一、引入maven依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
二、配置application.properties
# activemq
spring.activemq.broker-url=tcp://localhost:61616
spring.activemq.user=admin
spring.activemq.password=admin
#默认为true表示使用内存的activeMQ,不需要安装activeMQ server
spring.activemq.in-memory=false
#如果此处设置为true,需要加如下的依赖包 <groupId>org.apache.activemq</groupId>
# <artifactId>activemq-pool</artifactId> ,否则会自动配置失败,报JmsMessagingTemplate注入失败
spring.activemq.pool.enabled=false
- 注意value后面不能加空格,即spring.activemq.pool.enabled=false[空格]
三、在启动类中使用异步消息服务@EnableJms注解
@EnableJms
@SpringBootApplication
public class SpringbootActiveMqApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootActiveMqApplication.class, args);
}
}
四、编写消息生产者
package com.shyroke.jms;
import javax.jms.Destination;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jms.core.JmsMessagingTemplate;
import org.springframework.stereotype.Service;
@Service("producer")
public class Producer {
@Autowired // 也可以注入JmsTemplate,JmsMessagingTemplate对JmsTemplate进行了封装
private JmsMessagingTemplate jmsTemplate;
// 发送消息,destination是发送到的队列,message是待发送的消息
public void sendMessage(Destination destination, final String message) {
jmsTemplate.convertAndSend(destination, message);
}
}
五、编写两个消费者
package com.shyroke.jms;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class Consumer {
// 使用JmsListener配置消费者监听的队列,其中text是接收到的消息
@JmsListener(destination = "mytest.queue")
public void receiveQueue(String text) {
System.out.println("Consumer收到的报文为:"+text);
System.out.println("=================");
}
}
package com.shyroke.jms;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class Consumer2 {
// 使用JmsListener配置消费者监听的队列,其中text是接收到的消息
@JmsListener(destination = "mytest.queue")
public void receiveQueue(String text) {
System.out.println("Consumer2收到的报文为:"+text);
}
}
六、测试
package com.shyroke;
import javax.jms.Destination;
import org.apache.activemq.command.ActiveMQQueue;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import com.shyroke.jms.Producer;
@RunWith(SpringRunner.class)
@SpringBootTest
public class SpringbootActiveMqApplicationTests {
@Autowired
private Producer producer;
@Test
public void contextLoads() {
Destination destination=new ActiveMQQueue("mytest.queue");
for(int i=0; i<5; i++){
producer.sendMessage(destination, "生产者发送了消息"+i);
}
}
}
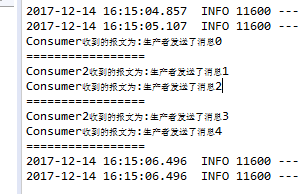