1. Description:
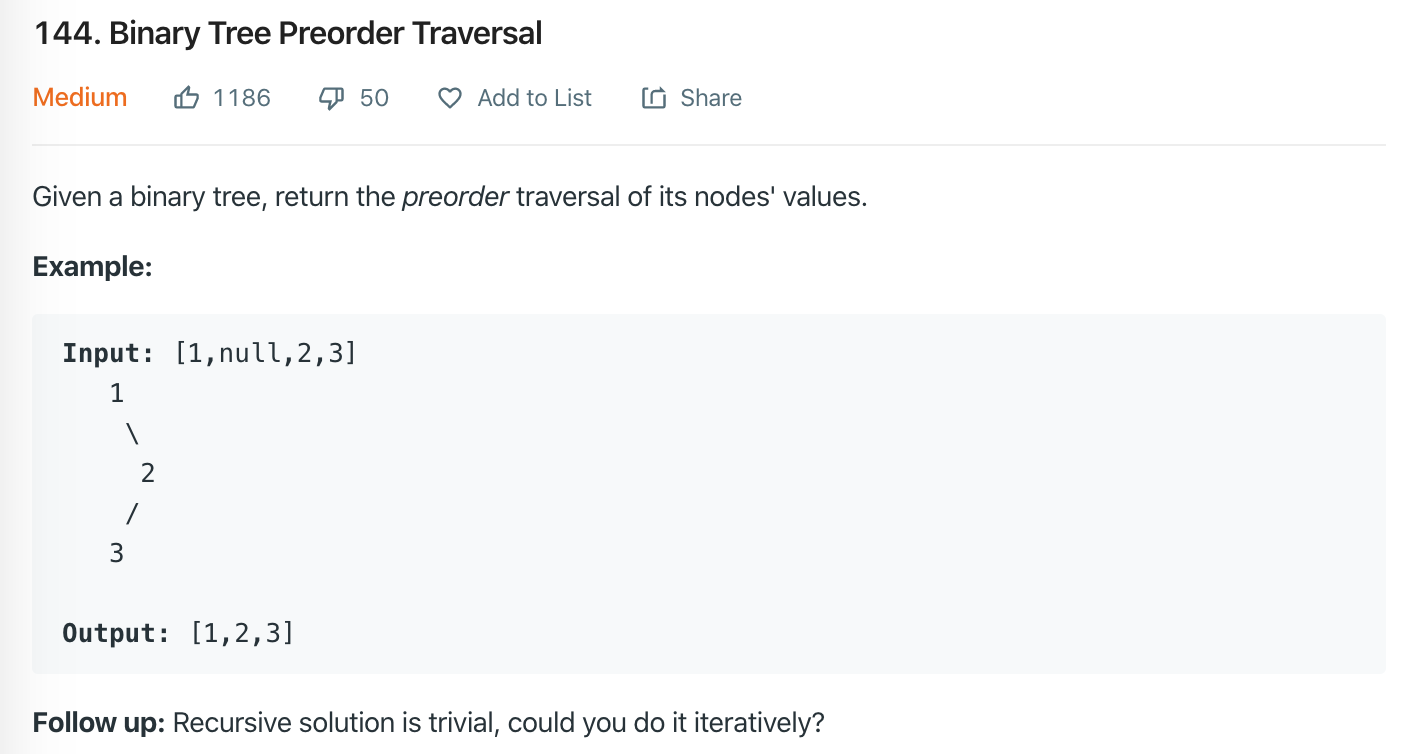
2.Solutions:
1 /**
2 * Created by sheepcore on 2019-05-09
3 * Definition for a binary tree node.
4 * public class TreeNode {
5 * int val;
6 * TreeNode left;
7 * TreeNode right;
8 * TreeNode(int x) { val = x; }
9 * }
10 */
11 class Solution {
12 public List<Integer> preorderTraversal(TreeNode root) {
13 List<Integer> list = new ArrayList<>();
14 Stack<TreeNode> stack = new Stack<>();
15 while(root != null || !stack.isEmpty()){
16 while(root != null){
17 list.add(root.val);
18 stack.push(root);
19 root = root.left;
20 }
21 if(!stack.isEmpty()) {
22 root = stack.pop();
23 root = root.right;
24 } else
25 return list;
26 }
27 return list;
28 }
29 }