代码:
#include <iostream>
#include <stack>
# include <string>
#include <stdexcept>
#include <stack>
# include <string>
#include <stdexcept>
using namespace std;
class My_Queue
{
public:
My_Queue(){};
~My_Queue(){};
int add(int var);
int poll();
int peek();
bool empty();
bool s_push_empty();
int stackPush_pop();
private:
stack<int> stackPush;
stack<int> stackPop;
};
{
public:
My_Queue(){};
~My_Queue(){};
int add(int var);
int poll();
int peek();
bool empty();
bool s_push_empty();
int stackPush_pop();
private:
stack<int> stackPush;
stack<int> stackPop;
};
//实现代码
int My_Queue::add(int var)
{
stackPush.push(var);
return 0;
}
int My_Queue::add(int var)
{
stackPush.push(var);
return 0;
}
int My_Queue::poll()
{
{
if(stackPush.empty()&&stackPop.empty()){
throw runtime_error("Empty Queue !");
}
else{
while(!stackPush.empty()){
int top_val = stackPush.top();
stackPush.pop();
stackPop.push(top_val);
}
}
int top_value = stackPop.top();//返回stackPop栈顶元素的引用
stackPop.pop();//弹出stackPop栈顶元素
return top_value;
}
throw runtime_error("Empty Queue !");
}
else{
while(!stackPush.empty()){
int top_val = stackPush.top();
stackPush.pop();
stackPop.push(top_val);
}
}
int top_value = stackPop.top();//返回stackPop栈顶元素的引用
stackPop.pop();//弹出stackPop栈顶元素
return top_value;
}
int My_Queue::peek()
{
if(stackPush.empty()&&stackPop.empty()){
throw runtime_error("Empty Queue !");
}
else{
while(!stackPush.empty()){
int top_val = stackPush.top();
stackPush.pop();
stackPop.push(top_val);
}
}
int top_value = stackPop.top();
return top_value;
}
bool My_Queue::empty()
{
if(stackPush.empty()&&stackPop.empty())
return true;
else
return false;
}
int My_Queue::stackPush_pop()//stackPush弹出
{
{
if(stackPush.empty()&&stackPop.empty()){
throw runtime_error("Empty Queue !");
}
else{
while(!stackPush.empty()){
int top_val = stackPush.top();
stackPush.pop();
stackPop.push(top_val);
}
}
int top_value = stackPop.top();
return top_value;
}
bool My_Queue::empty()
{
if(stackPush.empty()&&stackPop.empty())
return true;
else
return false;
}
int My_Queue::stackPush_pop()//stackPush弹出
{
if(!stackPush.empty()){
int _top_var = stackPush.top();
int _top_var = stackPush.top();
std::cout << _top_var << '
';
stackPush.pop();
}
}
bool My_Queue::s_push_empty()//判断stackPush为空?
{
if(stackPush.empty())
return true;
else
return false;
}
stackPush.pop();
}
}
bool My_Queue::s_push_empty()//判断stackPush为空?
{
if(stackPush.empty())
return true;
else
return false;
}
//测试代码
int main()
{
My_Queue myqueue;
int a[] = {1,3,2,2,1,5};
for(int i=0;i<6;i++)
{
myqueue.add(a[i]);
}
std::cout << "this is stackpush_data:" << ' ';
while (!myqueue.s_push_empty()) {
int main()
{
My_Queue myqueue;
int a[] = {1,3,2,2,1,5};
for(int i=0;i<6;i++)
{
myqueue.add(a[i]);
}
std::cout << "this is stackpush_data:" << ' ';
while (!myqueue.s_push_empty()) {
myqueue.stackPush_pop();//弹出stackPush中所有元素
}
}
for(int i=0;i<6;i++)
{
myqueue.add(a[i]);
}
std::cout << "this is queue stackPop_data(from_peek_function):" << myqueue.peek() << ' ';//弹出stackPop栈顶元素
std::cout << ' ';
std::cout << "this is queue stackPop_data(from_poll_function):" << ' ';
while(!myqueue.empty())
{
{
myqueue.add(a[i]);
}
std::cout << "this is queue stackPop_data(from_peek_function):" << myqueue.peek() << ' ';//弹出stackPop栈顶元素
std::cout << ' ';
std::cout << "this is queue stackPop_data(from_poll_function):" << ' ';
while(!myqueue.empty())
{
std::cout << myqueue.poll() << '
';//弹出stackPop所有元素
}
return 0;
}
return 0;
}
测试结果:
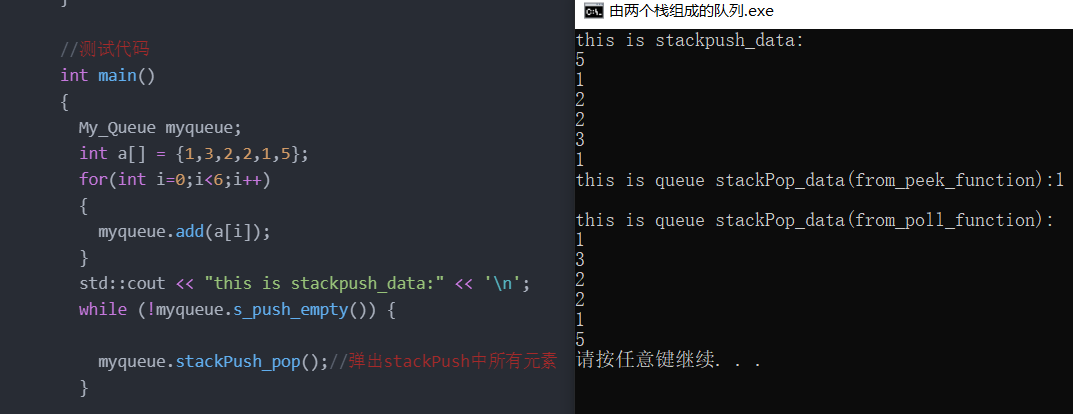