There are n children in Jzzhu's school. Jzzhu is going to give some candies to them. Let's number all the children from 1 to n. The i-th child wants to get at least ai candies.
Jzzhu asks children to line up. Initially, the i-th child stands at the i-th place of the line. Then Jzzhu start distribution of the candies. He follows the algorithm:
- Give m candies to the first child of the line.
- If this child still haven't got enough candies, then the child goes to the end of the line, else the child go home.
- Repeat the first two steps while the line is not empty.
Consider all the children in the order they go home. Jzzhu wants to know, which child will be the last in this order?
The first line contains two integers n, m (1 ≤ n ≤ 100; 1 ≤ m ≤ 100). The second line contains n integers a1, a2, ..., an (1 ≤ ai ≤ 100).
Output a single integer, representing the number of the last child.
5 2 1 3 1 4 2
4
6 4 1 1 2 2 3 3
6
传送门:点击打开链接
解体思路:简单模拟题,用队列模拟这个过程就可以。
代码:
#include <cstdio> #include <queue> using namespace std; typedef pair<int, int> P; queue<P> q; int main() { #ifndef ONLINE_JUDGE freopen("257Ain.txt", "r", stdin); #endif int n, m, ans = 0; scanf("%d%d", &n, &m); for(int i = 0; i < n; i++) { int x; scanf("%d", &x); q.push(P(x, i + 1)); } while(!q.empty()) { P p = q.front(); q.pop(); if(p.first > m) { p.first -= m; q.push(p); } ans = p.second; } printf("%d ", ans); return 0; }
Problem B
Jzzhu has invented a kind of sequences, they meet the following property:

You are given x and y, please calculate fn modulo 1000000007 (109 + 7).
The first line contains two integers x and y (|x|, |y| ≤ 109). The second line contains a single integer n (1 ≤ n ≤ 2·109).
Output a single integer representing fn modulo 1000000007 (109 + 7).
2 3 3
1
0 -1 2
1000000006
传送门:点击打开链接
解体思路:简单数学公式的推导,
f(n) = f(n-1) + f(n+1), f(n+1) = f(n) + f(n+2);
两式相加得:f(n-1) + f(n+2) = 0,
由上式可推得:f(n+2) + f(n+5) = 0;
由上两式得:f(n-1) = f(n+5),所以f(n)的周期为6;
我们仅仅需求出f的前六项就可以,ps:注意一点,f(n)可能为负值,对负数取模要先对负数加mod,使负数变为正数之后再取模。
代码:
#include <cstdio> const int mod = 1000000007; int main() { #ifndef ONLINE_JUDGE //freopen("257Bin.txt", "r", stdin); #endif int n, a [7]; scanf("%d%d%d", &a[0], &a[1], &n); for(int i = 2; i < 7; i++) a[i] = a[i - 1] - a[i - 2]; int t = a[(n - 1)% 6]; printf("%d ", t >= 0 ? t % mod : (t + 2 * mod) % mod); return 0; }
Problem C
Jzzhu has a big rectangular chocolate bar that consists of n × m unit squares. He wants to cut this bar exactly k times. Each cut must meet the following requirements:
- each cut should be straight (horizontal or vertical);
- each cut should go along edges of unit squares (it is prohibited to divide any unit chocolate square with cut);
- each cut should go inside the whole chocolate bar, and all cuts must be distinct.
The picture below shows a possible way to cut a 5 × 6 chocolate for 5 times.
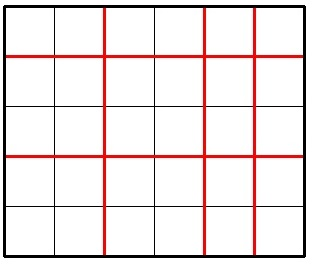
Imagine Jzzhu have made k cuts and the big chocolate is splitted into several pieces. Consider the smallest (by area) piece of the chocolate, Jzzhu wants this piece to be as large as possible. What is the maximum possible area of smallest piece he can get with exactly k cuts? The area of a chocolate piece is the number of unit squares in it.
A single line contains three integers n, m, k (1 ≤ n, m ≤ 109; 1 ≤ k ≤ 2·109).
Output a single integer representing the answer. If it is impossible to cut the big chocolate k times, print -1.
3 4 1
6
6 4 2
8
2 3 4
-1
解体思路:
n行m列,在水平方向最多切n-1刀,竖直方向最多切m-1刀,假设k>n+m-2,就是不能分割的情况;我们找出沿水平方向或竖直方向能够切的最多的刀数mx,假设k>mx,我们就如今这个方向切mx刀,剩下的就是要将一条长为(mn+1)巧克力切(k - mx)刀;其它的情况就是要么就是沿着水平方向切k刀,要么就是沿着竖直方向切k刀,取两者间的大者。
代码:
#include <cstdio> #include <iostream> #include <algorithm> using namespace std; int main() { int n, m, k; long long ans = -1; cin >> n >> m >> k; if(k > n + m -2) ans = -1; else { int mx = max(n - 1, m - 1); int mn = min(n - 1, m - 1); if(k > mx) ans = (mn + 1) / (k - mx + 1); else ans = max(1ll * n / (k + 1) * m, 1ll * m / (k + 1) * n); } cout << ans << endl; return 0; }