1. MVC && MVVM 定义及区别
MVC:Model - View - Controller
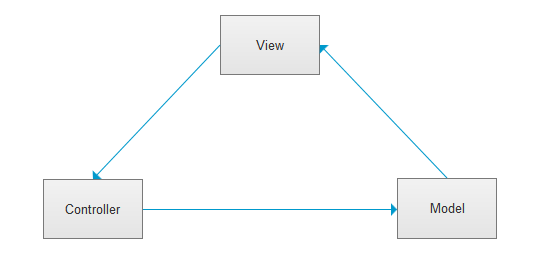
MVC 所有的通信都是单向的
MVVM: Model - View - ViewModel
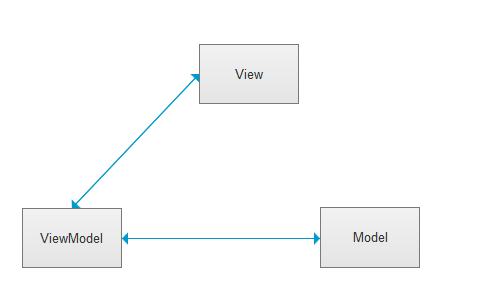
MVVM模式采用双向绑定,View的变动,自动反映在ViewModel中;反之 ViewModel的变动也会反映在View中。
2. JS原型
js中,所有类型的数据都拥有__proto__ 属性(隐式原型)
所有函数都拥有prototype属性(显式原型)
原型对象:拥有prototype属性的对象,在定义函数时就被自动创建
js面向对象编程:JavaScript的所有数据都可以看成对象,但它没有其他语言如Java、C#的类和实例的概念,JavaScript不区分类和实例,而是通过原型(prototype)来实现面向对象编程。
js面向对象编程主要体现在继承上,如:
/** 1.构造函数实现继承,缺点是只实现了部分继承,无法继承父类原型(prototype)对象的方法 */ function Parent1() { this.age = "parent1"; this.arr = [1,2,3]; } function Child1() { Parent1.call(this); //使用call继承 this.type = "child1" } Parent1.prototype.age = function () {} var a = new Child1(); console.log(a) /** 2.原型链继承,缺点是改变第一个对象的属性,第二个对象的属性也跟着改变了 */ function Parent2() { this.name = "parent2"; this.arr = [1,2,3]; } function Child2() { this.type = "child2" } Child2.prototype = new Parent2(); //原型链继承 // 继承的属性和方法挂载原型对象(__proto__)上,如果修改原型对象上的属性和方法,则其他实例中的属性和方法也跟着改变 var a = new Child2(); var b = new Child2(); a.arr.push(4) console.log(a.arr, b.arr) /** 3.组合继承 结合了构造函数方式和原型链继承方式的优点,避免了缺点 */ function Parent3(){ this.name ="parent3"; this.arr = [1,2,3]; } function Child3(){ Parent3.call(this) this.type = "child3" } Parent3.prototype.age = function(){} Child3.prototype = new Parent3() var a = new Child3(); var b = new Child3(); a.arr.push(4) console.log(a,b) /** 4.组合继承优化1 不用两次调用new Parent4(),缺点是构造函数指向的是一个(Parent4),无法区分实例是由父类创建还是由子类创建的 */ function Parent4(){ this.name = "parent4"; this.arr = [1,2,3]; } function Child4(){ Parent4.call(this); this.type = "child4" } Child4.prototype = Parent4.prototype; var a = new Child4(); var b = new Child4(); console.log(a instanceof Child4 , a instanceof Parent4) //true true console.log(a.constructor,b.constructor) //Parent4 /** 5.组合继承优化2 */ function Parent5(){ this.name = "parent5"; this.arr = [1,2,3]; } function Child5(){ Parent5.call(this); this.type = "child5"; } Child5.prototype = Object.create(Parent5.prototype); Child5.prototype.constructor = Child5; var a=new Child5(); console.log(a instanceof Parent5,a instanceof Child5); //true true console.log(a.constructor); //Child5
3. Angular、Angularjs
Angular:https://zhuanlan.zhihu.com/p/80791364
https://blog.csdn.net/powertoolsteam/article/details/65442639
Angularjs: https://blog.csdn.net/qq_34543438/article/details/72793622
4. ES6、TypeScript
TypeScript:https://www.tslang.cn/docs/home.html
5. Webpack、Gulp
webpack常见配置如下:
const HtmlWebpackPlugin = require('html-webpack-plugin'); //通过 npm 安装 const webpack = require('webpack'); //访问内置的插件 const path = require('path'); const config = { entry: './path/to/my/entry/file.js', output: { filename: 'my-first-webpack.bundle.js', path: path.resolve(__dirname, 'dist') }, module: { rules: [ { test: /.(js|jsx)$/, use: 'babel-loader' } ] }, plugins: [ new webpack.optimize.UglifyJsPlugin(), new HtmlWebpackPlugin({template: './src/index.html'}) ] }; module.exports = config;
配置主要包括 entry(入口),output(出口),module(配置规则等),plugins(插件配置)
gulp常见配置如下:
var gulp = require('gulp') gulp.task('static', function () { gulp.src('./src/font/**/*') .pipe(gulp.dest('./dist/font')); gulp.src('./src/images/**/*') .pipe(gulp.dest('./dist/images')); gulp.src('./src/html/*.html') .pipe(gulp.dest('./dist/html')); gulp.src('./src/css/**/*') .pipe(gulp.dest('dist/css')); console.log('css is done!'); }); gulp.task("default",['static'], function () { });
主要是把资源文件通过src引入,然后再pipe到其它处理逻辑。