Bezier参数方程
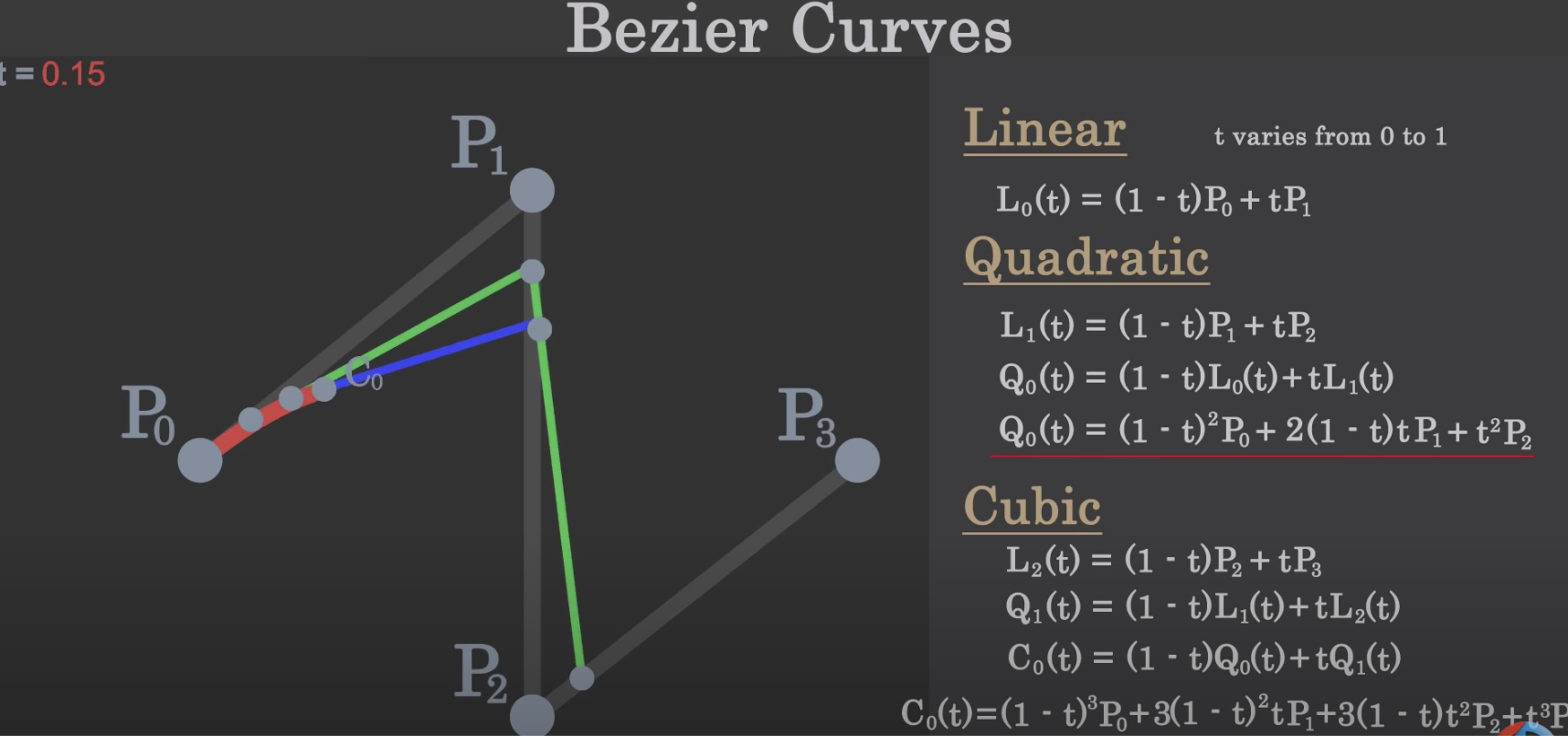
{
randomRange: function (min, max) {
return min + Math.random() * (max - min);
},
quadraticBezier: function (p0, p1, p2, t, pFinal) {
pFinal = pFinal || {};
pFinal.x = Math.pow(1 - t, 2) * p0.x +
(1 - t) * 2 * t * p1.x +
t * t * p2.x;
pFinal.y = Math.pow(1 - t, 2) * p0.y +
(1 - t) * 2 * t * p1.y +
t * t * p2.y;
return pFinal;
},
cubicBezier: function (p0, p1, p2, p3, t, pFinal) {
pFinal = pFinal || {};
pFinal.x = Math.pow(1 - t, 3) * p0.x +
Math.pow(1 - t, 2) * 3 * t * p1.x +
(1 - t) * 3 * t * t * p2.x +
t * t * t * p3.x;
pFinal.y = Math.pow(1 - t, 3) * p0.y +
Math.pow(1 - t, 2) * 3 * t * p1.y +
(1 - t) * 3 * t * t * p2.y +
t * t * t * p3.y;
return pFinal;
}
}
window.onload = function () {
let canvas = document.getElementById("canvas"),
context = canvas.getContext("2d"),
width = canvas.width = window.innerWidth,
height = canvas.height = window.innerHeight,
p0 = {
x: utils.randomRange(0, width),
y: utils.randomRange(0, height)
}
p1 = {
x: utils.randomRange(0, width),
y: utils.randomRange(0, height)
}
p2 = {
x: utils.randomRange(0, width),
y: utils.randomRange(0, height)
}
p3 = {
x: utils.randomRange(0, width),
y: utils.randomRange(0, height)
}
context.beginPath()
context.arc(p0.x, p0.y, 4, 0, Math.PI * 2, false)
context.fill()
context.beginPath()
context.arc(p1.x, p1.y, 4, 0, Math.PI * 2, false)
context.fill()
context.beginPath()
context.arc(p2.x, p2.y, 4, 0, Math.PI * 2, false)
context.fill()
context.beginPath()
context.arc(p3.x, p3.y, 4, 0, Math.PI * 2, false)
context.fill()
// context.beginPath()
// context.moveTo(p0.x,p0.y)
// context.bezierCurveTo(p1.x,p1.x,p2.x,p2.y,p3.x,p3.y)
// context.stroke()
let pFinal = {}
for(let t = 0; t <= 1; t+=0.01){
utils.cubicBezier(p0,p1,p2,p3,t,pFinal);
context.beginPath();
context.arc(pFinal.x, pFinal.y, 10, 0, Math.PI * 2, false)
context.stroke()
}
}