常用扩展方法
1 C#扩展方法
1.1 删除指定字符串
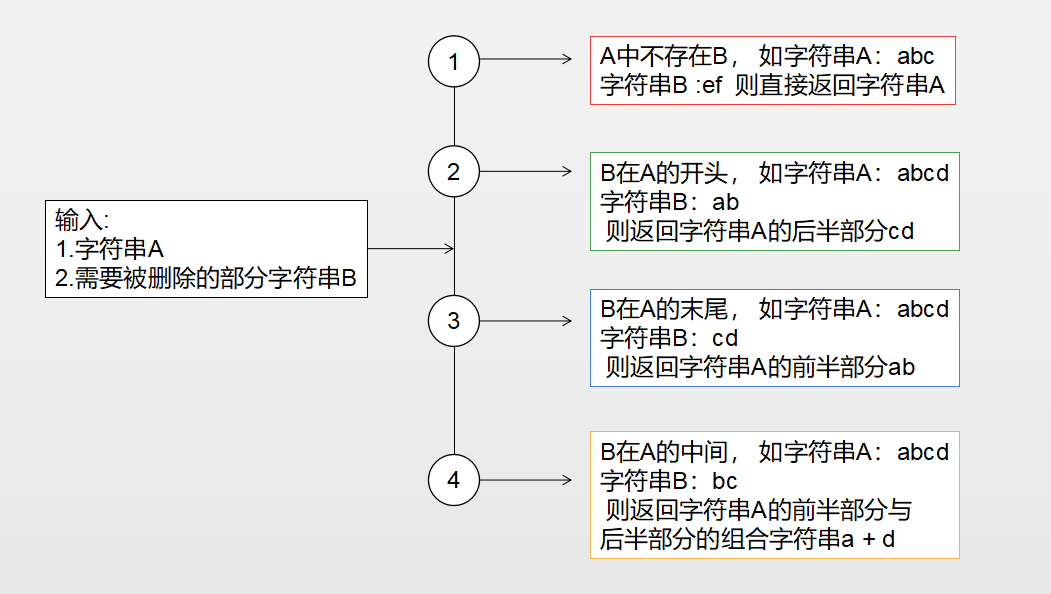
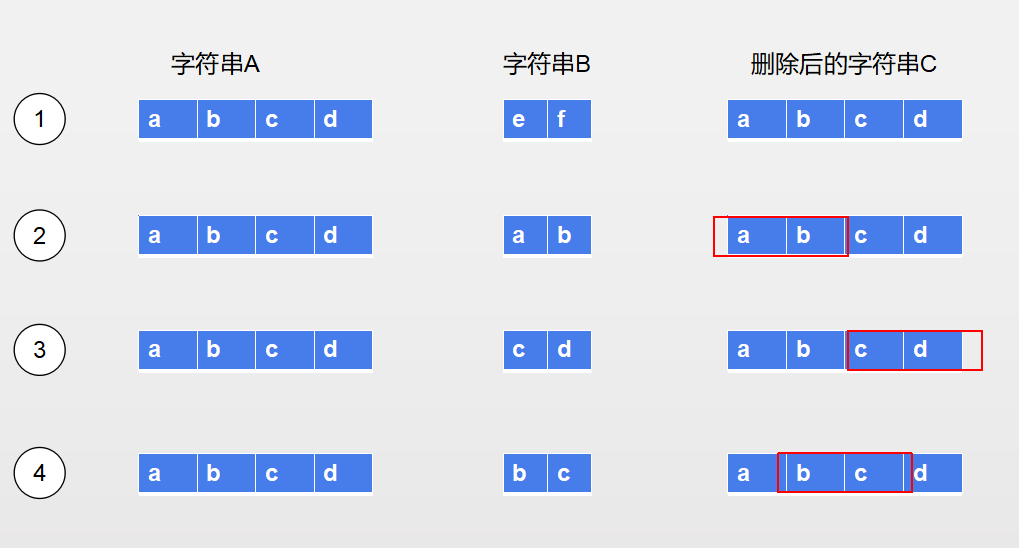
1.1.1 代码实现
`/// <summary>
/// 删除指定字符串
/// </summary>
public static string RemoveString(this string @this, string removeStr)
{
// 查找被删除的字符串的位置
int num = @this.IndexOf(removeStr);
// 1 被删除的字符串不存在
if (num == -1)
return @this;
// 2 被删除的字符串在开头
if (num == 0)
return @this.Substring(removeStr.Length);
// 3 被删除的字符串在末尾
if (@this.Length == removeStr.Length + num)
return @this.Substring(0, num);
// 4 被删除的字符串在中间
return @this.Substring(0, num) + @this.Substring(num + removeStr.Length);
}`
1.1.2 删除所有字符串扩展
`/// <summary>
/// 删除所有指定字符串
/// </summary>
public static string RemoveAllString(this string @this, string removeAllStr)
{
string removeStr = RemoveString(@this, removeAllStr);
// 查找有没有删除完所有的字符串
if (removeStr.IndexOf(removeAllStr) == -1)
return removeStr;
return RemoveAllString(removeStr, removeAllStr);
}`
1.2 比较所有字符串
/// <summary>
/// 比较所有字符串
/// </summary>
public static bool EqualsAny(this string @this, StringComparison comparisonType, params string[] values)
{
return values.Any(v => @this.Equals(v, comparisonType));
}
/// <summary>
/// https://docs.microsoft.com/zh-cn/dotnet/api/system.stringcomparison?redirectedfrom=MSDN&view=netframework-4.7.2
/// </summary>
public static bool EqualsAny(this string @this, params string[] values)
{
return EqualsAny(@this, StringComparison.CurrentCulture, values);
}
1.3 确定字符串是否包含任何提供的值
/// <summary>
/// 确定字符串是否包含任何提供的值
/// </summary>
public static bool ContainsAny(this string @this, params string[] values)
{
return @this.ContainsAny(StringComparison.CurrentCulture, values);
}
/// <summary>
/// 确定字符串是否包含任何提供的值
/// </summary>
public static bool ContainsAny(this string @this, StringComparison comparisonType, params string[] values)
{
return values.Any(v => @this.IndexOf(v, comparisonType) > -1);
}
1.4 随机数扩展
`public static class RandomExtensions{
/// <summary>
/// 随机获取项
/// </summary>
public static T GetRandItem<T>(this T[] source)
{
T[] list = ShuffleSort(source);
System.Random rnd = NewRandom();
int index = rnd.Next(0, list.Length);
return list[index];
}
/// <summary>
/// 随机获取字符串中的字符
/// </summary>
public static char GetRandItem(this string source)
{
char[] list = source.ToCharArray();
return GetRandItem<char>(list);
}
/// <summary>
/// 随机返回一个0 - 1的数
/// </summary>
public static double GetRandItemInRangeDouble()
{
System.Random random = NewRandom();
return random.NextDouble();
}
/// <summary>
/// 洗牌算法随机排序
/// </summary>
public static T[] ShuffleSort<T>(T[] source)
{
System.Random random = NewRandom();
int var;
T temp;
int len = source.Length;
for (int i = 0; i < len; ++i)
{
var = random.Next(0, len);
temp = source[i];
source[i] = source[var];
source[var] = temp;
}
return source;
}
/// <summary>
/// 随机数种子
/// </summary>
private static int GetRandomSeed()
{
byte[] bytes = new byte[4];
System.Security.Cryptography.RNGCryptoServiceProvider rng = new System.Security.Cryptography.RNGCryptoServiceProvider();
rng.GetBytes(bytes);
return BitConverter.ToInt32(bytes, 0);
}
private static System.Random NewRandom()
{
return new System.Random(GetRandomSeed());
}
}`
2 unity方法扩展
2.1 Vector3扩展
`public static class Vector3Extensions{
public static Vector3 CalculateMedian(this Vector3 @vector3, Vector3 other)
{
