jQuery 库 - 特性
jQuery 是一个 JavaScript 函数库。
jQuery 库包含以下特性:
- HTML 元素选取
- HTML 元素操作
- CSS 操作
- HTML 事件函数
- JavaScript 特效和动画
- HTML DOM 遍历和修改
- AJAX
- Utilities
jQuery 库位于一个 JavaScript 文件中,其中包含了所有的 jQuery 函数。
可以通过下面的标记把 jQuery 添加到网页中:
<head>
<script type="text/javascript" src="jquery.js"></script>
</head>
请注意,<script> 标签应该位于页面的 <head> 部分
<html> <head>隐藏了p开头的两段<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript"> $(document).ready(function(){ $("button").click(function(){$("p").hide();
}); }); </script> </head> <body> <h2>This is a heading</h2> <p>This is a paragraph.</p> <p>This is another paragraph.</p> <button type="button">Click me</button> </body> </html>
jQuery 语法是通过选取 HTML 元素的,并对元素执行某些操作
基础语法: $(selector).action()
- 美元符号定义 jQuery
- 选择符(selector)"查询"和"查找" HTML 元素
- jQuery 的 action() 执行对元素的操作
实例:
$(this).hide() - 隐藏当前元素
$("p").hide() - 隐藏所有段落
$("p.test").hide() - 隐藏所有 class="test" 的段落
$("#test").hide() - 隐藏所有 id="test" 的元素
您也许已经注意到在我们的实例中的所有 jQuery 函数位于一个 document ready 函数中:
// jQuery methods go here...
});
这是为了防止文档在完全加载(就绪)之前运行 jQuery 代码。
如果在文档没有完全加载之前就运行函数,操作可能失败。下面是两个具体的例子:
- 试图隐藏一个不存在的元素
- 获得未完全加载的图像的大小
提示:简洁写法(与以上写法效果相同):
// jQuery methods go here...
});
以上两种方式你可以选择你喜欢的方式实现文档就绪后执行jQuery方法。
2.jQuery 选择器
jQuery 选择器基于元素的id,类,类型,属性,属性值等用于 "查找" (或选择) HTML 元素。 它基于已经存在的 CSS 选择器, 除此之外,它还有一些自定义的选择器。
jQuery中所有选择器都以美元符号开头: $().
元素选择器
jQuery 元素选择器基于元素名选取元素。
在页面中选取所有 <p> 元素:
<head>
<script>
$(document).ready(function(){ // 在头部执行 button按钮 click点击 p标签隐藏
$("button").click(function(){
$("p").hide();
});
});
</script>
</head>
<h2>这是一个标题</h2>
<p>这是一个段落。</p>
<p>这是另一个段落。</p>
<button>点我</button>
</body>
</html>
#id 选择器
jQuery #id 选择器选择HTML 元素的 id 属性选取指定的元素。
页面中元素的 id 应该是唯一的,所以你要在页面中选取唯一的元素需要同通过 #id 选择器。
通过id选取元素语法如下:
.class 选择器
jQuery 类选择器可以通过指定的class查找元素。
语法如下:
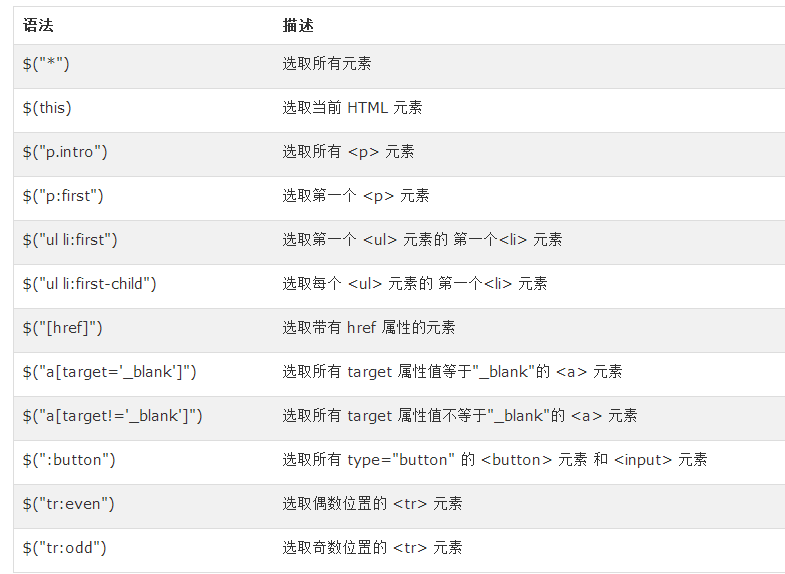
页面对不同访问者的响应叫做事件。
事件处理程序指的是当 HTML 中发生某些事件时所调用的方法。术语由事件"触发"(或"激发")经常会被使用。
实例s:
- 在元素上移动鼠标。
- 选取单选按钮
- 点击元素
术语由事件"触发"(或"激发")经常会被使用。实例: "按钮事件在你按下按键时触发"。
jQuery 事件方法语法
在jQuery中,大多数DOM事件都有一个等效的jQuery方法。
页面中指定一个点击事件:
下一步是定义什么时间触发事件。你可以 通过一个事件函数实现:
// action goes here!!
