在 OOPL 中,有静态方法、实例方法和虚方法,如下:
public sealed class String
{
public static bool IsNullOrEmpty(string s)
{
// ...
}
public string Replace(string old, string new)
{
// ...
}
}
public abstract class Stream
{
public virtual void WriteByte(byte value)
{
// ...
}
}
其中,IsNullOrEmpty 是静态方法,Replace 是实例方法,而 WriteByte 就是虚方法。其用法如下:
String name = null;
Console.WriteLine(String.IsNullOrEmpty(name)); // true
String s = "Hello, world!";
Console.WriteLine(s.Replace("world", "C#")); // Hello, C#!
虚方法需要在导出类(derived class)中重新定义,此处不赘。
而在 C# 3.0 中,又引入了一种方法,称为 扩展方法(Extension Method):
扩展方法是用新方法来扩展原有类型,而不用修改原有类型的定义。
扩展原有类型(Extending the existing type)
扩展原有类型的机制(手段)有类的继承,还有 C# 中的扩展方法。
类的继承通过增加新成员,可以扩展原有类型的数据成员(fields);可以定义新方法或重写被继承类的虚方法来扩展原有类型的方法成员(methods)。但继承机制也存在自己的问题,如果原有类型声明为封闭类型(sealed class),则无法使用继承机制扩展原有类型(如上述的 String class)。再者,导出类(derived class)对基类(base class)的扩展是作用在导出类上的,而非对基类自身的扩展。
扩展方法弥补了继承机制的不足。严格说来,扩展方法不是语言本身的机制,而是通过编译程序实现的语法便用设施。不管原有类型是封闭类型还是开放类型,都可以用扩展方法进行扩展。扩展方法只是扩展原有类的方法成员,并不能扩展原有类的数据成员。
An extension method is a static method of a static class, where the this modifier is applied to the first parameter. The type of the first parameter will be the type that is extended.
扩展方法是静态类的静态方法,其第一个参数要用 this 修饰,而这第一个参数的类型就是要扩展的类型。
例子:
public static class StringHelper
{
public static bool IsCapitalized(this string s)
{
if (string.IsNullOrEmpty(s)) return false;
return char.IsUpper(s[0]);
}
}
IsCapitalized 就是定义在静态类上的静态方法,而其第一个参数 string s 用 this 修饰,所以 IsCapitalized 是扩展方法,因为第一个参数的类型是 string,所以 IsCapitalized 扩展的是 string 类型。
用法:
Console.WriteLine("Perth".IsCapitalized());
"Perth".IsCapitalized() 是直接针对 string "Perth" 调用 IsCapitalized(),因为 IsCapitalized() 是 string 的扩展方法,所以这种调用是可以的。
扩展方法的调用经过编译器的解析后,就翻译为普通的静态方法调用,即:
Console.WriteLine(StringHelper.IsCapitalized("Perth"));
而 "Perth".IsCapitalized() 相对于 StringHelper.IsCapitalized("Perth") 而言,更为自然、简洁。
所以,扩展方法仍然是一种静态方法,只是 C# 语言引入的语法便用设施而已。编译器的翻译过程如下:
arg0.Method(arg1, arg2, ...); ==> StaticClass.Method(arg0, arg1, arg2, ...);
也就是将扩展方法调用翻译为普通的静态方法调用。
扩展方法的应用
string 类虽然提供了功能强大的众多方法,但总是不可能满足所有需求。有些经常使用而 string 类又没有提供的功能,可以写成扩展方法,并归入代码库(Library),可以再多个项目中使用。下面是一个例子,两个有关 string 的扩展方法,放入 StringExtensions 类中:
public static class StringExtensions
{
public static List<KeyValuePair<string, string>> Listize(this string ss)
{
List<KeyValuePair<string, string>> pList = new List<KeyValuePair<string, string>>();
string[] slist = ss.Split(new char[] { ';' });
foreach (string s in slist)
{
if (string.IsNullOrEmpty(s)) continue;
string[] pair = s.Split(new char[] { ':' });
if (pair.Length != 2) continue;
pList.Add(new KeyValuePair<string, string>(pair[0], pair[1]));
}
return pList;
}
public static string MatchAndReplace(this string src, string pattern, string dst)
{
List<string> mList = new List<string>();
foreach (Match m in Regex.Matches(src, pattern))
{
mList.Add(m.Value);
}
foreach (string s in mList)
{
if (string.IsNullOrEmpty(s)) continue;
src = src.Replace(s, dst);
}
return src;
}
}
{
public static List<KeyValuePair<string, string>> Listize(this string ss)
{
List<KeyValuePair<string, string>> pList = new List<KeyValuePair<string, string>>();
string[] slist = ss.Split(new char[] { ';' });
foreach (string s in slist)
{
if (string.IsNullOrEmpty(s)) continue;
string[] pair = s.Split(new char[] { ':' });
if (pair.Length != 2) continue;
pList.Add(new KeyValuePair<string, string>(pair[0], pair[1]));
}
return pList;
}
public static string MatchAndReplace(this string src, string pattern, string dst)
{
List<string> mList = new List<string>();
foreach (Match m in Regex.Matches(src, pattern))
{
mList.Add(m.Value);
}
foreach (string s in mList)
{
if (string.IsNullOrEmpty(s)) continue;
src = src.Replace(s, dst);
}
return src;
}
}
上述两个扩展方法的用例如下:
string sql = GetSQL();
string whereTail = "";
foreach (string k in po.CustomObject.Keys)
{
if (string.IsNullOrEmpty(k)) continue;
if (string.Compare(k, "DemoZoneList", true) == 0)
{
whereTail = po.CustomObject[k].Value.Listize().BuildWhereClauseInclusive();
}
else if (string.Compare(k, "NonDemoZoneList", true) == 0)
{
whereTail = po.CustomObject[k].Value.Listize().BuildWhereClauseExclusive();
}
}
if (!string.IsNullOrEmpty(whereTail))
{
sql = sql.MatchAndReplace("1[ ]*=[ ]*1", whereTail);
}
foreach (string k in po.CustomObject.Keys)
{
if (string.IsNullOrEmpty(k)) continue;
if (string.Compare(k, "DemoZoneList", true) == 0)
{
whereTail = po.CustomObject[k].Value.Listize().BuildWhereClauseInclusive();
}
else if (string.Compare(k, "NonDemoZoneList", true) == 0)
{
whereTail = po.CustomObject[k].Value.Listize().BuildWhereClauseExclusive();
}
}
if (!string.IsNullOrEmpty(whereTail))
{
sql = sql.MatchAndReplace("1[ ]*=[ ]*1", whereTail);
}
可以看到,string 的扩展方法就像原生方法(指 String 类定义的方法)一样调用,代码看起来更加自然、简洁。
扩展方法机制是为了解决 LINQ 的问题而引入的,关于这一点,当另文别述。关于扩展方法的深入讨论,可以参阅《C# in Depth》 Third Edition。
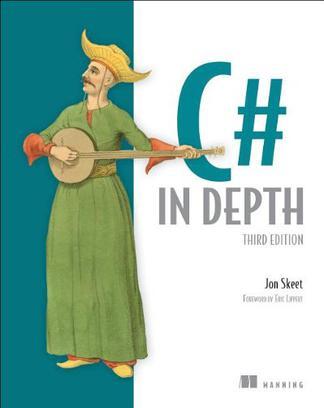