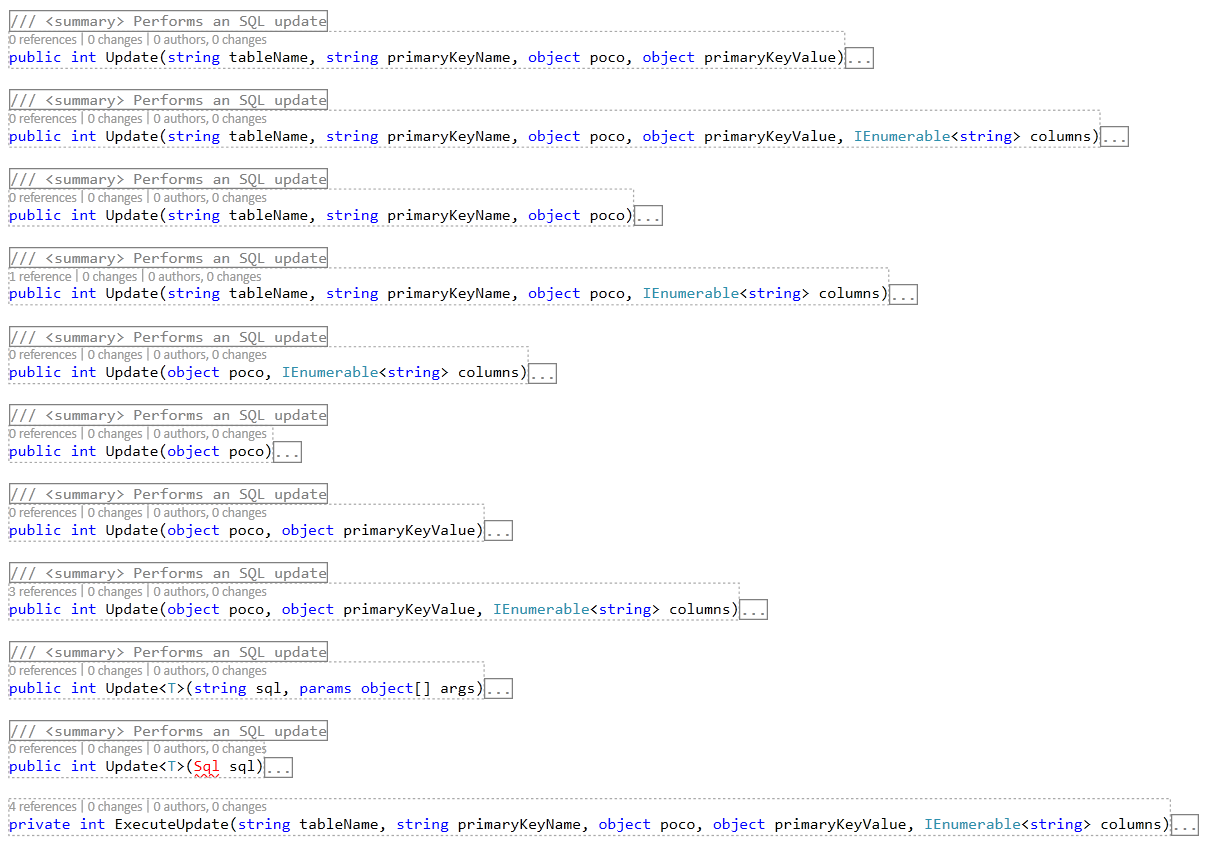

1 /// <summary> 2 /// Performs an SQL update 3 /// </summary> 4 /// <param name="tableName">The name of the table to update</param> 5 /// <param name="primaryKeyName">The name of the primary key column of the table</param> 6 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 7 /// <param name="primaryKeyValue">The primary key of the record to be updated</param> 8 /// <returns>The number of affected records</returns> 9 public int Update(string tableName, string primaryKeyName, object poco, object primaryKeyValue) 10 { 11 if (string.IsNullOrEmpty(tableName)) 12 throw new ArgumentNullException("tableName"); 13 14 if (string.IsNullOrEmpty(primaryKeyName)) 15 throw new ArgumentNullException("primaryKeyName"); 16 17 if (poco == null) 18 throw new ArgumentNullException("poco"); 19 20 return ExecuteUpdate(tableName, primaryKeyName, poco, primaryKeyValue, null); 21 } 22 23 /// <summary> 24 /// Performs an SQL update 25 /// </summary> 26 /// <param name="tableName">The name of the table to update</param> 27 /// <param name="primaryKeyName">The name of the primary key column of the table</param> 28 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 29 /// <param name="primaryKeyValue">The primary key of the record to be updated</param> 30 /// <param name="columns">The column names of the columns to be updated, or null for all</param> 31 /// <returns>The number of affected rows</returns> 32 public int Update(string tableName, string primaryKeyName, object poco, object primaryKeyValue, IEnumerable<string> columns) 33 { 34 if (string.IsNullOrEmpty(tableName)) 35 throw new ArgumentNullException("tableName"); 36 37 if (string.IsNullOrEmpty(primaryKeyName)) 38 throw new ArgumentNullException("primaryKeyName"); 39 40 if (poco == null) 41 throw new ArgumentNullException("poco"); 42 43 return ExecuteUpdate(tableName, primaryKeyName, poco, primaryKeyValue, columns); 44 } 45 46 /// <summary> 47 /// Performs an SQL update 48 /// </summary> 49 /// <param name="tableName">The name of the table to update</param> 50 /// <param name="primaryKeyName">The name of the primary key column of the table</param> 51 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 52 /// <returns>The number of affected rows</returns> 53 public int Update(string tableName, string primaryKeyName, object poco) 54 { 55 return Update(tableName, primaryKeyName, poco, null); 56 } 57 58 /// <summary> 59 /// Performs an SQL update 60 /// </summary> 61 /// <param name="tableName">The name of the table to update</param> 62 /// <param name="primaryKeyName">The name of the primary key column of the table</param> 63 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 64 /// <param name="columns">The column names of the columns to be updated, or null for all</param> 65 /// <returns>The number of affected rows</returns> 66 public int Update(string tableName, string primaryKeyName, object poco, IEnumerable<string> columns) 67 { 68 if (string.IsNullOrEmpty(tableName)) 