帮助类
#region 生成验证码图片
public ActionResult SecurityCode()
{
string code = CreateRandomCode(5); //生成验证字符串
TempData["SecurityCode"] = code; //保存验证字符串
return File(CreateValidateGraphic(code), "image/Jpeg");
}
/// <summary>
/// 生成随机的字符串
/// </summary>
[NonAction]
private string CreateRandomCode(int codeCount)
{
string allChar = "0,1,2,3,4,5,6,7,8,9,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,W,X,Y,Z";
string[] allCharArray = allChar.Split(',');
string randomCode = "";
int temp = -1;
Random rand = new Random();
for (int i = 0; i < codeCount; i++)
{
if (temp != -1)
{
rand = new Random(i * temp * ((int)DateTime.Now.Ticks));
}
int t = rand.Next(35);
if (temp == t)
{
return CreateRandomCode(codeCount);
}
temp = t;
randomCode += allCharArray[t];
}
return randomCode;
}
/// <summary>
/// 创建验证码的图片
/// </summary>
[NonAction]
public byte[] CreateValidateGraphic(string validateCode)
{
Bitmap image = new Bitmap((int)Math.Ceiling(validateCode.Length * 16.0), 27);
Graphics g = Graphics.FromImage(image);
try
{
//生成随机生成器
Random random = new Random();
//清空图片背景色
g.Clear(Color.White);
//画图片的干扰线
for (int i = 0; i < 25; i++)
{
int x1 = random.Next(image.Width);
int x2 = random.Next(image.Width);
int y1 = random.Next(image.Height);
int y2 = random.Next(image.Height);
g.DrawLine(new Pen(Color.Silver), x1, y1, x2, y2);
}
Font font = new Font("Arial", 13, (FontStyle.Bold | FontStyle.Italic));
LinearGradientBrush brush = new LinearGradientBrush(new Rectangle(0, 0, image.Width, image.Height),
Color.Blue, Color.DarkRed, 1.2f, true);
g.DrawString(validateCode, font, brush, 3, 2);
//画图片的前景干扰点
for (int i = 0; i < 100; i++)
{
int x = random.Next(image.Width);
int y = random.Next(image.Height);
image.SetPixel(x, y, Color.FromArgb(random.Next()));
}
//画图片的边框线
g.DrawRectangle(new Pen(Color.Silver), 0, 0, image.Width - 1, image.Height - 1);
//保存图片数据
MemoryStream stream = new MemoryStream();
image.Save(stream, ImageFormat.Jpeg);
//输出图片流
return stream.ToArray();
}
finally
{
g.Dispose();
image.Dispose();
}
}
#endregion
前台调用
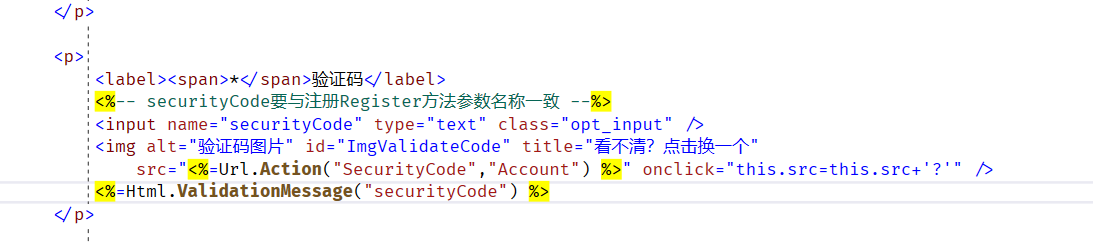
<% using (Html.BeginForm("Register", "Account", FormMethod.Post, new { id = "Form1", @class = "member_form" }))
{ %>
<p>
<label><span>*</span>用户名</label>
<%=Html.TextBox("LoginId", null, new { @class="opt_input" })%>
<%=Html.ValidationMessage("LoginId")%>
</p>
<p>
<label><span>*</span>真实姓名</label>
<%=Html.TextBox("Name", null, new {@class="opt_input" })%>
</p>
<p>
<label><span>*</span>密码</label>
<%=Html.Password("LoginPwd",null, new { @class="opt_input"})%>
<%=Html.ValidationMessage("LoginPwd")%>
</p>
<p>
<label><span>*</span>确认密码</label><input class="opt_input" name="PasswordConfirm" type="password" value="<%=Model != null ? Model.PasswordConfirm : ""%>" />
<%=Html.ValidationMessage("PasswordConfirm")%>
</p>
<p>
<label><span>*</span>电子邮件</label><input class="opt_input" name="Mail" type="text" value="<%= Model != null ? Model.Mail : ""%>" />
<%=Html.ValidationMessage("Mail")%>
</p>
<p>
<label><span>*</span>地址</label><input class="opt_input" name="Address" type="text" />
</p>
<p>
<label><span>*</span>手机</label><input class="opt_input" name="Phone" type="text" />
</p>
<p>
<label><span>*</span>验证码</label>
<%-- securityCode要与注册Register方法参数名称一致 --%>
<input name="securityCode" type="text" class="opt_input" />
<img alt="验证码图片" id="ImgValidateCode" title="看不清?点击换一个"
src="<%=Url.Action("SecurityCode","Account") %>" onclick="this.src=this.src+'?'" />
<%=Html.ValidationMessage("securityCode") %>
</p>
<p class="form_sub">
<input type="submit" value="确定了,马上提交" class="opt_sub" />
<%=Html.Submit("register","确定了,马上提交") %>
</p>
<p class="form_sub">加<span>*</span>的为必填项目</p>
<p class="form_sub">
><a href="login.html">已经有账号,马上登录</a><br />
>已有账号 <%= Html.ActionLink("点这里", "LogIn", "Account")%>登录
</p>
<%} %>