一、Struts2指定类型文件的下载
1、最终功能实现的截图:(点击文件下载链接,下载文件 )
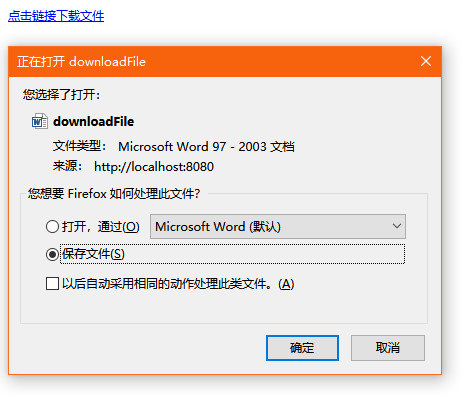
2、核心代码
index.jsp:
1 <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
2 <%
3 String path = request.getContextPath();
4 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
5 %>
6 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
7 <html>
8 <head>
9 <base href="<%=basePath%>">
10
11 <title>My JSP 'index.jsp' starting page</title>
12 <meta http-equiv="pragma" content="no-cache">
13 <meta http-equiv="cache-control" content="no-cache">
14 <meta http-equiv="expires" content="0">
15 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
16 <meta http-equiv="description" content="This is my page">
17 </head>
18 <body>
19 <a href="<%=path%>/downloadFile?download=UploadFile/readme.doc">点击链接下载文件</a>
20 </body>
21 </html>
struts.xml:
1 <?xml version="1.0" encoding="UTF-8" ?>
2 <!DOCTYPE struts PUBLIC
3 "-//Apache Software Foundation//DTD Struts Configuration 2.3//EN"
4 "http://struts.apache.org/dtds/struts-2.3.dtd">
5 <struts>
6 <package name="default" namespace="/" extends="struts-default">
7 <action name="downloadFile" class="com.thanlon.action.DownloadFileAction">
8 <result name="success" type="stream"><!-- result类型是流(stream)类型 -->
9 <param name="inputName">inputStream</param><!-- inputName指向被下载文件的来源,对应Action中返回的InputStream -->
10 <param name="contentType">${contentType}</param><!-- 下载的内容类型,如图片类型、文档类型等…… -->
11 <param name="contentDisposition">
12 <!-- 指定文件下载的处理方式,内联(inline)和附件(attachment)两种方式,attachment会弹出文件保存对话框 -->
13 attachment;filename=${filename}
14 </param>
15 </result>
16 </action>
17 </package>
18 </struts>
DownloadFileAction.java:
1 package com.thanlon.action;
2
3 import java.io.InputStream;
4
5 import org.apache.struts2.ServletActionContext;
6
7 import com.opensymphony.xwork2.Action;
8
9 public class DownloadFileAction implements Action {
10
11 private String downloadPath;// 下载的路径
12 private String contentType;// 下载文件的类型
13 private String fileName;// 文件类型
14
15 public String getDownloadPath() {
16 return downloadPath;
17 }
18
19 public void setDownloadPath(String downloadPath) {
20 this.downloadPath = downloadPath;
21 }
22
23 public String getContentType() {
24 return contentType;
25 }
26
27 public void setContentType(String contentType) {
28 this.contentType = contentType;
29 }
30
31 public String getFileName() {
32 return fileName;
33 }
34
35 public void setFileName(String fileName) {
36 this.fileName = fileName;
37 }
38
39 @Override
40 public String execute() throws Exception {
41 // TODO Auto-generated method stub