69 throw new ArgumentNullException("tableName"); 70 71 if (string.IsNullOrEmpty(primaryKeyName)) 72 throw new ArgumentNullException("primaryKeyName"); 73 74 if (poco == null) 75 throw new ArgumentNullException("poco"); 76 77 return ExecuteUpdate(tableName, primaryKeyName, poco, null, columns); 78 } 79 80 /// <summary> 81 /// Performs an SQL update 82 /// </summary> 83 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 84 /// <param name="columns">The column names of the columns to be updated, or null for all</param> 85 /// <returns>The number of affected rows</returns> 86 public int Update(object poco, IEnumerable<string> columns) 87 { 88 return Update(poco, null, columns); 89 } 90 91 /// <summary> 92 /// Performs an SQL update 93 /// </summary> 94 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 95 /// <returns>The number of affected rows</returns> 96 public int Update(object poco) 97 { 98 return Update(poco, null, null); 99 } 100 101 /// <summary> 102 /// Performs an SQL update 103 /// </summary> 104 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 105 /// <param name="primaryKeyValue">The primary key of the record to be updated</param> 106 /// <returns>The number of affected rows</returns> 107 public int Update(object poco, object primaryKeyValue) 108 { 109 return Update(poco, primaryKeyValue, null); 110 } 111 112 /// <summary> 113 /// Performs an SQL update 114 /// </summary> 115 /// <param name="poco">The POCO object that specifies the column values to be updated</param> 116 /// <param name="primaryKeyValue">The primary key of the record to be updated</param> 117 /// <param name="columns">The column names of the columns to be updated, or null for all</param> 118 /// <returns>The number of affected rows</returns> 119 public int Update(object poco, object primaryKeyValue, IEnumerable<string> columns) 120 { 121 if (poco == null) 122 throw new ArgumentNullException("poco"); 123 124 var pd = PocoData.ForType(poco.GetType(), _defaultMapper); 125 return ExecuteUpdate(pd.TableInfo.TableName, pd.TableInfo.PrimaryKey, poco, primaryKeyValue, columns); 126 } 127 128 /// <summary> 129 /// Performs an SQL update 130 /// </summary> 131 /// <typeparam name="T">The POCO class who's attributes specify the name of the table to update</typeparam> 132 /// <param name="sql">The SQL update and condition clause (ie: everything after "UPDATE tablename"</param> 133 /// <param name="args">Arguments to any embedded parameters in the SQL</param> 134 /// <returns>The number of affected rows</returns> 135 public int Update<T>(string sql, params object[] args) 136 { 137 if (string.IsNullOrEmpty(sql)) 138 throw new ArgumentNullException("sql"); 139 140 var pd = PocoData.ForType(typeof(T), _defaultMapper); 141 return Execute(string.Format("UPDATE {0} {1}", _provider.EscapeTableName(pd.TableInfo.TableName), sql), args); 142 } 143 144 /// <summary> 145 /// Performs an SQL update 146 /// </summary> 147 /// <typeparam name="T">The POCO class who's attributes specify