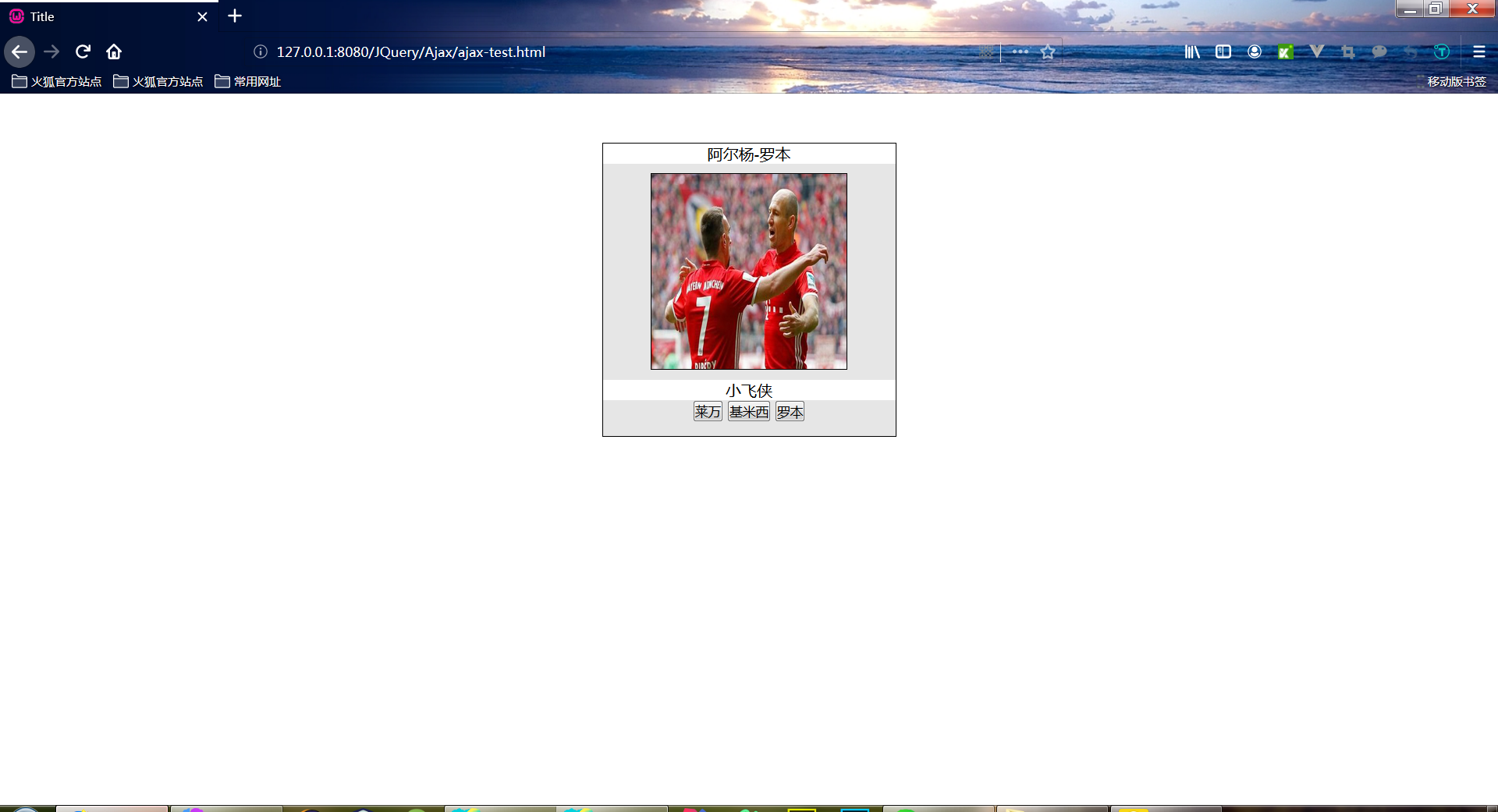
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <title>Title</title> 6 <style type="text/css"> 7 *{ 8 padding:0; 9 margin: 0; 10 } 11 div{ 12 width: 300px; 13 height: 300px; 14 border: 1px solid black; 15 margin: 50px auto; 16 text-align: center; 17 background: #e6e6e6; 18 } 19 img{ 20 width: 200px; 21 height: 200px; 22 display: block; 23 margin: 10px auto 10px; 24 border: 1px solid black; 25 } 26 p{ 27 text-align: center; 28 background-color:#fff; 29 30 } 31 </style> 32 <script src="myAjax.js"></script> 33 <script> 34 window.onload = function (ev) { 35 var oTitle = document.querySelector("#title"); 36 var oDes = document.querySelector("#des"); 37 var oImg = document.querySelector("img"); 38 39 var oBtns = document.querySelectorAll("button"); 40 41 42 oBtns[0].onclick = function () { 43 var self = this; 44 ajax({ 45 type:"get", 46 url:"ajax-test1.php", 47 data:{ 48 "name":this.getAttribute("name") 49 }, 50 timeout:5000, 51 success:function (xhr) { 52 /*向ajax后台的程序发送xmlhttp请求的时候, 后台程序接到请求会进行处理,处理结束后,可以返回一串数据给前台, 53 这个就是responseText. 54 */ 57 var res = xhr.responseText.split("|"); 58 oTitle.innerHTML = res[0]; 59 oDes.innerHTML = res[1]; 60 oImg.setAttribute("src",res[2]); 83 }, 84 error:function (xhr) { 85 alert(xhr.status); 86 } 87 }); 88 } 89 oBtns[1].onclick = function () { 90 var self = this; 91 ajax({ 92 type: "get", 93 url: "ajax-test2.php", 94 data: { 95 "name": this.getAttribute("name") 96 }, 97 timeout: 5000, 98 success: function (xhr) { 99 100 var name = self.getAttribute("name"); 101 var res = xhr.responseXML; 102 103 var title = res.querySelector(name + ">title").innerHTML; 104 var des = res.querySelector(name + ">des").innerHTML; 105 var image = res.querySelector(name + ">image").innerHTML; 106 107 oTitle.innerHTML = title; 108 oDes.innerHTML = des; 109 oImg.setAttribute("src", image);}, 110 error:function (xhr) { 111 alert(xhr.status); 112 } 113 }); 114 115 } 116 oBtns[2].onclick = function () { 117 var self = this; 118 ajax({ 119 type:"get", 120 