1. history 是什么?
window上的一个对象,由来存储浏览器访问过的历史
2. 用途:
可以动态跳转任意一个已在历史记录中的地址
3..history方法:
1.forward() : 向后翻一页
2. back(): 回退一页
3. go(num) : num为负值时 表示回退 num为正值时表示前进
4. pushState(data, title, url): 添加一条历史记录,受同源策略限制,添加历史纪录后页面地址改变但是页面不会更新——H5新增的方法 IE9以下不兼容 5. replaceState(data, title, url) 替换当前的历史记录,受同源策略限制,添加历史纪录后页面地址改变但是页面不会更新 —— H5新增的方法 IE9以下不兼容 4. history事件:
1.popstate: 每次地址改变时触发,注:pushSate和replaceState的时候不会触发该事件。只有当用户点击回退或前进按钮或者调用back , forward, go方法才会触发这个事件
2.hashchange: 地址栏中hash值改变的时候会触发
data:一个与指定网址相关的状态对象,popstate事件触发时,该对象会传入回调函数中。如果不需要这个对象,此处可以填null。 title:新页面的标题,但是所有浏览器目前都忽略这个值,因此这里可以填null。 url:新的网址,必须与当前页面处在同一个域。浏览器的地址栏将显示这个网址。
案例1:
history.forward() //前进一页
history.back() //回退一页
history,go(2) // 前进两页
history.go(-2) // 回退两页
console.log(history.length) // 打印挡墙历史记录的条数
案例2: 以下代码在服务器环境下
假设当前网址为http://localhost/index.html 使用pushState在历史记录里面添加一条历史记录
history.pushState({
page: 'page1'
}, 'page-1', './page.html');
打开当前的地址,会发现页面里面显示的内容为index.html里面的内容但是地址变为http://localhost/page.html ,这是因为pushState是向历史记录添加一条记录但是不会刷新页面。点击回退按钮地址栏会变化为index.html
即: pushState方法不会触发页面刷新,只是导致history对象发生变化,地址栏的显示地址发生变化
案例3:
假设当前网址为http://localhost/index.html 使用replaceState在历史记录里面替换一条历史记录
history.pushState({
page: 'page1'
}, 'page-1', './page.html');
打开当前的地址,会发现页面里面显示的内容为index.html里面的内容但是地址变为http://localhost/page.html ,点击回退按钮不会跳转到index.html页面,这是因为replaceState是在历史记录里面替换当前的记录,页面仍然不会刷新。
即: replaceState方法不会触发页面刷新,只是导致history对象发生变化,地址栏的显示地址发生变化
案例4:
假设当前网址为http://localhost/index.html
1 history.pushState({ 2 num: 1 3 }, 'title-history', '?num=1'); 4 history.pushState({ 5 num:2 6 }, 'title-history', '?num=2'); 7 history.pushState({ 8 num: 3 9 }, 'title-history', '?num=3'); 10 history.replaceState({ 11 num: 4 12 }, 'title-history', '?num=4'); 13 window.addEventListener('popstate', function (e) { 14 //打印当前页面的数据(状态信息) 15 console.log(e.state); 16 console.log(history.state) 17 }, false) 18 19 // 当前页面地址为 http://localhost/index.html?num=4 以下代码均在浏览器控制台里面触发 20 // history.back() // 当前页面地址为 http://localhost/index.html?num=2 21 // history.forward() // 当前页面地址为 http://localhost/index.html?num=4' 22 // history.go(-2) // 当前页面地址为 http://localhost/index.html?num=1