});
常用的jQuery事件方法
$(document).ready()
$(document).ready() 方法允许我们在文档完全加载完后执行函数。该事件方法在 jQuery 语法 章节中已经提到。
click()
click() 方法是当按钮点击事件被触发时会调用一个函数。
该函数在用户点击HTMl元素时执行。
dblclick()
当双击元素时,会发生 dblclick 事件。
dblclick() 方法触发 dblclick 事件,或规定当发生 dblclick 事件时运行的函数:
mouseenter()
当鼠标指针穿过元素时,会发生 mouseenter 事件。
mouseenter() 方法触发 mouseenter 事件,或规定当发生 mouseenter 事件时运行的函数:
<html><head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){ //开始页面加载jquery
$("#p1").mouseenter(function(){
alert("您的鼠标移到了 id=p1 的元素上!");
});
});
</script>
</head>
<body>
<p id="p1">鼠标指针进入此处,会看到弹窗。</p>
</body>
</html>
mouseleave()
当鼠标指针离开元素时,会发生 mouseleave 事件。
mouseleave() 方法触发 mouseleave 事件,或规定当发生 mouseleave 事件时运行的函数:
mousedown()
当鼠标指针移动到元素上方,并按下鼠标按键时,会发生 mousedown 事件。
mousedown() 方法触发 mousedown 事件,或规定当发生 mousedown 事件时运行的函数:
mouseup()
当在元素上放松鼠标按钮时,会发生 mouseup 事件。
mouseup() 方法触发 mouseup 事件,或规定当发生 mouseup 事件时运行的函数:
hover()
hover()方法用于模拟光标悬停事件。
当鼠标移动到元素上时,会触发指定的第一个函数(mouseenter);当鼠标移出这个元素时,会触发指定的第二个函数(mouseleave)。
focus()
当元素获得焦点时,发生 focus 事件。
focus() 方法触发 focus 事件,或规定当发生 focus 事件时运行的函数:
<html><head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("input").focus(function(){
$(this).css("background-color","#cccccc");
});
$("input").blur(function(){
$(this).css("background-color","#ffffff");
});
});
</script>
</head>
<body>
Name: <input type="text" name="fullname"><br>
Email: <input type="text" name="email">
</body>
</html>
blur()
当元素失去焦点时发生 blur 事件。
当通过鼠标点击选中元素或通过 tab 键定位到元素时,该元素就会获得焦点:
jQuery HTML
jQuery 拥有可操作 HTML 元素和属性的强大方法。1.jQuery DOM 操作
jQuery 中非常重要的部分,就是操作 DOM 的能力。
jQuery 提供一系列与 DOM 相关的方法,这使访问和操作元素和属性变得很容易。
DOM = Document Object Model(文档对象模型) DOM 定义访问 HTML 和 XML 文档的标准: "W3C 文档对象模型独立于平台和语言的界面,允许程序和脚本动态访问和更新文档的内容、结构以及样式。" |
下面的例子演示如何通过 jQuery text() 和 html() 方法来获得内容:
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("#btn1").click(function(){
alert("Text: " + $("#test").text()); //$("#test").text() #test是p段落id的test .text()获得内容 text() - 设置或返回所选元素的文本内容
});
$("#btn2").click(function(){
alert("HTML: " + $("#test").html()); //$("#test").html() #.html()获得内容 html() - 设置或返回所选元素的内容(包括 HTML 标记)
});
});
</script>
</head>
<body>
<p id="test">This is some <b>bold</b> text in a paragraph.</p> //html有<b>
<button id="btn1">Show Text</button> //button按钮
<button id="btn2">Show HTML</button>
</body>
</html>
下面的例子演示如何通过 jQuery val() 方法获得输入字段的值:
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
alert("Value: " + $("#test").val()); // val() - 设置或返回表单字段的值
});
});
</script>
</head>
<body>
<p>Name: <input type="text" id="test" value="Mickey Mouse"></p>
<button>Show Value</button>
</body>
</html>
获取属性 - attr()
下面的例子演示如何获得链接中 href 属性的值:
<html><head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
alert($("#w3s").attr("href")); //id w3s attr("href")属性值
});
});
</script>
