一、登录界面及更改密码界面
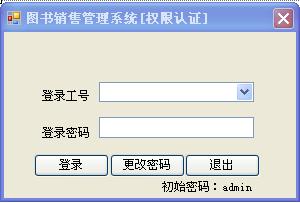

二、创建存储过程
1、pr_login
CREATE proc pr_login
@id varchar(10), --输入参数
@pwd varchar(10), --输入参数
@result varchar(10) output --输出参数
as
select * from login where 登录工号=@id
if(@@rowcount<1)
begin
set @result='没有此工号'
end
else
begin
select * from login where (登录工号=@id)and(登录密码=@pwd)
if(@@rowcount<1)
begin
set @result='密码错误'
end
else
begin
set @result='ACCESS'
end
end
return
2、pr_OperateLogin
CREATE proc pr_OperateLogin
@id varchar(10),
@pwd varchar(10),
@filter char(10)
as
if(@filter='普通查询')
select 登录工号 from login --用来加载工号
else if(@filter='按工号查询')
select 登录工号,登录密码 from login where 登录工号=@id --用来判断密码输入的正确与否
else if(@filter='更新密码') --更改密码
update login set 登录密码=@pwd where 登录工号=@id
return
三、创建类LinkDB.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
using System.Data.SqlTypes;

namespace BookSale


{
class LinkDB

{
SqlConnection conn = new SqlConnection("server=localhost;integrated security=sspi;database=library"); //连接的是本地数据库

//调用存储过程判断是否登录成功(bllogin为true表示登录成功;为false表示登录失败)
public bool Login(string strid, string strpwd)

{
bool bllogin = false;
SqlCommand cmd = new SqlCommand("pr_login",conn);
cmd.CommandType = CommandType.StoredProcedure;

cmd.Parameters.Add("@id",SqlDbType.VarChar,10).Value=strid;//输入参数
cmd.Parameters.Add("@pwd", SqlDbType.VarChar, 10).Value = strpwd;//输入参数

SqlParameter ParameterResult = new SqlParameter("@result",SqlDbType.VarChar,10);
ParameterResult.Direction = ParameterDirection.Output; //输出参数
cmd.Parameters.Add(ParameterResult);

conn.Open();
cmd.ExecuteNonQuery();
conn.Close();

if (ParameterResult.Value.ToString().Trim() == "ACCESS")

{
bllogin = true;
}
if (ParameterResult.Value.ToString().Trim() == "没有此工号")

{
bllogin = false;
MessageBox.Show("输入的工号错误(工号不能为空),请输入正确的工号!","提示",MessageBoxButtons.OK,MessageBoxIcon.Information);
}
if(ParameterResult.Value.ToString().Trim()=="密码错误")

{
bllogin = false;
MessageBox.Show("输入的密码错误(密码不能为空),请重新输入!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
return bllogin;
}

//用来查询,供加载登录工号和判断密码是否正确(有返回值)
public DataTable queryLogin(string str_id,string str_pwd,string str_Filter)

{
SqlCommand cmd = new SqlCommand("pr_OperateLogin", conn);
cmd.CommandType = CommandType.StoredProcedure;

cmd.Parameters.Add("@id", SqlDbType.VarChar, 10).Value = str_id;
cmd.Parameters.Add("@pwd", SqlDbType.VarChar, 10).Value = str_pwd;
cmd.Parameters.Add("@filter",SqlDbType.Char,10).Value=str_Filter;
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
da.Fill(dt);
return dt;
}

//用来修改用户密码(无返回值)
public void updateLogin(string str_id,string str_pwd,string str_Filter)

{
SqlCommand cmd = new SqlCommand("pr_OperateLogin",conn);
cmd.CommandType = CommandType.StoredProcedure;

cmd.Parameters.Add("@id", SqlDbType.VarChar, 10).Value = str_id;
cmd.Parameters.Add("@pwd", SqlDbType.VarChar, 10).Value = str_pwd;
cmd.Parameters.Add("@filter", SqlDbType.Char, 10).Value = str_Filter;
conn.Open();
cmd.ExecuteNonQuery();
conn.Close();
}
}
}

四、loginFrm.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Text;
using System.Windows.Forms;

namespace BookSale


{
public partial class loginFrm : Form

{
public loginFrm()

{
InitializeComponent();
}
//------退出------
private void btnexit_Click(object sender, EventArgs e)

{
this.Close();
}

LinkDB linkDB = new LinkDB();
DataTable dt;
private void loginFrm_Load(object sender, EventArgs e)

{
this.AcceptButton = this.btnenter; //按Enter键对应登录键
this.CancelButton = this.btnexit;
string strid = "";
string strpwd = "";
string strFilter = "普通查询";
dt = linkDB.queryLogin(strid,strpwd,strFilter);
for (int i = 0; i < dt.Rows.Count; i++)