42 downloadPath = ServletActionContext.getRequest().getParameter(
43 "download");
44 System.out.println(downloadPath);// downloadPath为相对路径,打印出“/UploadFile/readme.doc”
45 int position = downloadPath.lastIndexOf("/");// 借助lastIndexOf函数,从右向左查找第一个/
46 System.out.println(position);// 打印"/"所造的坐标
47 if (position > 0) {
48 fileName = downloadPath.substring(position + 1);// 得到文件的名字(全名,带后缀)
49 System.out.println(fileName);// 輸出文件名,本例輸出readme.doc
50 } else {
51 fileName = downloadPath;
52 }
53 contentType = "application/msword";// 指定下载问文件的类型
54 return SUCCESS;
55 }
56
57 /**
58 * 返回InputStream
59 *
60 * @return
61 */
62 public InputStream getInputStream() {
63 InputStream inputStream = ServletActionContext.getServletContext()
64 .getResourceAsStream(downloadPath);
65 // System.out.println(inputStream);输出inputStream,如果为null表示路径出错
66 return inputStream;
67 }
68 }
二、Struts2多种类型(不指定)文件的下载
1、火狐浏览器下测试对比后,很明显可以看出添加资源文件后,下载时文件的类型被设置成文件真实类型。
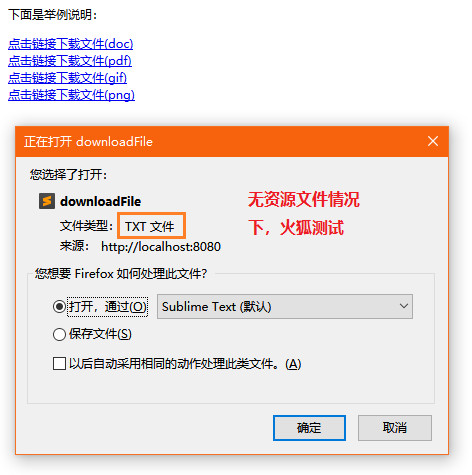
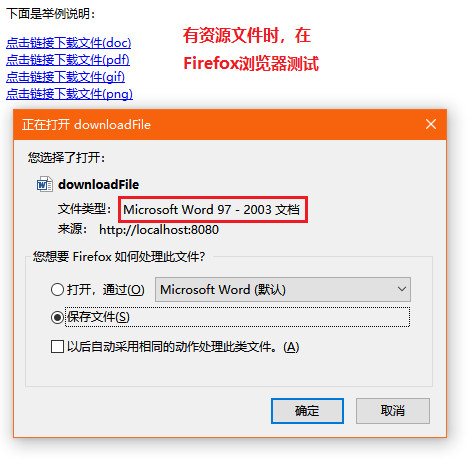
下面同样是有无资源文件的对比,也是很明显可以看出。使用资源文件的,下载文件时带上了正确的文件后缀。
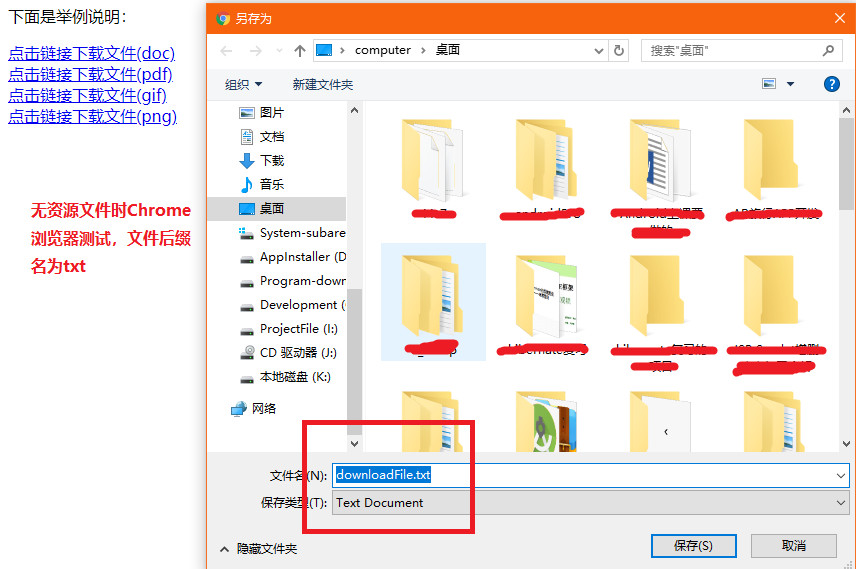
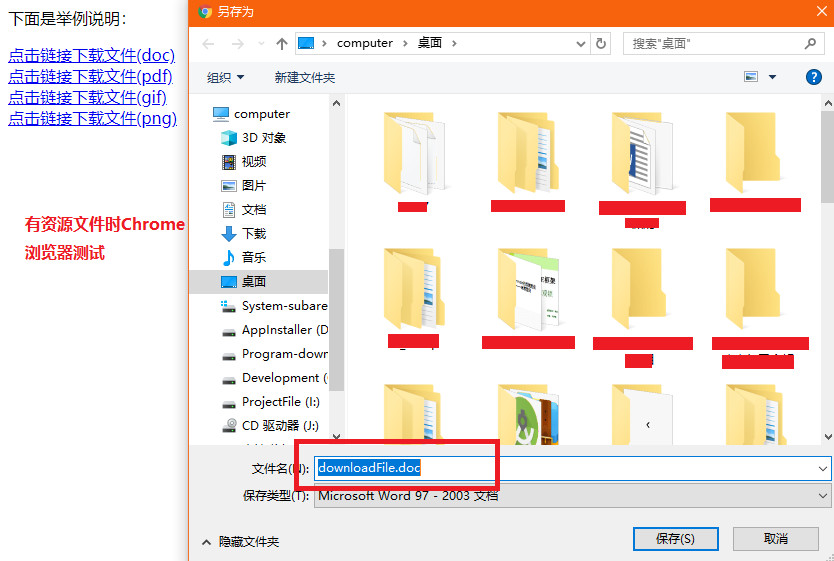
2、核心代码
index.jsp:
1 <%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
2 <%
3 String path = request.getContextPath();
4 String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
5 %>
6 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
7 <html>
8 <head>
9 <base href="<%=basePath%>">
10
11 <title>My JSP 'index.jsp' starting page</title>
12 <meta http-equiv="pragma" content="no-cache">
13 <meta http-equiv="cache-control" content="no-cache">
14 <meta http-equiv="expires" content="0">
15 <meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
16 <meta http-equiv="description" content="This is my page">
17 </head>
18 <body>
19 <p>下面是举例说明:</p>
20 <a href="<%=path%>/downloadFile?download=UploadFile/readme.doc">点击链接下载文件(doc)</a><br>
21 <a href="<%=path%>/downloadFile?download=UploadFile/thanlon.pdf">点击链接下载文件(pdf)</a><br>
22 <a href="<%=path%>/downloadFile?download=UploadFile/demo.gif">点击链接下载文件(gif)</a><br>
23 <a href="<%=path%>/downloadFile?download=UploadFile/logo.png">点击链接下载文件(png)</a>
24 </body>
25 </html>
struts.xml:
与上一例(Struts2指定类型文件的下载)的struts.xml相同
DownloadFileAction.java:
1 package com.thanlon.action;
2
3 import java.io.InputStream;
4
5 import org.apache.struts2.ServletActionContext;
6
7 import com.opensymphony.xwork2.Action;
8 import com.opensymphony.xwork2.ActionSupport;
9
10 public class DownloadFileAction extends ActionSupport {
11
12 private String downloadPath;// 下载的路径
13 private String contentType;// 下载文件的类型