</head>
<body>
<p><a href="/" id="w3s">ziqiangxuetang.com</a></p>
<button>Show href Value</button>
</body>
</html>
设置内容 - text()、html() 以及 val()
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
<script>
$(document).ready(function(){
$("#btn1").click(function(){
$("#test1").text("Hello world!"); //往 id="test1" This is a paragraph. 内容设置为Hello world!
});
$("#btn2").click(function(){
$("#test2").html("<b>Hello world!</b>");
});
$("#btn3").click(function(){
$("#test3").val("Dolly Duck");
});
});
</script>
</head>
<body>
<p id="test1">This is a paragraph.</p>
<p id="test2">This is another paragraph.</p>
<p>Input field: <input type="text" id="test3" value="Mickey Mouse"></p>
<button id="btn1">Set Text</button>
<button id="btn2">Set HTML</button>
<button id="btn3">Set Value</button>
</body>
</html>
回调函数:
A要完成1件事,但对这件事的某个环节不清楚,必须通过B来完成这个环节;
问题是B完成这个环节的时间是不确定的,A如果一直问B,效率会很差;
但B是能够准确知道完成时间的,完成的时候B会给A一个通知,告诉A,你可以行动了,并把完成的结果(参数)告诉A
这样双方都不累;
实际操作的时候,A只要把某个函数fun写上自己的实现即可,但这个函数是B声明的,并且B在适当的时候调用这个函数,这个函数就是回调函数
text()、html() 以及 val() 的回调函数
上面的三个 jQuery 方法:text()、html() 以及 val(),同样拥有回调函数。回调函数由两个参数:被选元素列表中当前元素的下标,以及原始(旧的)值。然后以函数新值返回您希望使用的字符串。
<head>
<script>
$(document).ready(function(){
$("#btn1").click(function(){ //id="btn1" 点击
});
});
$("#btn2").click(function(){
$("#test2").html(function(i,origText){
return "Old html: " + origText + " New html: Hello <b>world!</b> (index: " + i + ")";
});
});
});
</script>
</head>
<body>
<p id="test1">This is a <b>bold</b> paragraph.</p>
<p id="test2">This is another <b>bold</b> paragraph.</p>
<button id="btn1">Show Old/New Text</button>
<button id="btn2">Show Old/New HTML</button>
</body>
</html>
jQuery attr() 方法也用于设置/改变属性值。
下面的例子演示如何改变(设置)链接中 href 属性的值:
<head>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#w3s").attr("href","/jquery");
});
});
</script>
</head>
<body>
<p><a href="/" id="w3s">ziqiangxuetang.com</a></p>
<button>Change href Value</button>
<p>Mouse over the link (or click on it) to see that the value of the href attribute has changed.</p>
</body>
</html>
attr() 方法也允许您同时设置多个属性。
下面的例子演示如何同时设置 href 和 title 属性:
<html><head>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#w3s").attr({
"href" : "/jquery",
"title" : "ZiQiangXueTang jQuery Tutorial"
});
});
});
</script>
</head>
<body>
<p><a href="/" id="w3s">ziqiangxuetang.com</a></p>
<button>Change href and title</button>
<p>Mouse over the link to see that the href attribute has changed and a title attribute is set.</p>
</body>
</html>
attr() 的回调函数
jQuery 方法 attr(),也提供回调函数。回调函数由两个参数:被选元素列表中当前元素的下标,以及原始(旧的)值。然后以函数新值返回您希望使用的字符串。
<html><head>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#w3s").attr("href", function(i,origValue){
return origValue + "/jquery";
});
});
});
</script>
</head>
<body>
