1.Activity跳转
设置两个地方
1.1 需要在AndroidMainfest里面进行参数设置 将activity放到里面
<activity android:name=".ResultActivity"/>
1.2 在需要跳转的activity中使用意图调用目标的activity.class
Intent intent = new Intent(this, ResultActivity.class);
2.Activity间的数据传递
2.1 数据的发送
intent.putExtra("name", name);
intent.putExtra("sex", sex);
2.2 数据的接收
String name = intent.getStringExtra("name"); int sex = intent.getIntExtra("sex", 0);
下面开始放上正式的代码:
首先是AndroidMainfest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.a2_quality_caculator"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.2_quality_caculator"> <activity android:name=".MainActivity"> <!--意图过滤器--> <intent-filter> <!--定义主路口--> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <!--意图activity也就是新的页面--> <activity android:name=".ResultActivity"/> </application> </manifest>
主要页面 MainActivity.java 主要用于获得用户输入的姓名 性别,同时将数据进行发送
package com.example.a2_quality_caculator; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.text.TextUtils; import android.view.View; import android.widget.EditText; import android.widget.RadioGroup; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private EditText et_name; private RadioGroup rg_group; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); et_name = (EditText) findViewById(R.id.et_name); rg_group = (RadioGroup) findViewById(R.id.radioGroup1); } public void click(View view) { //[1] 获取用户名 String name = et_name.getText().toString().trim(); //[2] 判断name为空 if (TextUtils.isEmpty(name)) { Toast.makeText(getApplicationContext(), "请输入姓名", Toast.LENGTH_LONG).show(); return; } //[3] 判断用户选择的性别 int radioButtonId = rg_group.getCheckedRadioButtonId(); int sex = 0; switch (radioButtonId) { case R.id.rb_male: sex = 1; break; case R.id.rb_female: sex = 2; break; case R.id.rb_other: sex = 3; break; } if (sex == 0) { Toast.makeText(getApplicationContext(), "请选择性别", Toast.LENGTH_LONG).show(); return; } //[4] 进行意图跳转 Intent intent = new Intent(this, ResultActivity.class); intent.putExtra("name", name); intent.putExtra("sex", sex); startActivity(intent); } }
主要页面 activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <EditText android:id="@+id/et_name" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="请输入姓名" /> <RadioGroup android:id="@+id/radioGroup1" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <RadioButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="男" android:layout_marginLeft="25dp" android:id="@+id/rb_male"/> <RadioButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:checked="true" android:text="女" android:layout_marginLeft="25dp" android:id="@+id/rb_female"/> <RadioButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="人妖" android:layout_marginLeft="25dp" android:id="@+id/rb_other"/> </RadioGroup> <Button android:onClick="click" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="计算" /> </LinearLayout>
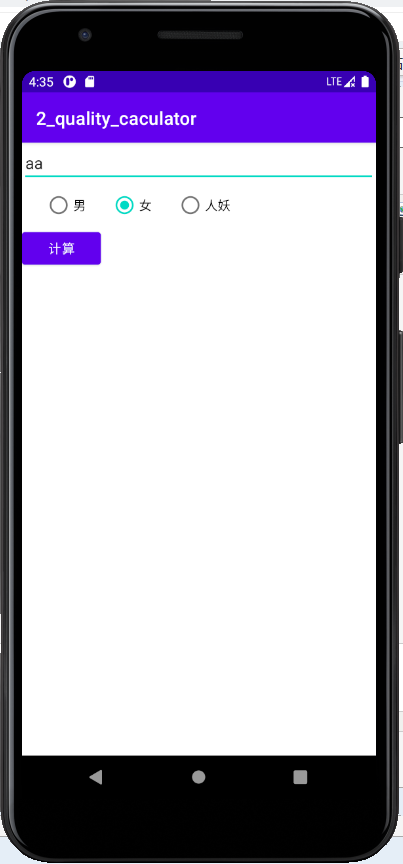
调转页面 主要用于接收数据,显示数据的结果和根据姓名来计算人品
ReusltActivity.java 主要用于接收数据,显示数据 和 人品预测
package com.example.a2_quality_caculator; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.widget.TextView; import java.io.UnsupportedEncodingException; public class ResultActivity extends Activity { protected void onCreate(Bundle savedInstancestate) { super.onCreate(savedInstancestate); //[1] 加载布局 setContentView(R.layout.activity_result); //获得对象 TextView tv_name = findViewById(R.id.tv_name); TextView tv_sex = findViewById(R.id.tv_sex); TextView tv_result = findViewById(R.id.tv_result); //[2]获取mainActivity传递过来的数据 Intent intent = getIntent(); //[3]获取name和sex的数据 String name = intent.getStringExtra("name"); int sex = intent.getIntExtra("sex", 0); //[4]显示name的数据 tv_name.setText(name); byte[] bytes = null; System.out.println("sex" + sex); //[5] 显示性别 try { switch (sex) { case 1: tv_sex.setText("男"); bytes = name.getBytes("gbk"); break; case 2: tv_sex.setText("女"); bytes = name.getBytes("utf-8"); break; case 3: tv_sex.setText("人妖"); bytes = name.getBytes("ISO-8859-1"); break; } }catch (UnsupportedEncodingException e) { e.printStackTrace(); } //[6]计算人品值 int total = 0; for (byte b: bytes) { int number = b&0xff; total += number; } //获取得分 int score = Math.abs(total) % 100; //[7]显示结果 if (score > 90) { tv_result.setText("您的人品非常好"); }else if (score > 80) { tv_result.setText("您的人品还可以"); }else if (score > 60) { tv_result.setText("您的人品刚好及格"); }else{ tv_result.setText("您的人品太差了"); } } }
跳转界面显示 activity_result.xml