14 private String fileName;// 文件类型
15
16 public String getDownloadPath() {
17 return downloadPath;
18 }
19
20 public void setDownloadPath(String downloadPath) {
21 this.downloadPath = downloadPath;
22 }
23
24 public String getContentType() {
25 return contentType;
26 }
27
28 public void setContentType(String contentType) {
29 this.contentType = contentType;
30 }
31
32 public String getFileName() {
33 return fileName;
34 }
35
36 public void setFileName(String fileName) {
37 this.fileName = fileName;
38 }
39
40 @Override
41 public String execute() throws Exception {
42 // TODO Auto-generated method stub
43 downloadPath = ServletActionContext.getRequest().getParameter(
44 "download");
45 System.out.println(downloadPath);// downloadPath为相对路径,打印出“/UploadFile/readme.doc”
46 int position = downloadPath.lastIndexOf("/");// 借助lastIndexOf函数,从右向左查找第一个/
47 // System.out.println(position);// 打印"/"所在的坐标
48 if (position > 0) {
49 fileName = downloadPath.substring(position + 1);// 得到文件的名字(全名,带后缀)
50 System.out.println(fileName);// 輸出文件名
51 } else {
52 fileName = downloadPath;
53 }
54 int extPos = fileName.lastIndexOf(".");
55 System.out.println(extPos);
56 String contentTypeKey = null;
57 if (extPos > 0) {
58 contentTypeKey = "struts.contentType" + fileName.substring(extPos);//带.的后缀
59 System.out.println(contentTypeKey);
60 }else {
61 contentTypeKey = "struts.contentType.txt";
62 //如果没有在属性文件中指定类型:如果是文件,则下载的文件默认后缀为txt文件类型;如果是图片则不会是txt类型,会是自身类型,或者特殊类型,比如jpg会是jfif类型。
63 }
64 contentType =getText(contentTypeKey);// 指定下载问文件的类型此类继承了ActionSupport,直接使用getText()函数
65 System.out.println(contentTypeKey);
66 return SUCCESS;
67 }
68 /**
69 * 返回InputStream
70 *
71 * @return
72 */
73 public InputStream getInputStream() {
74 InputStream inputStream = ServletActionContext.getServletContext()
75 .getResourceAsStream(downloadPath);
76 // System.out.println(inputStream);输出inputStream,如果为null表示路径出错
77 return inputStream;
78 }
79 }
DownloadFileAction.properties:
1 struts.contentType.gif=image/gif
2 struts.contentType.jpg=image/jpg
3 struts.contentType.jpeg=image/jpeg
4 struts.contentType.bmp=image/bmp
5 struts.contentType.png=image/png
6 struts.contentType.txt=text/plain
7 struts.contentType.swf=application/x-shockwave-flash
8 struts.contentType.doc=application/msword
9 struts.contentType.xls=application/vnd.ms-excel
10 struts.contentType.pdf=application/pdf
11 struts.contentType.ppt=application/vnd.ms-powerpoint
12 struts.contentType.exe=application/octet-stream
附:个人网站www.nxl123.cn(后台采用Python Flask框架搭建,2019年1月1日将升级完成并正式启用。哎,本人是学生狗呢!网站做的不好希望大家多多提意见或建议吧!?别骂我就好!……以后SEO什么的还得多向大家学习……)