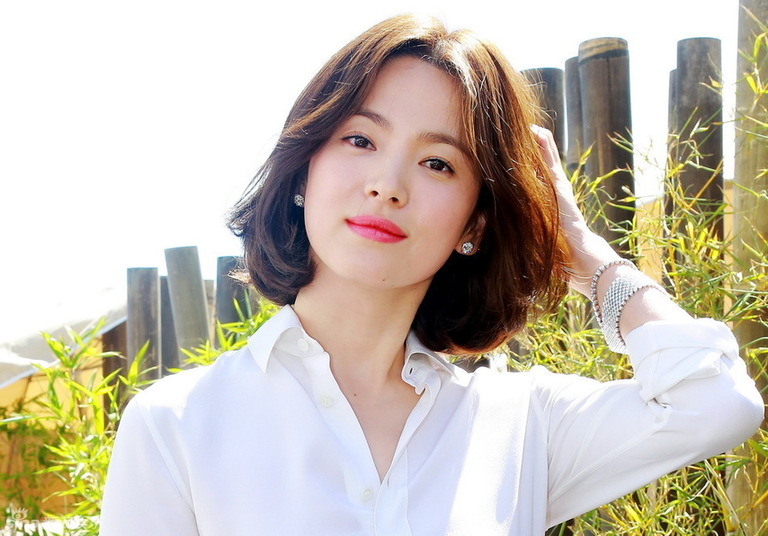
(1)需要导入的包
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
<version>1.5.0.RELEASE</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>2.8.0</version>
</dependency>
(2)redis配置文件
如果是springboot项目,可自己根据实际情况修改成类配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<bean id="jedisPoolConfig" class="redis.clients.jedis.JedisPoolConfig">
<property name="maxTotal" value="150"></property>
<property name="maxIdle" value="30"></property>
<property name="minIdle" value="10"></property>
<property name="maxWaitMillis" value="15000"></property>
<property name="minEvictableIdleTimeMillis" value="300000"></property>
<property name="numTestsPerEvictionRun" value="3"></property>
<property name="timeBetweenEvictionRunsMillis" value="60000"></property>
<property name="testOnBorrow" value="true"></property>
<property name="testOnReturn" value="true"></property>
<property name="testWhileIdle" value="true"></property>
</bean>
<bean id="jedisConnectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory" destroy-method="destroy">
<property name="hostName" value="127.0.0.1" />
<property name="port" value="6379" />
<property name="timeout" value="15000" />
<property name="database" value="0" />
<property name="password" value="" />
<property name="usePool" value="true" />
<property name="poolConfig" ref="jedisPoolConfig" />
</bean>
<!-- redis template definition p表示对该bean里面的属性进行注入,格式为p:属性名=注入的对象 效果与在bean里面使用<property>标签一样 -->
<bean id="redisTemplate" class="org.springframework.data.redis.core.RedisTemplate"
p:connection-factory-ref="jedisConnectionFactory">
<!-- 序列化方式 建议key/hashKey采用StringRedisSerializer。 -->
<property name="keySerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<property name="hashKeySerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<property name="valueSerializer">
<bean class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" />
</property>
<property name="hashValueSerializer">
<bean class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" />
</property>
</bean>
</beans>
(3)RedisUtil类
import java.io.Serializable;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.dao.DataAccessException;
import org.springframework.data.redis.connection.RedisConnection;
import org.springframework.data.redis.core.BoundSetOperations;
import org.springframework.data.redis.core.HashOperations;
import org.springframework.data.redis.core.ListOperations;
import org.springframework.data.redis.core.RedisCallback;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.data.redis.serializer.RedisSerializer;
import org.springframework.stereotype.Service;
@Service
public class RedisUtil
{
@Autowired
@Qualifier("redisTemplate")
public RedisTemplate<String, Object> redisTemplate;
@Autowired
@Qualifier("redisTemplate")
protected RedisTemplate<Serializable, Serializable> redisTemplateSerializable;
/**
* 缓存基本的对象,Integer、String、实体类等
* @param key 缓存的键值
* @param value 缓存的值
* @return 缓存的对象
*/
public void setCacheObject(String key, Object value)
{
redisTemplate.opsForValue().set(key,value);
}
public void removeCacheObject(String key){
redisTemplate.delete(key);
}
/**
* 获得缓存的基本对象。
* @param key 缓存键值
* @return 缓存键值对应的数据
*/
public Object getCacheObject(String key/*,ValueOperations<String,T> operation*/)
{
return redisTemplate.opsForValue().get(key);
}
/**
* 缓存List数据
* @param key 缓存的键值
* @param dataList 待缓存的List数据
* @return 缓存的对象
*/
public Object setCacheList(String key, List<Object> dataList)
{
ListOperations<String, Object> listOperation = redisTemplate.opsForList();
if(null != dataList)
{
int size = dataList.size();
for(int i = 0; i < size ; i ++)
{
listOperation.rightPush(key,dataList.get(i));
}
}
// redisTemplate.delete(key);
// listOperation.rightPushAll(key, dataList);
return listOperation;
}
/**
* 获得缓存的list对象
* @param key 缓存的键值
* @return 缓存键值对应的数据
*/
public List<Object> getCacheList(String key) {// List<Object> dataList = new ArrayList<Object>();
ListOperations<String, Object> listOperation = redisTemplate.opsForList();
