需求
编写全流程接口测试用例时,有时候需要进行一些redis操作,比如读取或删除缓存信息。
实现
1.生成RedisTemplate
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.RedisPassword;
import org.springframework.data.redis.connection.RedisStandaloneConfiguration;
import org.springframework.data.redis.connection.jedis.JedisClientConfiguration;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.serializer.JdkSerializationRedisSerializer;
import org.springframework.data.redis.serializer.RedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import own.slim.http.HttpFixture;
import redis.clients.jedis.JedisPoolConfig;
public class RedisInit {
private final static Logger logger = LoggerFactory.getLogger(RedisInit.class);
private JedisPoolConfig jedisPoolConfig;
private RedisConnectionFactory redisConnectionFactory;
private StringRedisTemplate stringRedisTemplate;
private RedisTemplate redisTemplate;
private String database = "0";
private String hostname;
private String port = "6379";
private String passwd = null;
public RedisInit(String hostname) {
this.hostname = hostname;
}
public RedisInit(String database, String hostname, String port) {
this.database = database;
this.hostname = hostname;
this.port = port;
}
public RedisInit(String database, String hostname, String port, String passwd) {
this.database = database;
this.hostname = hostname;
this.port = port;
this.passwd = passwd;
}
public StringRedisTemplate getStringRedisTemplate() {
logger.info("redis initial, database:{}, hostname:{}, port:{}, passwd:{}",
database, hostname, port, passwd);
jedisPoolConfig = new JedisPoolConfig();
//最大连接数
jedisPoolConfig.setMaxTotal(100);
//最小空闲连接数
jedisPoolConfig.setMinIdle(20);
//当池内没有可用的连接时,最大等待时间
jedisPoolConfig.setMaxWaitMillis(10000);
//单机版jedis
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration();
//设置redis服务器的host或者ip地址
redisStandaloneConfiguration.setHostName(hostname);
//设置默认使用的数据库
redisStandaloneConfiguration.setDatabase(Integer.parseInt(database));
//设置密码
if (null != this.passwd) {
redisStandaloneConfiguration.setPassword(RedisPassword.of(this.passwd));
}
//设置redis的服务的端口号
redisStandaloneConfiguration.setPort(Integer.parseInt(port));
//获得默认的连接池构造器
JedisClientConfiguration.JedisPoolingClientConfigurationBuilder jpcb =
(JedisClientConfiguration.JedisPoolingClientConfigurationBuilder) JedisClientConfiguration.builder();
//指定jedisPoolConifig来修改默认的连接池构造器
jpcb.poolConfig(jedisPoolConfig);
//通过构造器来构造jedis客户端配置
JedisClientConfiguration jedisClientConfiguration = jpcb.build();
redisConnectionFactory = new JedisConnectionFactory(redisStandaloneConfiguration, jedisClientConfiguration);
stringRedisTemplate = new StringRedisTemplate(redisConnectionFactory);
// RedisSerializer<String> redisSerializer = new StringRedisSerializer();//Long类型不可以会出现异常信息;
// stringRedisTemplate.setKeySerializer(redisSerializer);
// stringRedisTemplate.setHashKeySerializer(redisSerializer);
return stringRedisTemplate;
}
public RedisTemplate getRedisTemplate() {
logger.info("redis initial, database:{}, hostname:{}, port:{}, passwd:{}",
database, hostname, port, passwd);
jedisPoolConfig = new JedisPoolConfig();
//最大连接数
jedisPoolConfig.setMaxTotal(100);
//最小空闲连接数
jedisPoolConfig.setMinIdle(20);
//当池内没有可用的连接时,最大等待时间
jedisPoolConfig.setMaxWaitMillis(10000);
//单机版jedis
RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration();
//设置redis服务器的host或者ip地址
redisStandaloneConfiguration.setHostName(hostname);
//设置默认使用的数据库
redisStandaloneConfiguration.setDatabase(Integer.parseInt(database));
//设置密码
if (null != this.passwd) {
