
Code
1
2
3
4
namespace ConsoleApplication1
5

{
6
using System.Data;
7
using System;
8
class Program
9
{
10
static void Main(string[] args)
11
{
12
13
TestTableRowState();
14
}
15
16
public static void TestTableRowState()
17
{
18
DataTable table = new DataTable("MasterTable");
19
20
DataColumn column;
21
DataRow row;
22
23
column = new DataColumn();
24
column.DataType = System.Type.GetType("System.Int32");
25
column.ColumnName = "id";
26
column.ColumnMapping = MappingType.Hidden;
27
column.ReadOnly = true;
28
column.Unique = true;
29
table.Columns.Add(column);
30
31
column = new DataColumn();
32
column.DataType = System.Type.GetType("System.String");
33
column.ColumnName = "FirstName";
34
column.AutoIncrement = false;
35
column.Caption = "第一字段";
36
column.ReadOnly = false;
37
column.Unique = false;
38
table.Columns.Add(column);
39
40
DataColumn[] PrimaryKeyColumns = new DataColumn[1];
41
PrimaryKeyColumns[0] = table.Columns["id"];
42
table.PrimaryKey = PrimaryKeyColumns;
43
44
DataSet dataset = new DataSet();
45
dataset.Tables.Add(table);
46
47
int rowCount = 10;
48
for (int i = 0; i < rowCount; i++)
49
{
50
row = table.NewRow();
51
row["id"] = i;
52
row["FirstName"] = "liao";
53
table.Rows.Add(row);
54
Console.WriteLine("The Row_{0}'s RowState is {1}.", i.ToString(), row.RowState.ToString());
55
}
56
Console.WriteLine("The table RowsCount is {0}.", rowCount);
57
58
//新添加一行
59
row = table.NewRow();
60
row["id"] = 100;
61
row["FirstName"] = "liao";
62
table.Rows.Add(row);
63
table.AcceptChanges();
64
rowCount = table.Rows.Count;
65
Console.WriteLine();
66
Console.WriteLine("The Row_{0}'s RowState is {1}.", rowCount, row.RowState.ToString());
67
Console.WriteLine("The table RowsCount is {0}.", rowCount);
68
Console.WriteLine();
69
70
//删除新添加的行
71
table.Rows.Remove(row);
72
rowCount = table.Rows.Count;
73
for (int i = 0; i < rowCount; i++)
74
{
75
Console.WriteLine("The Row_{0}'s RowState is {1}.", i.ToString(), row.RowState.ToString());
76
}
77
78
Console.WriteLine("The table RowsCount is {0}.", rowCount);
79
80
81
//查询RowState的行
82
DataRow[] delRows = table.Select(null, null, DataViewRowState.Deleted);
83
84
Console.WriteLine("Deleted rows:\n");
85
86
foreach (DataColumn catCol in table.Columns)
87
Console.Write(catCol.ColumnName + "\t");
88
Console.WriteLine();
89
90
foreach (DataRow delRow in delRows)
91
{
92
foreach (DataColumn catCol in table.Columns)
93
Console.Write(delRow[catCol, DataRowVersion.Original] + "\t");
94
Console.WriteLine();
95
}
96
97
Console.ReadLine();
98
}
99
}
100
}
101
运行结果如下:
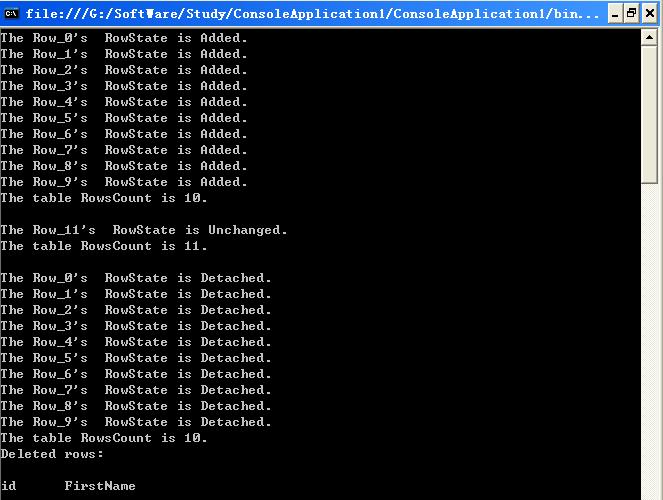
问题,为什么第二次输出的RowState 是Detached呢?
不解!希望帮忙解析一下。