List<Object> dataList = listOperation.range(key, 0, -1);
/*Long size = listOperation.size(key);
for(int i = 0 ; i < size ; i ++) {
dataList.add(listOperation.leftPop(key));
}*/
return dataList;
}
/**
* 根据多个key获取多个值
* @param keySet
* @return
*/
public List<Object> getCacheValueByKeySet(Set<String> keySet) {
ValueOperations<String, Object> valueOperation = redisTemplate.opsForValue();
List<Object> dataObject = valueOperation.multiGet(keySet);
return dataObject;
}
public List<Object> pipelineGetCacheValueByKeySet(final Set<String> keySet){
//pipeline
RedisCallback<List<Object>> pipelineCallback = new RedisCallback<List<Object>>() {
@Override
public List<Object> doInRedis(RedisConnection connection) throws DataAccessException {
connection.openPipeline();
for (String key : keySet) {
connection.incr(key.getBytes());
}
return connection.closePipeline();
}
};
List<Object> results = (List<Object>)redisTemplate.execute(pipelineCallback);
return results;
}
/**
* 获得缓存的list对象
* @Title: range
* @Description: TODO(这里用一句话描述这个方法的作用)
* @param @param key
* @param @param start
* @param @param end
* @param @return
* @return List<T> 返回类型
* @throws
*/
public List<Object> range(String key, long start, long end)
{
ListOperations<String, Object> listOperation = redisTemplate.opsForList();
return listOperation.range(key, start, end);
}
/**
* list集合长度
* @param key
* @return
*/
public Long listSize(String key) {
return redisTemplate.opsForList().size(key);
}
/**
* 覆盖操作,将覆盖List中指定位置的值
* @param key
* @param index 位置
* @param obj 值
* @return 状态码
* */
public void listSet(String key, int index, Object obj) {
redisTemplate.opsForList().set(key, index, obj);
}
/**
* 向List尾部追加记录
*
* @param key
* @param obj
* @return 记录总数
* */
public long leftPush(String key, Object obj) {
return redisTemplate.opsForList().leftPush(key, obj);
}
/**
* 向List头部追加记录
*
* @param key
* @param obj
* @return 记录总数
* */
public long rightPush(String key, Object obj) {
return redisTemplate.opsForList().rightPush(key, obj);
}
/**
* 算是删除吧,只保留start与end之间的记录
*
* @param key
* @param start 记录的开始位置(0表示第一条记录)
* @param end 记录的结束位置(如果为-1则表示最后一个,-2,-3以此类推)
* @return 执行状态码
* */
public void trim(String key, int start, int end) {
redisTemplate.opsForList().trim(key, start, end);
}
/**
* 删除List中c条记录,被删除的记录值为value
*
* @param key
* @param i 要删除的数量,如果为负数则从List的尾部检查并删除符合的记录
* @param obj 要匹配的值
* @return 删除后的List中的记录数
* */
public long remove(String key, long i, Object obj) {
return redisTemplate.opsForList().remove(key, i, obj);
}
/**
* 缓存Set
* @param key 缓存键值
* @param dataSet 缓存的数据
* @return 缓存数据的对象
*/
public BoundSetOperations<String, Object> setCacheSet(String key,Set<Object> dataSet)
{
BoundSetOperations<String, Object> setOperation = redisTemplate.boundSetOps(key);
/*T[] t = (T[]) dataSet.toArray();
setOperation.add(t);*/
Iterator<Object> it = dataSet.iterator();
while(it.hasNext())
{
setOperation.add(it.next());
}
return setOperation;
}
/**
* 获得缓存的set
* @param key
* @return
*/
public Set<Object> getCacheSet(String key/*,BoundSetOperations<String,T> operation*/)
{
Set<Object> dataSet = new HashSet<Object>();
BoundSetOperations<String,Object> operation = redisTemplate.boundSetOps(key);
Long size = operation.size();
for(int i = 0 ; i < size ; i++)
{
dataSet.add(operation.pop());
}
return dataSet;
}
/**
* 缓存Map
* @param key
* @param dataMap
* @return
*/
public int setCacheMap(String key,Map<Object, Object> dataMap)
{
if(null != dataMap)
{
HashOperations<String, Object, Object> hashOperations = redisTemplate.opsForHash();
hashOperations.putAll(key,dataMap);
// for (Map.Entry<Object, Object> entry : dataMap.entrySet()) {
// /*System.out.println("Key = " + entry.getKey() + ", Value = " + entry.getValue()); */
// if(hashOperations != null){
// hashOperations.put(key,entry.getKey(),entry.getValue());
// } else{
// return 0;
// }
// }
} else{
return 0;
}
return dataMap.size();
}
/**
* 删除缓存Map
* @param key
* @param dataMap
* @return
*/
public void removeCacheMap(String key, Map<Object, Object> dataMap) {