url:"ajax-test3.php", 121 data:{ 122 "name":this.getAttribute("name") 123 }, 124 timeout:5000, 125 success:function (xhr) { 126 127 var str = xhr.responseText; 128 var obj = JSON.parse(str); 129 var name = obj.tx; 130 oTitle.innerHTML = name.title; 131 oDes.innerHTML = name.des; 132 oImg.setAttribute("src",name.image); 133 134 }, 135 error:function (xhr) { 136 alert(xhr.status); 137 } 138 }); 139 }; 140 } 141 </script> 142 </head> 143 <body> 144 <div> 145 <p id="title">拜仁球星</p> 146 <img src="" alt=""> 147 <p id="des">球星介绍</p> 148 <button name="nz">莱万</button> 149 <button name="bb">基米西</button> 150 <button name="tx">罗本</button></div> 151 </body> 152 </html>
ajax-test1.php
1 <?php 2 //1.定义字典保存商品信息 3 $products = array("nz"=>array("title"=>"莱万","des"=>"最佳射手","image"=>"../images/1.jpg"), 4 "bb"=>array("title"=>"基米西","des"=>"鸡哥最可爱","image"=>"../images/2.jpg"), 5 "tx"=>array("title"=>"罗本","des"=>"小飞侠","image"=>"../images/3.jpg")); 6 //2.获取前端传递的参数$_GET 7 $name = $_GET["name"]; 8 //echo $name; 9 //3.根据前端传入的key,获取对应的字典 10 $product = $products[$name]; 11 //print_r($product); 12 //4.将内容取出来,返回给前端 13 echo $product["title"]; 14 echo "|"; 15 echo $product["des"]; 16 echo "|"; 17 echo $product["image"];
ajax-test2.php
1 <?php 2 //执行结果中有中文,必须在PHP文件顶部设置 3 header("content-type:text/html;charset=utf-8"); 4 //如果PHP中需要返回XML数据,也必须在PHP文件顶部设置 5 header("content-type:text/xml;charset=utf-8"); 6 echo file_get_contents("ajax-test.xml"); 8 ?>
ajax-test.xml
1 <?xml version="1.0" encoding="UTF-8" ?> 2 <products> 3 <nz> 4 <title>莱万</title> 5 <des>最佳射手</des> 6 <image>../images/1.jpg</image> 7 </nz> 8 <bb><title>基米西</title> 9 <des>可爱</des> 10 <image>../images/2.jpg</image></bb> 11 <tx><title>罗本</title> 12 <des>小飞侠</des> 13 <image>../images/3.jpg</image></tx> 14 </products>
ajax-test3.php
1 <?php 2 3 echo file_get_contents("ajax-test.txt"); 4 ?>
ajax-test.txt
{ "nz":{ "title":"莱万", "des":"最佳射手", "image":"../images/1.jpg"}, "bb":{"title":"基米西", "des":"可爱", "image":"../images/2.jpg"}, "tx":{"title":"阿尔杨-罗本", "des":"小飞侠", "image":"../images/3.jpg"} }