1.使用useHistory做页面跳转导航
1导入
import { useHistory } from "react-router-dom";
2.使用跳转页面
function Home() { const history = useHistory(); function handleClick() { history.push("/home"); } return ( <button type="button" onClick={handleClick}>Go home </button> ); }
3.使用跳转页面携带参数
import { useHistory } from "react-router-dom"; function HomeButton() { const history = useHistory(); let id = 1; function handleClick() { history.push({pathname: '/home', state: {id: id}}) } return ( <button type="button" onClick={handleClick}> Go home </button> ); }
2.使用useLocation 获取跳转携带的值
4.在home页面中获取id值
导入
import { useLocation } from 'react-router-dom';
const location = useLocation(); //获取跳转页面携带的值
console.log(location.state.id);
3.React useHistory 更新为useNavigate如何传值
1.组件跳转并传值
(1)导入
import { useNavigate } from ‘react-router-dom’;
(2)使用
const navigate = useNavigate();
点击事件中使用
(1)导入
import { useNavigate } from ‘react-router-dom’;
(2)使用
const navigate = useNavigate();
点击事件中使用

组件“/machine”为已经定义好的路由,state负责传值state:{参数:值}
(3)获取值
导入import { useLocation } from ‘react-router-dom’;
使用
let location = useLocation();
let server_id = location.state;
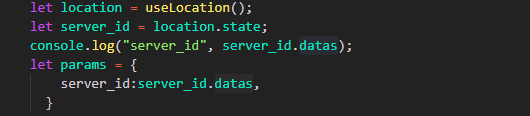
如果是React Router v4,可以使用以下方法:
1、使用withRouter组件
import { withRouter } from 'react-router-dom'; const Button = withRouter(({ history }) => ( <button type="button" onClick={() => { history.push('/new-location'); }} > {' '} Click Me!{' '} </button> ));
2、使用<Route>标签
import { Route } from 'react-router-dom'; const Button = () => ( <Route render={({ history }) => ( <button type="button" onClick={() => { history.push('/new-location'); }} > {' '} Click Me!{' '} </button> )} /> );
3、使用context(这个方法不推荐,context api不是很稳定。)
const Button = (props, context) => (
<button
type="button"
onClick={() => {
context.history.push('/new-location');
}}
>
Click Me!{' '}
</button>
);
Button.contextTypes = {
history: React.PropTypes.shape({
push: React.PropTypes.func.isRequired,
}),
};