if (null != dataMap) {
HashOperations<String, Object, Object> hashOperations = redisTemplate.opsForHash();
for (Map.Entry<Object, Object> entry : dataMap.entrySet()) {
hashOperations.delete(key, entry.getKey(), entry.getValue());
}
}
}
/**
* 获得缓存的Map
* @param key
* @return
*/
public Map<Object, Object> getCacheMap(String key/*,HashOperations<String,String,T> hashOperation*/)
{
Map<Object, Object> map = redisTemplate.opsForHash().entries(key);
/*Map<String, T> map = hashOperation.entries(key);*/
return map;
}
/**
* 缓存Map
* @param key
* @param dataMap
* @return
*/
public void setCacheIntegerMap(String key,Map<Integer, Object> dataMap)
{
HashOperations<String, Object, Object> hashOperations = redisTemplate.opsForHash();
if(null != dataMap)
{
for (Map.Entry<Integer, Object> entry : dataMap.entrySet()) {
/*System.out.println("Key = " + entry.getKey() + ", Value = " + entry.getValue()); */
hashOperations.put(key,entry.getKey(),entry.getValue());
}
}
}
/**
* 获得缓存的Map
* @param key
* @return
*/
public Map<Object, Object> getCacheIntegerMap(String key/*,HashOperations<String,String,T> hashOperation*/)
{
Map<Object, Object> map = redisTemplate.opsForHash().entries(key);
/*Map<String, T> map = hashOperation.entries(key);*/
return map;
}
/**
* 从hash中删除指定的存储
*
* @return 状态码,1成功,0失败
* */
public void deleteMap(String key) {
redisTemplate.setEnableTransactionSupport(true);
redisTemplate.opsForHash().delete(key);
}
/**
* 设置过期时间
* @param key
* @param time
* @param unit
* @return
*/
public boolean expire(String key, long time, TimeUnit unit) {
return redisTemplate.expire(key, time, unit);
}
/**
* 获取过期时间
* @param key
* @param unint
* @return
*/
public Long getExpire(String key,TimeUnit unint) {
return redisTemplate.getExpire(key, unint);
}
/**
* increment
* @param key
* @param step
* @return
*/
public long increment(String key, long step) {
return redisTemplate.opsForValue().increment(key, step);
}
/**
* 判断是否存在key
* @param key
* @return
*/
public boolean hasKey(String key){
return redisTemplate.hasKey(key);
}
/**
*
* @param map
*/
public void batchSetObject(final Map<String, Object> map){
redisTemplate.executePipelined(new RedisCallback() {
@Override
public Object doInRedis(RedisConnection connection)
throws DataAccessException {
for(String key : map.keySet()){
byte[] rawKey = serialize(key, redisTemplate.getKeySerializer());
byte[] rawValue = serialize(map.get(key), redisTemplate.getValueSerializer());
connection.set(rawKey, rawValue);
}
return null;
}
});
}
/**
* 删除redis的所有数据
*/
@SuppressWarnings({"unchecked", "rawtypes"})
public String flushDB() {
return redisTemplateSerializable.execute(new RedisCallback() {
public String doInRedis(RedisConnection connection) throws DataAccessException {
connection.flushDb();
return "ok";
}
});
}
/**
* 删除redis的一条数据
*/
public long del(final byte[] key) {
return (long) redisTemplateSerializable.execute(new RedisCallback<Object>() {
public Long doInRedis(RedisConnection connection) {
return connection.del(key);
}
});
}
@SuppressWarnings({"unchecked", "rawtypes"})
public byte[] get(final byte[] key) {
return (byte[]) redisTemplateSerializable.execute(new RedisCallback() {
public byte[] doInRedis(RedisConnection connection) throws DataAccessException {
return connection.get(key);
}
});
}
/**
* @param key
* @param value
* @param liveTime
*/
public void set(final byte[] key, final byte[] value, final long liveTime) {
redisTemplateSerializable.execute(new RedisCallback<Object>() {
public Long doInRedis(RedisConnection connection) throws DataAccessException {
connection.set(key, value);
if (liveTime > 0) {
connection.expire(key, liveTime);
}
return 1L;
}
});
}
/**
* 发布信息
* @param channel
* @param value
*/
public void convertAndSend(String channel, String value){
redisTemplate.convertAndSend(channel,value);
}
@SuppressWarnings("unchecked")
private byte[] serialize(Object value, RedisSerializer serializer) {
if (serializer == null && value instanceof byte[]) {
return (byte[]) value;
}
return serializer.serialize(value);
}
}