<p><a href="/" id="w3s">ziqiangxuetang.com</a></p>
<button>Change href Value</button>
<p>Mouse over the link (or click on it) to see that the value of the href attribute has changed.</p>
</body>
</html>
jQuery 添加元素
- append() - 在被选元素的结尾插入内容
- prepend() - 在被选元素的开头插入内容
- after() - 在被选元素之后插入内容
- before() - 在被选元素之前插入内容
jQuery append() 方法在被选元素的结尾插入内容。
$("p").append("Some appended text.");
jQuery prepend() 方法在被选元素的开头插入内容。
$("p").prepend("Some prepended text.");
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
<script>
function appendText()
{
var txt1="<p>Text.</p>"; // Create text with HTML
var txt2=$("<p></p>").text("Text."); // Create text with jQuery
var txt3=document.createElement("p");
txt3.innerHTML="Text."; // Create text with DOM
$("body").append(txt1,txt2,txt3); // Append new elements
}
</script>
</head>
<body>
<p>This is a paragraph.</p>
<button onclick="appendText()">Append text</button>
</body>
</html>
jQuery after() 方法在被选元素之后插入内容。
$("img").after("Some text after");
jQuery before() 方法在被选元素之前插入内容。
$("img").before("Some text before");
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
function afterText()
{
var txt1="<b>I </b>"; // Create element with HTML
var txt2=$("<i></i>").text("love "); // Create with jQuery
var txt3=document.createElement("big"); // Create with DOM
txt3.innerHTML="jQuery!";
$("img").after(txt1,txt2,txt3); // Insert new elements after img
}
</script>
</head>
<body>
<img src="/static/images/logo.png">
<br><br>
<button onclick="afterText()">Insert after</button>
</body>
</html>
删除元素/内容
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").remove();
});
});
</script>
</head>
<body>
<div id="div1" style="height:100px;300px;border:1px solid black;">
This is some text in the div.
<p>This is a paragraph in the div.</p>
<p>This is another paragraph in the div.</p>
</div>
<br>
<button>Remove div element</button>
</body>
</html>
jQuery empty() 方法删除被选元素的子元素。
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").empty();
});
});
</script>
</head>
<body>
<div id="div1" style="height:100px;300px;border:1px solid black;">
This is some text in the div.
<p>This is a paragraph in the div.</p>
<p>This is another paragraph in the div.</p>
</div>
<br>
<button>Empty the div element</button>
</body>
</html>
过滤被删除的元素
该参数可以是任何 jQuery 选择器的语法。
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("p").remove(".italic"); //是删掉id为关键词的这一行,而不是保留这一行
});
});
</script>
</head>
<body>
<p>This is a paragraph in the div.</p>
<p class="italic"><i>This is another paragraph in the div.</i></p>
<p class="italic"><i>This is another paragraph in the div.</i></p>
<button>Remove all p elements with class="italic"</button>
</body>
</html>
2.removeClass() - 从被选元素删除一个或多个类
3.toggleClass() - 对被选元素进行添加/删除类的切换操作
4.css() - 设置或返回样式属性
jQuery addClass() 方法
