二、Connector/c++库的编译:
1、把MySql数据库安装完成后,把bin目录加入环境变量。
2、下载boost库,官网就有下载: http://www.boost.org/
3、安装cmake工具,加入环境变量。
命令行: cmake -G , 列出cmake支持生成工程文件的各个版本。
4、下载 Connector/c++ 源文件 :http://dev.mysql.com/downloads/connector/cpp/
Select Platform: 下拉选项选择 Source Code 。这里貌似要翻墙才行。
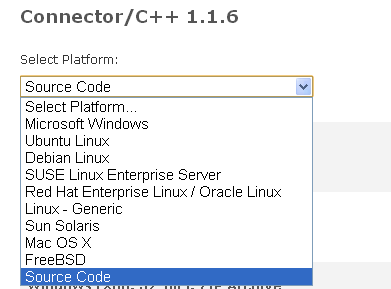
一、生成工程文件
解压Connector/c++ ,命令行 cd 到解压目录 。我编译的是2010版,命令如下:
cmake -G "Visual Studio 10 2010" -DBOOST_ROOT:STRING="E:GitHub"
-DBOOST_ROOT:STRING 指定 boost库所在目录
执行完后,就得到sln工程文件。我这里得到的是:MYSQLCPPCONN.sln
二、编译
打开工程文件,编译 MYSQLCPPCONN 方案。 在 mysql-connector-c++-1.1.6driverDebug 目录下就可以得到 dll 和 lib文件。
2.1、使用 mysqlcppconn.dll 你需要 libmysql.dll 库文件。
这个文件你可以在 mysql的安装目录 C:Program FilesMySQLMySQL Server 5.6lib 下找到。
2.2、工程中的其他方案,都是 Connector/C++ 的使用例子。作为参考非常有用,建议大家看一下。
三、库的使用
库使用需要几个文件:
1、include 文件夹 c/c++ /常规/附加包含目录
Connector/c++ 的安装版里面的Include 文件夹。或者把 /driver以及/driver/nativeapi 里面的头文件拷贝到一个文件夹里面(注意nativeapi要改名为 cppconn)。
2、Connector/c++ 库文件 和 MySql库文件:
2.1、mysqlcppconn.dll /debug,exe生成目录
2.2、mysqlcppconn.lib 链接器/输入/附加依赖项
2.3、libmysql.dll /debug
3、boost库所在目录 c/c++/常规/附加包含目录
四、使用例子
先建数据库:
create database test;
建表:
use test; create table test(id int ,name varchar(32));
插入数据:
insert into test.test value(1001,"sanyue"); insert into test.test value(1002,"sixbeauty"); insert into test.test value(1003,"chouwa");
创建存储过程:
use test; delimiter $ create procedure testproc1 (nId int) --注意这里和select那句的 ";" begin select id,name from test where id = nId; end $ delimiter ;
例子:
#include "mysql_connection.h" #include "mysql_driver.h" #include "cppconn/prepared_statement.h" #include "stdlib.h" using namespace std; typedef boost::scoped_ptr<sql::mysql::MySQL_Driver> MySQL_Driver; typedef boost::scoped_ptr<sql::Connection> Connection; typedef boost::scoped_ptr<sql::PreparedStatement> PreparedStatement; typedef boost::scoped_ptr<sql::Statement> Statement; typedef boost::scoped_ptr<sql::ResultSet> ResultSet; #define CRTDBG_MAP_ALLOC int main() { sql::mysql::MySQL_Driver *driver; Connection con(NULL); PreparedStatement prepareState(NULL); ResultSet result(NULL); //初始化驱动 try { driver=(sql::mysql::get_mysql_driver_instance()); //建立连接 con.reset(driver->connect("tcp://127.0.0.1:3306","root","123456")); if(con->isValid() == false) { cout<<"连接失败"<<endl; return -1; } // 查询存储过程 prepareState.reset(con->prepareStatement("call test.testproc1(?)")); prepareState->setInt(1,1001); prepareState->executeUpdate(); result.reset(prepareState->getResultSet()); // 输出结果 while(result->next()) { int id = result->getInt("id"); string name = result->getString("name"); cout<<"testuser: "<< id <<" , "<<name<<endl; } result->close(); prepareState->close(); con->close(); /* result.reset(NULL); prepareState.reset(NULL); con.reset(NULL); */ _CrtDumpMemoryLeaks(); //_CrtDumpMemoryLeaks(); } catch (sql::SQLException &e) { cout<<e.what()<<",state:"<<e.getSQLState()<<endl; cout<<"errorCode: " << e.getErrorCode()<<endl; } return 0; }