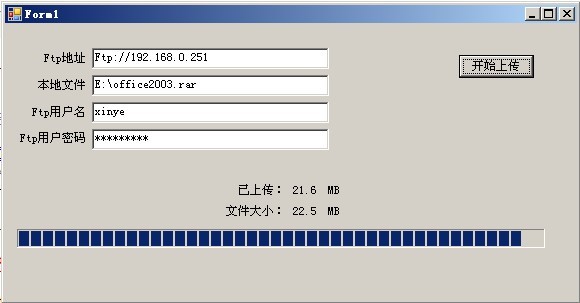
以下是Ftpupload类的代码 [代码写的简易,不包含异常处理,测试时请填写真实地址和和可用的FTP信息]
如果有朋友看了还不明白;请留个言;我会把连测试程序代码打包发给你

1 using System;
2 using System.Collections.Generic;
3 using System.Text;
4 using System.Net;
5 using System.IO;
6
7
8 /*
9 *[使用异步操作将文件上载到 FTP 服务器]
10 *
11 *BeginGetRequestStream和EndGetRequestStream
12 *
13 * *********************************************************************
14 * 参数:一个FTP服务器地址 string类型
15 *
16 * 一个本地文件的位置[需要上传的文件]; string类型
17 *
18 * 属性:设置要上传到FTP文件名为随机还是手动, bool类型
19 *
20 * FTP服务器用户名和密码;
21 *
22 * 使用次线程调用,控制进度条:
23 *
24 * Ftpupload a = new Ftpupload(path,finame,true);//true为自动更换随机文件名
25 * a.User = FTP用户名;
26 * a.Password = FTP密码;
27 * a.fileinfo = finfo;
28 * a.updateProgress+=new Ftpupload.ProgressHandler(a_updateProgress);
29 * a.UploadFtpFile();
30 *
31 * 异步线程操作需要判断控件InvokeRequired属性,然后可以调用委托更新进度
32 *
33 *
34 * *********************************************************************
35 *
36 */
37
38
39 namespace Jiangmingxiang.Program.Ftp_UpLoad
40 {
41 class Ftpupload
42 {
43 //委托更新进度-更新进度事件[updateProgress]
44 public delegate void ProgressHandler(long current, long total);
45 public event ProgressHandler updateProgress;
46 //成员声明
47 private FtpWebRequest ftpreq; //Ftp请求对象
48 private Uri uri;
49 private const int bufferLength = 2048; //设置缓冲区大小;
50 private string ftpuri;
51 private string fileuri;
52 private bool namerandom;
53 private string strUser,strPassword;
54 public FileInfo _fileinfo;
55
56
57 //属性声明
58 public string User
59 {
60 get { return strUser; }
61 set { strUser = value; }
62 }
63
64 public string Password
65 {
66 get { return strPassword; }
67 set { strPassword = value; }
68 }
69
70 public FileInfo fileinfo
71 {
72 get { return _fileinfo; }
73 set { _fileinfo= value; }
74 }
75
76 //构造函数
77 public Ftpupload(string _ftpuri, string _fileuri)
78 {
79 namerandom = false;
80 ftpuri = _ftpuri;//[得到FTP地址]
81 fileuri = _fileuri;//[得到本地文件地址]
82 // fileinfo = new FileInfo(fileuri);
83 }
84
85 public Ftpupload(string _ftpuri,string _fileuri,bool _namerandom)
86 {
87 namerandom = _namerandom;
88 ftpuri = _ftpuri;//[得到FTP地址]
89 fileuri = _fileuri;//[得到本地文件地址]
90 // fileinfo = new FileInfo(fileuri);
91 //UploadFtpFile();
92 }
93
94 //函数声明
95 public void UploadFtpFile()
96 {
97 if (namerandom)
98 {
99 DateTime tempdate = new DateTime();
100 tempdate = DateTime.Now;
101 uri = new Uri(ftpuri + "/" + tempdate.ToString() + fileinfo.Extension);
102 }
103 else
104 {
105 uri = new Uri(ftpuri+"/"+fileinfo.Name);
106 }
107 ftpreq = (FtpWebRequest)WebRequest.Create(uri);
108 ftpreq.Method = WebRequestMethods.Ftp.UploadFile;
109 NetworkCredential UserAndPassword = new NetworkCredential();
110
111 UserAndPassword.UserName = strUser;
112 UserAndPassword.Password = strPassword;
113 ftpreq.Credentials = UserAndPassword;
114 //开始以异步方式打开请求的内容流以便写入
115 ftpreq.BeginGetRequestStream(new AsyncCallback(EndGetStreamCallback),ftpreq);
116 }
117
118 private void EndGetStreamCallback(IAsyncResult ar)
119 {
120 Stream requestStream = null;
121 requestStream = ftpreq.EndGetRequestStream(ar);
122 byte[] buffer = new byte[bufferLength];
123 int count = 0; //记录已读文件流大小
124 int readBytes = 0; //记录是否读到文件流末尾
125 long filesize=fileinfo.Length;
126 FileStream stream = File.OpenRead(fileuri);//请求以流的方式写入到FTP上的本地文件
127 do
128 {
129 readBytes = stream.Read(buffer, 0, bufferLength);
130 requestStream.Write(buffer, 0, readBytes);
131 count += readBytes;
132 if (updateProgress != null)
133 {
134 updateProgress(count, filesize);
135 }
136 }
137 while (readBytes != 0);
138 requestStream.Close();
139 ftpreq.BeginGetResponse(new AsyncCallback(EndGetResponseCallback),ftpreq);
140 }
141
142 private void EndGetResponseCallback(IAsyncResult ar)
143 {
144 FtpWebResponse response = null;
145 response = (FtpWebResponse)ftpreq.EndGetResponse(ar);
146 response.Close();
147 }
148 }
149 }