redisStandaloneConfiguration.setPassword(RedisPassword.of(this.passwd));
}
//设置redis的服务的端口号
redisStandaloneConfiguration.setPort(Integer.parseInt(port));
//获得默认的连接池构造器
JedisClientConfiguration.JedisPoolingClientConfigurationBuilder jpcb =
(JedisClientConfiguration.JedisPoolingClientConfigurationBuilder) JedisClientConfiguration.builder();
//指定jedisPoolConifig来修改默认的连接池构造器
jpcb.poolConfig(jedisPoolConfig);
//通过构造器来构造jedis客户端配置
JedisClientConfiguration jedisClientConfiguration = jpcb.build();
redisConnectionFactory = new JedisConnectionFactory(redisStandaloneConfiguration, jedisClientConfiguration);
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<String, Object>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
JdkSerializationRedisSerializer redisSerializer = new JdkSerializationRedisSerializer();
redisTemplate.setKeySerializer(redisSerializer);
redisTemplate.setHashKeySerializer(redisSerializer);
redisTemplate.afterPropertiesSet();
return redisTemplate;
}
}
2.编写构造函数和成员变量
private String database = "0";
private String hostname;
private String port = "6379";
private String passwd = null;
private StringRedisTemplate stringRedisTemplate;
private RedisTemplate redisTemplate;
public RedisFixture(String hostname) {
this.hostname = hostname;
initStringRedisTemplate();
}
public RedisFixture(String database, String hostname, String port) {
this.database = database;
this.hostname = hostname;
this.port = port;
initStringRedisTemplate();
}
public RedisFixture(String database, String hostname, String port, String passwd) {
this.database = database;
this.hostname = hostname;
this.port = port;
this.passwd = passwd;
initStringRedisTemplate();
}
private void initStringRedisTemplate() {
String key = database + hostname + port;
if (RedisContext.getInstance().StringRedisTemplateMap.containsKey(key)) {
stringRedisTemplate = RedisContext.getInstance().StringRedisTemplateMap.get(key);
} else {
RedisInit redisInit = new RedisInit(database, hostname, port, passwd);
stringRedisTemplate = redisInit.getStringRedisTemplate();
RedisContext.getInstance().StringRedisTemplateMap.put(key, stringRedisTemplate);
}
if (RedisContext.getInstance().redisTemplateMap.containsKey(key)) {
redisTemplate=RedisContext.getInstance().redisTemplateMap.get(key);
myRedisAssistant=RedisContext.getInstance().myRedisAssistantMap.get(key);
} else {
RedisInit redisInit = new RedisInit(database, hostname, port, passwd);
redisTemplate= redisInit.getRedisTemplate();
RedisContext.getInstance().redisTemplateMap.put(key,redisTemplate);
myRedisAssistant=new MyRedisAssistantImpl();
myRedisAssistant.setRedisTemplate(redisTemplate);
RedisContext.getInstance().myRedisAssistantMap.put(key,myRedisAssistant);
}
}
2.实现方法(关键字)
public String read(String path) {
String value = "null";
if (stringRedisTemplate.hasKey(path)) {
value = stringRedisTemplate.opsForValue().get(path);
} else {
value = "the key is error, please check!!!";
}
logger.info("read path:{},and value is {}", path, value);
return value;
}
public boolean del(String path) {
boolean flag = false;
if (stringRedisTemplate.hasKey(path)) {
stringRedisTemplate.delete(path);
flag = true;
} else {
flag = true;
}
logger.info("del path:{},and result is {}", path, flag);
return flag;
}
使用
1.引入类对应package
|import |
|own.slim.redis|
2.编写脚本
|script|redis fixture|0 |172.20.0.59 |6379|
|ensure|add; |!-fitnesse:redis:test-!|{"code":"123456"}|1 |
3.测试
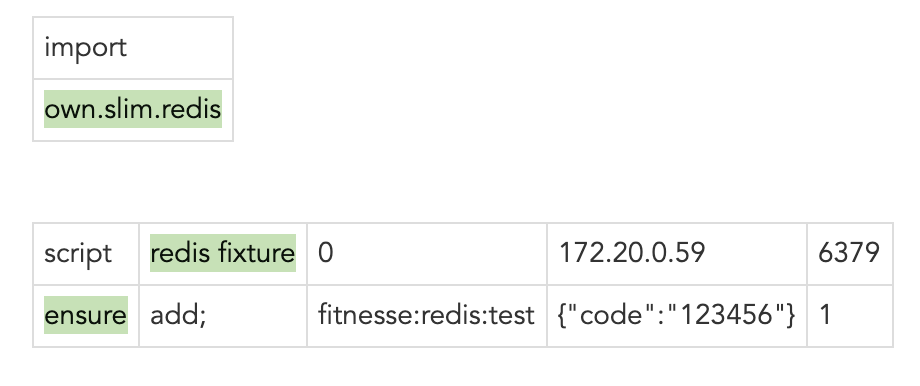

总结
Redis的关键字妙用很多,不仅可以用于操作被测系统的缓存信息,还可以服务于测试框架自身,比如可以在redis储存一些系统相关的变量信息,以及测试结果等。