Description
Bob enjoys playing computer games, especially strategic games, but sometimes he cannot find the solution fast enough and then he is very sad. Now he has the following problem. He must defend a medieval city, the roads of which form a tree. He has to put the minimum number of soldiers on the nodes so that they can observe all the edges. Can you help him?
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
For example for the tree: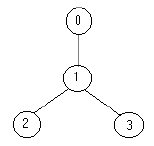
the solution is one soldier ( at the node 1).
Your program should find the minimum number of soldiers that Bob has to put for a given tree.
For example for the tree:
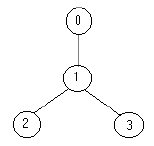
Input
The input contains several data sets in text format. Each data set represents a tree with the following description:
- the number of nodes
- the description of each node in the following format node_identifier:(number_of_roads) node_identifier1 node_identifier2 ... node_identifiernumber_of_roads or node_identifier:(0)
Output
The output should be printed on the standard output. For each given input data set, print one integer number in a single line that gives the result (the minimum number of soldiers). An example is given in the following:
题目大意:给一棵树,求最小顶点覆盖(即用最小的点覆盖所有的边)。
思路:DP靠边。树肯定是二分图无误,在二分图中,最小顶点覆盖=最大匹配,直接匈牙利跑出答案。
代码(375MS):

1 #include <cstdio> 2 #include <cstring> 3 #include <iostream> 4 #include <algorithm> 5 using namespace std; 6 7 const int MAXN = 1510; 8 const int MAXE = MAXN << 1; 9 10 int head[MAXN]; 11 int to[MAXE], next[MAXE]; 12 int n, ecnt; 13 14 void init() { 15 memset(head, 0, sizeof(head)); 16 ecnt = 2; 17 } 18 19 void add_edge(int u, int v) { 20 to[ecnt] = v; next[ecnt] = head[u]; head[u] = ecnt++; 21 to[ecnt] = u; next[ecnt] = head[v]; head[v] = ecnt++; 22 } 23 24 int link[MAXN], dep[MAXN]; 25 bool vis[MAXN]; 26 27 bool dfs(int u) { 28 for(int p = head[u]; p; p = next[p]) { 29 int &v = to[p]; 30 if(vis[v]) continue; 31 vis[v] = true; 32 if(!~link[v] || dfs(link[v])) { 33 link[v] = u; 34 return true; 35 } 36 } 37 return false; 38 } 39 40 int main() { 41 while(scanf("%d", &n) != EOF) { 42 init(); 43 memset(dep, 0, sizeof(dep)); 44 for(int i = 0; i < n; ++i) { 45 int a, b, c; 46 scanf("%d:(%d)", &a, &b); 47 while(b--) { 48 scanf("%d", &c); 49 dep[c] = dep[a] + 1; 50 add_edge(a, c); 51 } 52 } 53 int ans = 0; 54 memset(link, 255, sizeof(link)); 55 for(int i = 0; i < n; ++i) { 56 if(dep[i] & 1) continue; 57 memset(vis, 0, sizeof(vis)); 58 if(dfs(i)) ++ans; 59 } 60 printf("%d ", ans); 61 } 62 }