下面的例子展示如何向不同的元素添加 class 属性。当然,在添加类时,您也可以选取多个元素:
$("div").addClass("important");
});
您也可以在 addClass() 方法中规定多个类:
});
jQuery removeClass() 方法
下面的例子演示如何不同的元素中删除指定的 class 属性:
$("h1,h2,p").removeClass("blue");
});
jQuery toggleClass() 方法
下面的例子将展示如何使用 jQuery toggleClass() 方法。该方法对被选元素进行添加/删除类的切换操作:
$("h1,h2,p").toggleClass("blue");
});
jQuery css() Method
css() 方法设置或返回被选元素的一个或多个样式属性。
返回 CSS 属性
如需返回指定的 CSS 属性的值,请使用如下语法:
下面的例子将返回首个匹配元素的 background-color 值:
设置 CSS 属性
如需设置指定的 CSS 属性,请使用如下语法:
下面的例子将为所有匹配元素设置 background-color 值:
设置多个 CSS 属性
如需设置多个 CSS 属性,请使用如下语法:
下面的例子将为所有匹配元素设置 background-color 和 font-size:
<head>
<script>
$(document).ready(function(){
$("button").click(function(){
$("p").css({"background-color":"yellow","font-size":"200%"});
});
});
</script>
</head>
<body>
<h2>This is a heading</h2>
<p style="This is a paragraph.</p>
<p style="This is a paragraph.</p>
<p style="This is a paragraph.</p>
<p>This is a paragraph.</p>
<button>Set multiple styles for p</button>
</body>
</html>
jQuery 尺寸
通过 jQuery,很容易处理元素和浏览器窗口的尺寸。
jQuery 提供多个处理尺寸的重要方法:
width()
height()
innerWidth()
innerHeight()
outerWidth()
outerHeight()
jQuery width() 和 height() 方法
width() 方法设置或返回元素的宽度(不包括内边距、边框或外边距)。
height() 方法设置或返回元素的高度(不包括内边距、边框或外边距)。
下面的例子返回指定的 <div> 元素的宽度和高度:
var txt="";
txt+="Width: " + $("#div1").width() + "</br>";
txt+="Height: " + $("#div1").height();
$("#div1").html(txt);
});
jQuery innerWidth() 和 innerHeight() 方法
innerWidth() 方法返回元素的宽度(包括内边距)。
innerHeight() 方法返回元素的高度(包括内边距)。
下面的例子返回指定的 <div> 元素的 inner-width/height:
var txt="";
txt+="Inner " + $("#div1").innerWidth() + "</br>";
txt+="Inner height: " + $("#div1").innerHeight();
$("#div1").html(txt);
});
jQuery outerWidth() 和 outerHeight() 方法
outerWidth() 方法返回元素的宽度(包括内边距和边框)。
outerHeight() 方法返回元素的高度(包括内边距和边框)。
下面的例子返回指定的 <div> 元素的 outer-width/height:
var txt="";
txt+="Outer " + $("#div1").outerWidth() + "</br>";
txt+="Outer height: " + $("#div1").outerHeight();
$("#div1").html(txt);
});
jQuery AJAX 简介
什么是 AJAX?
AJAX = 异步 JavaScript 和 XML(Asynchronous JavaScript and XML)。
简短地说,在不重载整个网页的情况下,AJAX 通过后台加载数据,并在网页上进行显示。
关于 jQuery 与 AJAX
jQuery 提供多个与 AJAX 有关的方法。
通过 jQuery AJAX 方法,您能够使用 HTTP Get 和 HTTP Post 从远程服务器上请求文本、HTML、XML 或 JSON - 同时您能够把这些外部数据直接载入网页的被选元素中。
jQuery load() 方法
jQuery load() 方法是简单但强大的 AJAX 方法。
load() 方法从服务器加载数据,并把返回的数据放入被选元素中。
语法:
必需的 URL 参数规定您希望加载的 URL。
可选的 data 参数规定与请求一同发送的查询字符串键/值对集合。
可选的 callback 参数是 load() 方法完成后所执行的函数名称。
这是示例文件("demo_test.txt")的内容:
<p id="p1">This is some text in a paragraph.</p>
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").load("/demo/ajax/demo_test.txt");
});
});
</script>
</head>
<body>
<div id="div1"><h2>使用 jQuery AJAX 修改文本内容</h2></div>
<button>获取外部内容</button>
</body>
</html>
也可以把 jQuery 选择器添加到 URL 参数。
下面的例子把 "demo_test.txt" 文件中 id="p1" 的元素的内容,加载到指定的 <div> 元素中:
