1. Description: 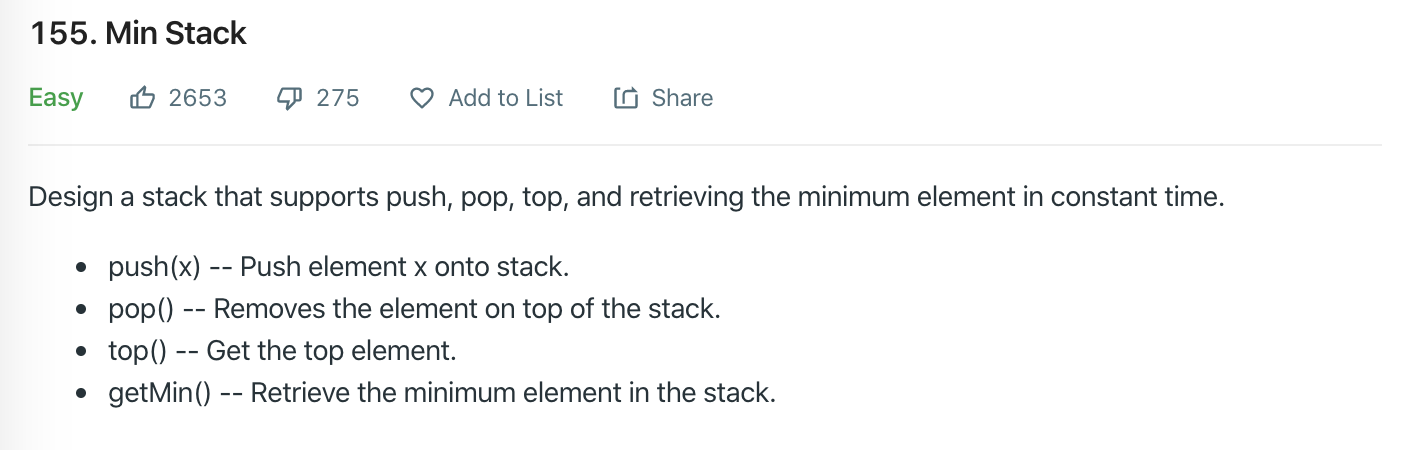
2. Examples:

3.Solutions:
1 /**
2 * Created by sheepcore on 2019-05-07
3 * Your MinStack object will be instantiated and called as such:
4 * MinStack obj = new MinStack();
5 * obj.push(x);
6 * obj.pop();
7 * int param_3 = obj.top();
8 * int param_4 = obj.getMin();
9 */
10 class MinStack {
11 /** initialize your data structure here. */
12 private Stack<Integer> stack;
13
14 public MinStack(){
15 stack = new Stack<Integer>();
16 }
17 public int getMin(){
18 int min = Integer.MAX_VALUE;
19 for(Integer integer : stack){
20 if(integer < min)
21 min = integer;
22 }
23 return min;
24 }
25 public void push(int x){
26 stack.push(x);
27 }
28
29 public int top(){
30 return stack.peek();
31 }
32
33 public int pop(){
34 return stack.pop();
35 }
36 }