转自:http://4137613.blog.51cto.com/4127613/754729
第一部分:基本图形绘制
cocos2dx封装了大量opengl函数,用于快速绘制基本图形,这些代码的例子在,testsDrawPrimitivesTest目录下
注意,该方法是重载node的draw方法实现的,在智能机上,并不推荐直接绘制几何图形,因为大量的坐标编码会极大降低工作效率,应尽量使用Image。而且cocos2dx的渲染机制会造成前后遮挡问题,尤其是几何图形与图片等其他node混合绘制时。
void DrawPrimitivesTest::draw() { CCLayer::draw(); CCSize s = CCDirector::sharedDirector()->getWinSize(); // draw a simple line // The default state is: // Line Width: 1 // color: 255,255,255,255 (white, non-transparent) // Anti-Aliased glEnable(GL_LINE_SMOOTH); ccDrawLine( CCPointMake(0, 0), CCPointMake(s.width, s.height) ); // line: color, width, aliased // glLineWidth > 1 and GL_LINE_SMOOTH are not compatible //注意:线宽>1 则不支持GL_LINE_SMOOTH // GL_SMOOTH_LINE_WIDTH_RANGE = (1,1) on iPhone glDisable(GL_LINE_SMOOTH); glLineWidth( 5.0f ); /*glColor4ub(255,0,0,255);*/ glColor4f(1.0, 0.0, 0.0, 1.0); ccDrawLine( CCPointMake(0, s.height), CCPointMake(s.width, 0) ); // TIP: // If you are going to use always the same color or width, you don't // need to call it before every draw // // Remember: OpenGL is a state-machine. // draw big point in the center // 注意:cocos2dx绘制的是方块点 glPointSize(64); /*glColor4ub(0,0,255,128);*/ glColor4f(0.0, 0.0, 1.0, 0.5); ccDrawPoint( CCPointMake(s.width / 2, s.height / 2) ); // draw 4 small points // 注意:cocos2dx绘制的是方块点 CCPoint points[] = { CCPointMake(60,60), CCPointMake(70,70), CCPointMake(60,70), CCPointMake(70,60) }; glPointSize(4); /*glColor4ub(0,255,255,255);*/ glColor4f(0.0, 1.0, 1.0, 1.0); ccDrawPoints( points, 4); // draw a green circle with 10 segments glLineWidth(16); /*glColor4ub(0, 255, 0, 255);*/ glColor4f(0.0, 1.0, 0.0, 1.0); //参数依次是:中心点,半径,角度,分段数,是否连接中心点 ccDrawCircle( CCPointMake(s.width/2, s.height/2), 100, 0, 10, false); // draw a green circle with 50 segments with line to center glLineWidth(2); /*glColor4ub(0, 255, 255, 255);*/ glColor4f(0.0, 1.0, 1.0, 1.0); ccDrawCircle( CCPointMake(s.width/2, s.height/2), 50, CC_DEGREES_TO_RADIANS(90), 50, true); // open yellow poly /*glColor4ub(255, 255, 0, 255);*/ glColor4f(1.0, 1.0, 0.0, 1.0); glLineWidth(10); CCPoint vertices[] = { CCPointMake(0,0), CCPointMake(50,50), CCPointMake(100,50), CCPointMake(100,100), CCPointMake(50,100) }; //参数依次是:点数组,点数量,是否封闭 ccDrawPoly( vertices, 5, false); // closed purple poly /*glColor4ub(255, 0, 255, 255);*/ glColor4f(1.0, 0.0, 1.0, 1.0); glLineWidth(2); CCPoint vertices2[] = { CCPointMake(30,130), CCPointMake(30,230), CCPointMake(50,200) }; ccDrawPoly( vertices2, 3, true); // draw quad bezier path //绘制有一个控制点的贝塞尔曲线 ccDrawQuadBezier(CCPointMake(0,s.height), CCPointMake(s.width/2,s.height/2), CCPointMake(s.width,s.height), 50); // draw cubic bezier path //绘制有两个控制点的贝塞尔曲线 ccDrawCubicBezier(CCPointMake(s.width/2, s.height/2), CCPointMake(s.width/2+30,s.height/2+50), CCPointMake(s.width/2+60,s.height/2-50),CCPointMake(s.width, s.height/2),100); //恢复opengl的正常参数 // restore original values glLineWidth(1); /*glColor4ub(255,255,255,255);*/ glColor4f(1.0, 1.0, 1.0, 1.0); glPointSize(1); }
第二部分:字符串绘制
#1 cocos2dx的字符串绘制使用的是Label,cocos2dx并不直接支持在屏幕中绘制字符串(这是有道理的,因为我们不能直接把一个string做成一个节点,那样很难理解),如果要直接绘制的话,可以自己封装opengl函数(网上有很多例子,一般是用texture做)。
其实最简单的绘制例子就是最开始的那个Helloworld。核心代码如下:
// Create a label and initialize with string "Hello World". CCLabelTTF* pLabel = CCLabelTTF::labelWithString("Hello World", "Thonburi", 64); CC_BREAK_IF(! pLabel); // Get window size and place the label upper. CCSize size = CCDirector::sharedDirector()->getWinSize(); pLabel->setPosition(ccp(size.width / 2, size.height - 20)); // Add the label to HelloWorld layer as a child layer. this->addChild(pLabel, 1);
建立一个CCLabelTTF并添加到子节点即可。
#2 绘制中文
但,如果绘制中文呢?
