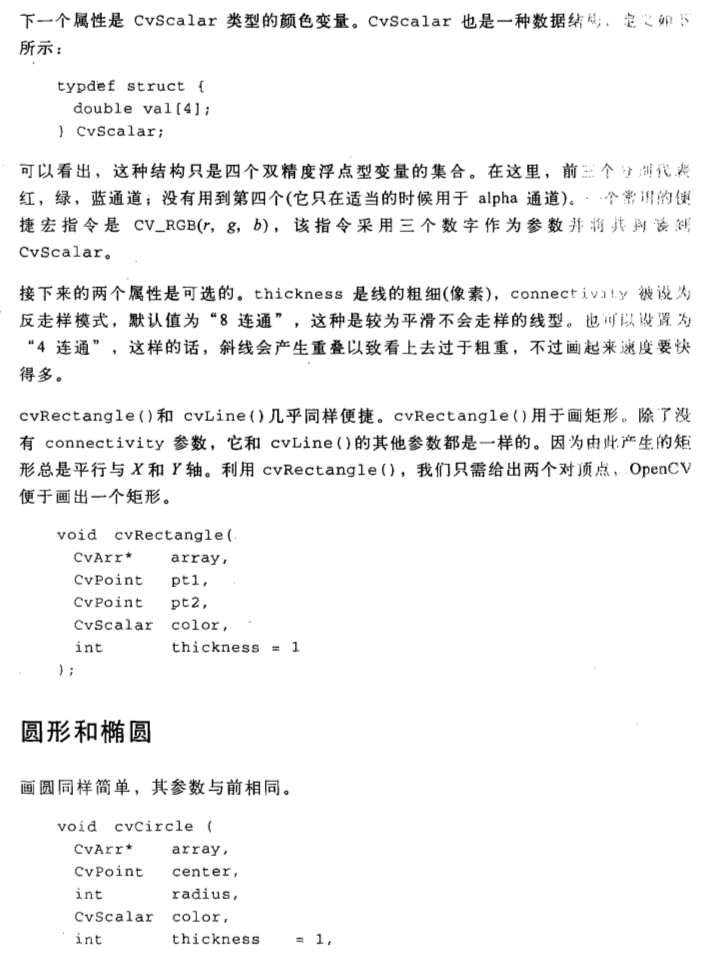
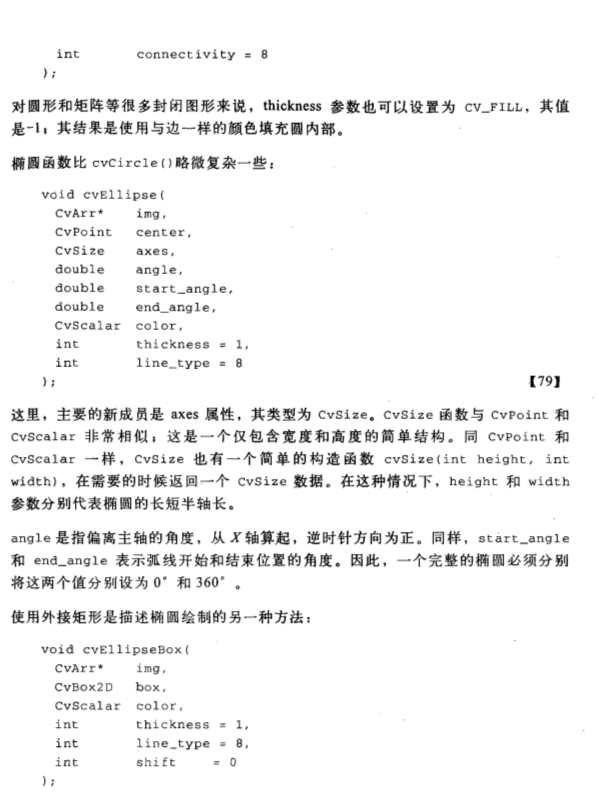
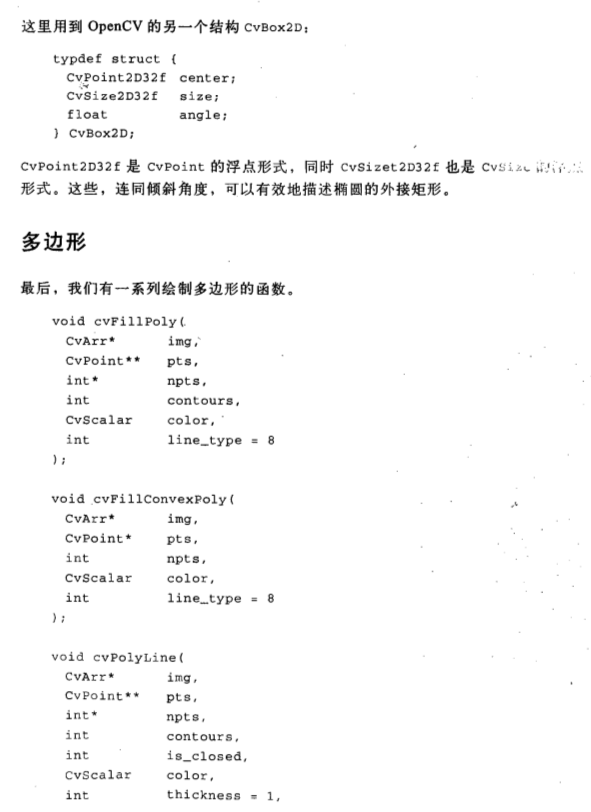
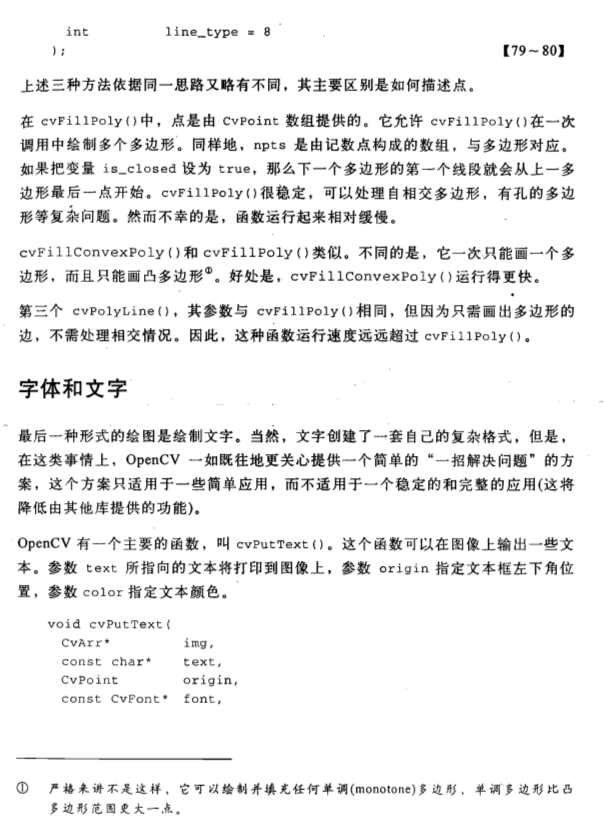
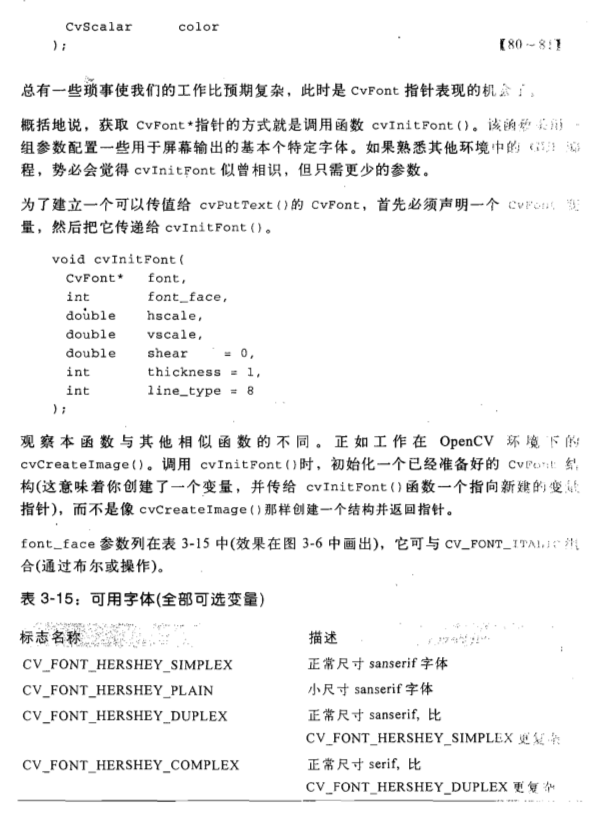
