一、Lock 接口相关 API
Java 8 之前使用 synchronized,之后用 lock 锁。
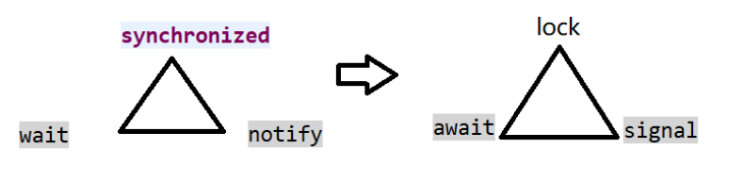
二、Lock 接口实现线程通信
要求:有两个线程,实现对一个初始值是 0 的变量进行操作,一个线程对当前数值加 1,另一个线程对当前数值减 1,这两个线程交替完成效果,要求用线程间通信。
实现:
//第一步 创建资源类,定义属性和操作方法
class Share {
private int number = 0;
//创建 Lock
private Lock lock = new ReentrantLock();
private Condition condition = lock.newCondition();
//+1
public void incr() throws InterruptedException {
//上锁
lock.lock();
try {
//1. 判断
while (number != 0) {
condition.await();
}
//2. 干活
number++;
System.out.println(Thread.currentThread().getName() + "::" + number);
//3. 通知
condition.signalAll();
} finally {
//解锁
lock.unlock();
}
}
//-1
public void decr() throws InterruptedException {
//上锁
lock.lock();
try {
//1. 判断
while (number != 1) {
condition.await();
}
//2. 干活
number--;
System.out.println(Thread.currentThread().getName() + "::" + number);
//3. 通知
condition.signalAll();
} finally {
//解锁
lock.unlock();
}
}
}
public class ThreadDemo2 {
public static void main(String[] args) {
//第三步 创建多个线程,调用资源类的操作方法;
Share share = new Share();
//创建线程
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
share.incr();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "AA").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
share.decr();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "BB").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
share.incr();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "CC").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
share.decr();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "DD").start();
}
}