<html><head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").load("/demo/ajax/demo_test.txt #p1");
});
});
</script>
</head>
<body>
<div id="div1"><h2>Let jQuery AJAX Change This Text</h2></div>
<button>Get External Content</button>
</body>
</html>
可选的 callback 参数规定当 load() 方法完成后所要允许的回调函数。回调函数可以设置不同的参数:
- responseTxt - 包含调用成功时的结果内容
- statusTXT - 包含调用的状态
- xhr - 包含 XMLHttpRequest 对象
下面的例子会在 load() 方法完成后显示一个提示框。如果 load() 方法已成功,则显示"外部内容加载成功!",而如果失败,则显示错误消息:
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#div1").load("/demo/ajax/demo_test.txt",function(responseTxt,statusTxt,xhr){
if(statusTxt=="success")
alert("外部内容载入成功!");
if(statusTxt=="error")
alert("Error: "+xhr.status+": "+xhr.statusText);
});
});
});
</script>
</head>
<body>
<div id="div1"><h2>使用 jQuery AJAX 修改该文本</h2></div>
<button>获取外部内容</button>
</body>
</html>
jQuery – AJAX get() 和 post() 方法
jQuery get() 和 post() 方法用于通过 HTTP GET 或 POST 请求从服务器请求数据。
HTTP 请求:GET vs. POST
两种在客户端和服务器端进行请求-响应的常用方法是:GET 和 POST。
- GET - 从指定的资源请求数据
- POST - 向指定的资源提交要处理的数据
GET 基本上用于从服务器获得(取回)数据。注释:GET 方法可能返回缓存数据。
POST 也可用于从服务器获取数据。不过,POST 方法不会缓存数据,并且常用于连同请求一起发送数据。
如需学习更多有关 GET 和 POST 以及两方法差异的知识,请阅读我们的 HTTP 方法 - GET 对比 POST。
jQuery $.get() 方法
$.get() 方法通过 HTTP GET 请求从服务器上请求数据。
语法:
必需的 URL 参数规定您希望请求的 URL。
可选的 callback 参数是请求成功后所执行的函数名。
下面的例子使用 $.get() 方法从服务器上的一个文件中取回数据:
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.get("/try/ajax/demo_test.php",function(data,status){
alert("Data: " + data + "
Status: " + status);
});
});
});
</script>
</head>
<body>
<button>Send an HTTP GET request to a page and get the result back</button>
</body>
</html>
$.get() 的第一个参数是我们希望请求的 URL("demo_test.php")。
第二个参数是回调函数。第一个回调参数存有被请求页面的内容,第二个回调参数存有请求的状态。
提示: 这个 PHP 文件 ("demo_test.php") 类似这样:
echo "This is some text from an external PHP file.";
?>
jQuery $.post() 方法
语法:
必需的 URL 参数规定您希望请求的 URL。
可选的 data 参数规定连同请求发送的数据。
可选的 callback 参数是请求成功后所执行的函数名。
下面的例子使用 $.post() 连同请求一起发送数据:
<html>
<head>
<script src="http://libs.baidu.com/jquery/1.10.2/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("button").click(function(){
$.post("/try/ajax/demo_test_post.php",
{
name:"Weizhong Tu",
city:"TianJin"
},
function(data,status){
alert("Data: " + data + "
Status: " + status);
});
});
});
</script>
</head>
<body>
<button>发送一个 HTTP POST 请求道页面并获取返回内容</button>
</body>
</html>
$.post() 的第一个参数是我们希望请求的 URL ("demo_test_post.php")。
然后我们连同请求(name 和 city)一起发送数据。
"demo_test_post.php" 中的 PHP 脚本读取这些参数,对它们进行处理,然后返回结果。
第三个参数是回调函数。第一个回调参数存有被请求页面的内容,而第二个参数存有请求的状态。
提示: 这个 PHP 文件 ("demo_test_post.php") 类似这样:
$name = isset($_POST['name']) ? htmlspecialchars($_POST['name']) : '';
$city = isset($_POST['city']) ? htmlspecialchars($_POST['city']) : '';
echo 'Dear ' . $name;
echo 'Hope you live well in ' . $city;
?>