Problem Description
Five hundred years later, the number of dragon balls will increase unexpectedly, so it's too difficult for Monkey King(WuKong) to gather all of the dragon balls together.
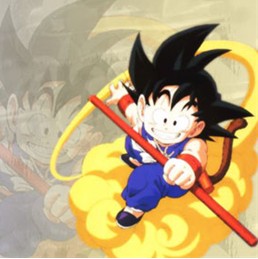
His country has N cities and there are exactly N dragon balls in the world. At first, for the ith dragon ball, the sacred dragon will puts it in the ith city. Through long years, some cities' dragon ball(s) would be transported to other cities. To save physical strength WuKong plans to take Flying Nimbus Cloud, a magical flying cloud to gather dragon balls.
Every time WuKong will collect the information of one dragon ball, he will ask you the information of that ball. You must tell him which city the ball is located and how many dragon balls are there in that city, you also need to tell him how many times the ball has been transported so far.
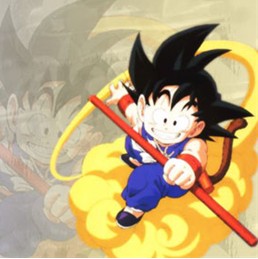
His country has N cities and there are exactly N dragon balls in the world. At first, for the ith dragon ball, the sacred dragon will puts it in the ith city. Through long years, some cities' dragon ball(s) would be transported to other cities. To save physical strength WuKong plans to take Flying Nimbus Cloud, a magical flying cloud to gather dragon balls.
Every time WuKong will collect the information of one dragon ball, he will ask you the information of that ball. You must tell him which city the ball is located and how many dragon balls are there in that city, you also need to tell him how many times the ball has been transported so far.
Input
The first line of the input is a single positive integer T(0 < T <= 100).
For each case, the first line contains two integers: N and Q (2 < N <= 10000 , 2 < Q <= 10000).
Each of the following Q lines contains either a fact or a question as the follow format:
T A B : All the dragon balls which are in the same city with A have been transported to the city the Bth ball in. You can assume that the two cities are different.
Q A : WuKong want to know X (the id of the city Ath ball is in), Y (the count of balls in Xth city) and Z (the tranporting times of the Ath ball). (1 <= A, B <= N)
For each case, the first line contains two integers: N and Q (2 < N <= 10000 , 2 < Q <= 10000).
Each of the following Q lines contains either a fact or a question as the follow format:
T A B : All the dragon balls which are in the same city with A have been transported to the city the Bth ball in. You can assume that the two cities are different.
Q A : WuKong want to know X (the id of the city Ath ball is in), Y (the count of balls in Xth city) and Z (the tranporting times of the Ath ball). (1 <= A, B <= N)
Output
For each test case, output the test case number formated as sample output. Then for each query, output a line with three integers X Y Z saparated by a blank space.
Sample Input
2
3 3
T 1 2
T 3 2
Q 2
3 4
T 1 2
Q 1
T 1 3
Q 1
Sample Output
Case 1:
2 3 0
Case 2:
2 2 1
3 3 2
这道题还是一道很经典的并查集。
只怪自己太粗心,题目意思看错了。
题目意思是:T A B意思是把A球所在城市的所有球传送到B球所在的城市。
隐藏意思是:也就是说每一个城市都只被传送了一次,因为当A球所在城市的所有球都到了B球所在城市的时候,那么之前A球所在的城市的就没有球了,那么那个B球肯定不会再出现在之前A球所在的城市。
那么Q A意思是:将A球所在的城市编号输出来,这一步其实很简单,只要用f[]数组记录一下,用find()函数查找根节点就能实现。
将A球所在城市的所有球的数量输出来,其实也很简单,另外开一个count[]数组,当需要合并的时候,将A球所在城市的球数加到B球所在城市,即:count[yy]+=count[xx];
至于A球移动的次数,如果题目意思没理解错,这个也是很容易求得,因为每一个城市最多被移动一次,那么要得到球被移动的次数其实就是它自己本身移动的次数加上其所有父亲移动的次数,举例说明:当1——》2,2——》3,当1——》2的时候,1球本身移动了一次,2——》3的时候,1的父亲2也移动了一次,所以1的移动次数就是sum[k]+=sum[f[k]];
下面是实现代码:
View Code

1 #include <iostream> 2 #include <stdio.h> 3 #include <string.h> 4 5 using namespace std; 6 int n,q; 7 int f[11111],count[11111],sum[11111]; 8 void init() 9 { 10 int i; 11 for(i=1;i<=n;i++) 12 { 13 f[i]=i; 14 count[i]=1; 15 sum[i]=0; 16 } 17 } 18 int find(int k) 19 { 20 if(f[k]!=k) 21 { 22 int t=find(f[k]); 23 sum[k]+=sum[f[k]]; 24 f[k]=t; 25 } 26 return f[k]; 27 } 28 void Union(int x,int y) 29 { 30 int xx=find(x); 31 int yy=find(y); 32 if(xx!=yy) 33 { 34 f[xx]=yy; 35 count[yy]+=count[xx]; 36 sum[xx]=1; 37 } 38 } 39 int main() 40 { 41 int t; 42 scanf("%d",&t); 43 int cnt=1; 44 while(t--) 45 { 46 scanf("%d%d",&n,&q); 47 printf("Case %d:\n",cnt++); 48 init(); 49 while(q--) 50 { 51 char op[2]; 52 scanf("%s",op); 53 if(op[0]=='T') 54 { 55 int a,b; 56 scanf("%d%d",&a,&b); 57 Union(a,b); 58 } 59 else 60 { 61 int pos; 62 scanf("%d",&pos); 63 int res=find(pos); 64 printf("%d %d %d\n",res,count[res],sum[pos]); 65 } 66 } 67 } 68 return 0; 69 }