{
this.cmbid.Items.Add(dt.Rows[i][0]);
}
}

//------登录------
public static bool str_pass = false;
public bool blpass = false;
private void btnenter_Click(object sender, EventArgs e)

{
string str_id = this.cmbid.Text.ToString().Trim();
string str_pwd = this.txtpwd.Text.ToString().Trim();
blpass = linkDB.Login(str_id, str_pwd);
if (blpass == false)

{
this.txtpwd.Clear();
this.cmbid.Focus();
return;
}
this.Close();
}

//------更改密码------
private void btnmodify_Click(object sender, EventArgs e)

{
modifyFrm modifyForm = new modifyFrm();
modifyForm.Show();
}
}
}
五、modifyFrm.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Text;
using System.Windows.Forms;

namespace BookSale


{
public partial class modifyFrm : Form

{
public modifyFrm()

{
InitializeComponent();
}

private void btnexit_Click(object sender, EventArgs e)

{
this.Close();
}

LinkDB linkDB = new LinkDB();
DataTable dt1; //用来存放所有的登录工号
DataTable dt2; //用来存放筛选出来的登录工号、登录密码
private void modifyFrm_Load(object sender, EventArgs e)

{
Loading1();
for (int i = 0; i < dt1.Rows.Count; i++)

{
this.cmbid.Items.Add(dt1.Rows[i][0]);
}
}

//加载登录工号
protected void Loading1()

{
string strid = "";
string strpwd = "";
string strfilter1 = "普通查询";
dt1 = linkDB.queryLogin(strid, strpwd, strfilter1);
}

//加载筛选出来的登录工号、登录密码
protected void Loading2()

{
string strid = this.cmbid.Text.ToString().Trim();
string strpwd = "";
string strfilter2 = "按工号查询";
dt2 = linkDB.queryLogin(strid, strpwd, strfilter2);
}

//------更改按钮------
private void btnmodify_Click(object sender, EventArgs e)

{
string str_id = this.cmbid.Text.ToString().Trim();
if (str_id == "") //工号为空

{
MessageBox.Show("工号不为空!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.cmbid.Focus();
return;
}
if (this.cmbid.Text.ToString().Trim() != "") //工号不为空,则判断工号是否输入正确

{
Loading2();
if (dt2.Rows.Count!=0) //工号输入正确

{
if (this.txtpwd.Text.ToString().Trim() == "") //密码为空

{
MessageBox.Show("密码不能为空!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.txtpwd.Focus();
return;
}
else //密码不为空,则判断密码是否输入正确

{

if (this.txtpwd.Text.ToString().Trim() == dt2.Rows[0][1].ToString().Trim()) //密码正确

{
if (this.txtnewpwd.Text.ToString().Trim() == "" || this.txtnewpwd_again.Text.ToString().Trim() == "") //新密码和确认密码不能为空

{
MessageBox.Show("新密码和确认密码不能为空!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.txtnewpwd.Focus();
return;
}
else //新密码和确认密码都不为空,则判断新密码和确认密码是否一致

{
if (this.txtnewpwd.Text.ToString().Trim() == this.txtnewpwd_again.Text.ToString().Trim()) //新密码和确认密码一致

{
string strid = this.cmbid.Text.ToString().Trim();
string strpwd = this.txtnewpwd.Text.ToString().Trim();
string strfilter3 = "更新密码";
linkDB.updateLogin(strid, strpwd, strfilter3);
MessageBox.Show("密码修改成功!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.txtpwd.Clear();
this.txtnewpwd.Clear();
this.txtnewpwd_again.Clear();
return;
}
else //新密码和确认密码不一致

{
MessageBox.Show("2次输入的密码不一致,请重新输入新密码和确认密码!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.txtnewpwd.Clear();
this.txtnewpwd_again.Clear();
this.txtnewpwd.Focus();
return;
}
}
}
else //密码输入错误

{
MessageBox.Show("输入的密码不正确!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
this.txtpwd.Clear();
this.txtpwd.Focus();
return;
}
}
}
else //工号输入错误

{
MessageBox.Show("输入的工号错误,请输入正确的工号!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
return;
}
}
}
}
}
六、Programe.cs
using System;
using System.Collections.Generic;
using System.Windows.Forms;

namespace BookSale


{
static class Program

{

/**//// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()

{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
loginFrm loginForm = new loginFrm();
loginForm.ShowDialog();
if (loginForm.blpass==true)

{
Application.Run(new mainFrm());
}
}
}
}
七、创建数据库
use master
go
create database library
go
create table login
(登录工号 varchar(10),
登录密码 varchar(10)
)
go
insert into login values('DEMO','admin')
go
PS:以上程序写的比较粗糙,有什么不妥的地方,请大家给予指正。