return new Vector3(vector3.x + other.x, vector3.y + other.y, vector3.z + other.z) * 0.5f;
}
public static Vector3 SetX(this Vector3 @vector3, float x)
{
return new Vector3(x, vector3.y, vector3.z);
}
public static Vector3 SetY(this Vector3 @vector3, float y)
{
return new Vector3(vector3.x, y, vector3.z);
}
public static Vector3 SetZ(this Vector3 @vector3, float z)
{
return new Vector3(vector3.x, vector3.y, z);
}
}`
`public static class TransformExtensions{
public static void SetX(this Transform @transform, float x)
{
@transform.position = new Vector3(x, @transform.position.y, @transform.position.z);
}
public static void SetY(this Transform @transform, float y)
{
@transform.position = new Vector3(@transform.position.x, y, @transform.position.z);
}
public static void SetZ(this Transform @transform, float z)
{
@transform.position = new Vector3(@transform.position.x, @transform.position.y, z);
}
public static void RestartTransform(this Transform @transform)
{
@transform.position = Vector3.zero;
@transform.localRotation = Quaternion.identity;
@transform.localScale = Vector3.one;
}
public static void ResetChildTransform(this Transform @transform, bool recursive = false)
{
foreach (Transform child in transform)
{
child.RestartTransform();
if (recursive)
{
child.ResetChildTransform(recursive);
}
}
}
public static void AddChildrens(this Transform @transform, params GameObject[] childrens)
{
Array.ForEach(childrens, child => child.transform.parent = transform);
}
public static T Position<T>(this T @this, Vector3 position) where T : Component
{
@this.transform.position = position;
return @this;
}
public static T LocalScale<T>(this T @this, Vector3 localPosition) where T : Component
{
@this.transform.localPosition = localPosition;
return @this;
}
public static T LocalScale<T>(this T @this, float size) where T : Component
{
@this.transform.localPosition = Vector3.one * size;
return @this;
}
public static T Rotation<T>(this T @this, Quaternion rotation) where T : Component
{
@this.transform.rotation = rotation;
return @this;
}
public static T LocalRotation<T>(this T @this, Quaternion localRotation) where T : Component
{
@this.transform.localRotation = localRotation;
return @this;
}
public static T[] GetComponentsInRealChildren<T>(this GameObject @this, bool isGrandsonObj = false)
{
T[] childrens = @this.GetComponentsInChildren<T>(isGrandsonObj);
List<T> list = new List<T>(childrens);
list.RemoveAt(0);
return list.ToArray();
}
public static T[] GetComponentsInRealChildren<T>(this Transform @this, bool isGrandsonObj = false)
{
T[] childrens = @this.GetComponentsInChildren<T>(isGrandsonObj);
List<T> list = new List<T>(childrens);
list.RemoveAt(0);
return list.ToArray();
}
public static Transform[] GetRealChildren(this Transform @this)
{
List<Transform> list = new List<Transform>();
foreach(Transform child in @this)
{
list.Add(child);
}
return list.ToArray();
}
public static GameObject[] GetRealChildren(this GameObject @this)
{
List<GameObject> list = new List<GameObject>();
foreach (Transform child in @this.transform)
{
list.Add(child.gameObject);
}
return list.ToArray();
}
public static GameObject[] ConvertGameObjects(this Transform[] @this)
{
int len = @this.Length;
GameObject[] objs = new GameObject[len];
for(int i = 0; i < len; i++)
{
objs[i] = @this[i].gameObject;
}
return objs;
}
}`