the name of the table to update</typeparam> 148 /// <param name="sql"> 149 /// An SQL builder object representing the SQL update and condition clause (ie: everything after "UPDATE 150 /// tablename" 151 /// </param> 152 /// <returns>The number of affected rows</returns> 153 public int Update<T>(Sql sql) 154 { 155 if (sql == null) 156 throw new ArgumentNullException("sql"); 157 158 var pd = PocoData.ForType(typeof(T), _defaultMapper); 159 return Execute(new Sql(string.Format("UPDATE {0}", _provider.EscapeTableName(pd.TableInfo.TableName))).Append(sql)); 160 } 161 162 private int ExecuteUpdate(string tableName, string primaryKeyName, object poco, object primaryKeyValue, IEnumerable<string> columns) 163 { 164 try 165 { 166 OpenSharedConnection(); 167 try 168 { 169 using (var cmd = CreateCommand(_sharedConnection, "")) 170 { 171 var sb = new StringBuilder(); 172 var index = 0; 173 var pd = PocoData.ForObject(poco, primaryKeyName, _defaultMapper); 174 if (columns == null) 175 { 176 foreach (var i in pd.Columns) 177 { 178 // Don't update the primary key, but grab the value if we don't have it 179 if (string.Compare(i.Key, primaryKeyName, true) == 0) 180 { 181 if (primaryKeyValue == null) 182 primaryKeyValue = i.Value.GetValue(poco); 183 continue; 184 } 185 186 // Dont update result only columns 187 if (i.Value.ResultColumn) 188 continue; 189 190 // Build the sql 191 if (index > 0) 192 sb.Append(", "); 193 sb.AppendFormat("{0} = {1}{2}", _provider.EscapeSqlIdentifier(i.Key), _paramPrefix, index++); 194 195 // Store the parameter in the command 196 AddParam(cmd, i.Value.GetValue(poco), i.Value.PropertyInfo); 197 } 198 } 199 else 200 { 201 foreach (var colname in columns) 202 { 203 var pc = pd.Columns[colname]; 204 205 // Build the sql 206 if (index > 0) 207 sb.Append(", "); 208 sb.AppendFormat("{0} = {1}{2}", _provider.EscapeSqlIdentifier(colname), _paramPrefix, index++); 209 210 // Store the parameter in the command 211 AddParam(cmd, pc.GetValue(poco), pc.PropertyInfo); 212 } 213 214 // Grab primary key value 215 if (primaryKeyValue == null) 216 { 217 var pc = pd.Columns[primaryKeyName]; 218 primaryKeyValue = pc.GetValue(poco); 219 } 220 } 221 222 // Find the property info for the primary key 223 PropertyInfo pkpi = null; 224 if (primaryKeyName != null) 225 { 226 PocoColumn col; 227 pkpi = pd.Columns.TryGetValue(primaryKeyName, out col) 228 ? col.PropertyInfo 229 : new { Id = primaryKeyValue }.GetType().GetProperties()[0]; 230 } 231 232 cmd.CommandText = string.Format("UPDATE {0} SET {1} WHERE {2} = {3}{4}", 233 _provider.EscapeTableName(tableName), sb.ToString(), _provider.EscapeSqlIdentifier(primaryKeyName), _paramPrefix, index++); 234 AddParam(cmd, primaryKeyValue, pkpi); 235 236 DoPreExecute(cmd); 237 238 // Do it 239 var retv = cmd.ExecuteNonQuery(); 240 OnExecutedCommand(cmd); 241 return retv; 242 } 243 } 244 finally 245 { 246 CloseSharedConnection(); 247 } 248 } 249 catch (Exception x) 250 { 251 if (OnException(x)) 252 throw; 253 return -1; 254 } 255 }