案例4: 实现过滤效果
初始效果:
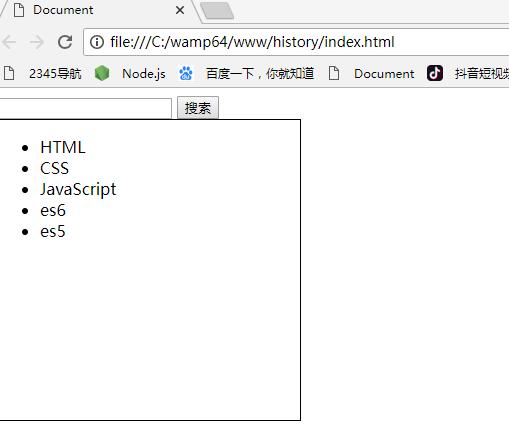
搜索关键词后的效果:
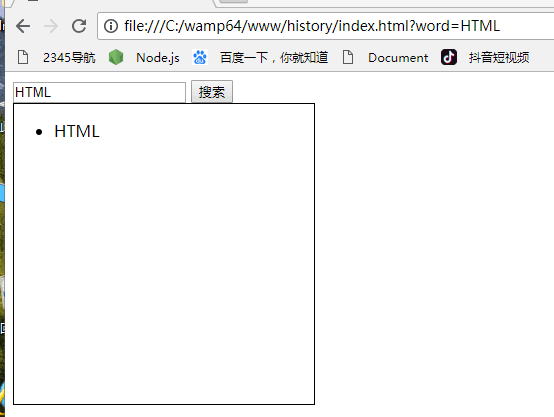
点击回退按钮之后的效果
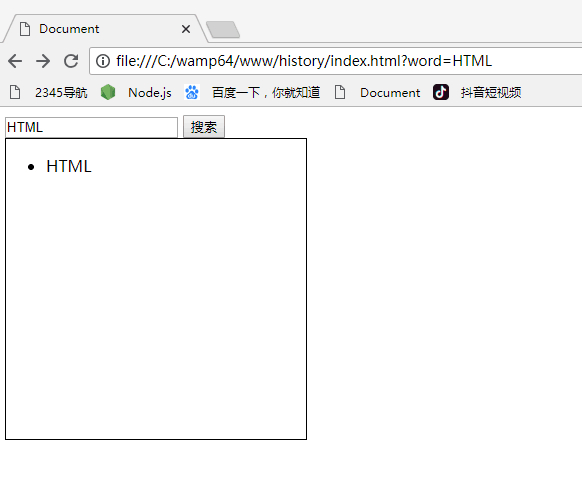
1 代码: 2 <!DOCTYPE html> 3 <html lang="en"> 4 <head> 5 <meta charset="UTF-8"> 6 <meta name="viewport" content="width=device-width, initial-scale=1.0"> 7 <meta http-equiv="X-UA-Compatible" content="ie=edge"> 8 <title>Document</title> 9 <style> 10 .content { 11 300px; 12 height: 300px; 13 border: 1px solid #000; 14 } 15 </style> 16 </head> 17 <body> 18 <input type="text" id="words"> 19 <input type="button" value="搜索" id="btn"> 20 <div class="content"> 21 <ul id="showData"> 22 </ul> 23 </div> 24 <script> 25 var words = document.getElementById('words'); 26 var data = [{ 27 name: 'HTML', 28 id: 'html' 29 }, { 30 name: 'CSS', 31 id: 'css' 32 }, { 33 name: 'JavaScript', 34 id: 'js' 35 }, { 36 name: 'es6', 37 id: 'es6' 38 }, { 39 name: 'es5', 40 id: 'es5', 41 }]; 42 function renderDom(data) { 43 var str = ''; 44 data.forEach(function (item, index) { 45 str += '<li>' + item.name + '</li>'; 46 }); 47 showData.innerHTML = str; 48 } 49 renderDom(data); 50 btn.onclick = function (e) { 51 var filterData = data.filter(function (item, index) { 52 return item.name.indexOf(words.value) > -1; 53 }); 54 history.pushState({ 55 word: words.value 56 }, null, '?word=' + words.value); 57 renderDom(filterData); 58 } 59 window.onpopstate = function (e) { 60 console.log(e); 61 var key = e.state ? e.state.word : ''; 62 var filterData = data.filter(function (item, index) { 63 return item.name.indexOf(key) > -1; 64 }); 65 renderDom(filterData); 66 words.value = key; 67 } 68 </script> 69 </body> 70 </html>
欢迎加入web前端冲击顶级高薪大厂学习群,群聊号码:820269529