CCLabelTTF* pLabel = CCLabelTTF::labelWithString("你好,世界", "Thonburi", 64);
注意:需要使用VS的另存为功能
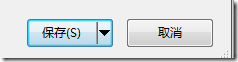
将含有中文字符串的源代码,保存为UTF-8格式

否则显示为乱码。
手动保存比较麻烦,可以使用批量转换工具,如:boomworks的“文件编码转换工具”
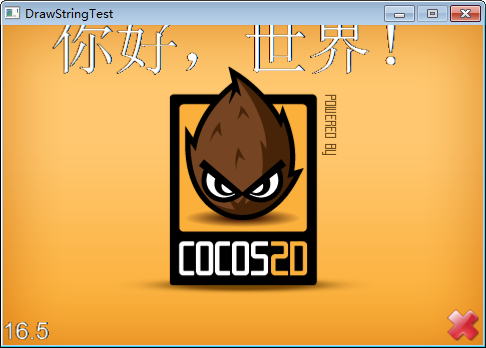
#3 文字锚点对齐与坐标计算
为了便于字体对齐,我们在很多游戏引擎中,都使用对齐锚点的功能,如j2me的anchor参数接口。
我们添加一个CCLayer,并重载他的draw函数,然后在draw中绘制十字线。
void HelloWorldLayer::draw() { CCLayer::draw(); CCSize s = CCDirector::sharedDirector()->getWinSize(); glEnable(GL_LINE_SMOOTH); ccDrawLine( CCPointMake(0, s.height/2), CCPointMake(s.width, s.height/2) ); ccDrawLine( CCPointMake(s.width/2, 0), CCPointMake(s.width/2, s.height) ); }
然后,我们重写绘制字体函数,将坐标修改为屏幕正中
pLabel->setPosition(ccp(size.width / 2, size.height/2));
可以看到,cocos2d默认锚点是node的中心。
.png)

如果要采用其他方式对齐,如左上角,可以使用getContentSize()获取CCSize。然后调整位置。
注意:中文字符获取宽高似乎有bug,在win32上面获得不了准确的数值。
注意:由于手机不同平台的适配方案不同,我们在写坐标时,不要使用绝对坐标值的加减,而应使用比例,乘除等方法。否则,开启适配函数后,坐标值会被映射成多个像素点,造成渲染错位。
第三部分:绘制图片
cocos2dx中并没有直接绘制图片的概念,我们一般是使用CCSprite。核心代码如下:
// 3. Add add a splash screen, show the cocos2d splash image. CCSprite* pSprite = CCSprite::spriteWithFile("HelloWorld.png"); CC_BREAK_IF(! pSprite); // Place the sprite on the center of the screen pSprite->setFlipX(true); //可以手动设置图形旋转和镜像,而不是使用Action,因为有许多Action是个过程,而不是直接显示结果 pSprite->setRotation(90); pSprite->setPosition(ccp(size.width/2, size.height/2)); // Add the sprite to HelloWorld layer as a child layer. this->addChild(pSprite, 0);
绘制后的效果如下: